How to Change Button Text on Click in ReactJS ?
Last Updated :
17 Dec, 2023
In this article, we will learn about diverse methods for altering button text dynamically upon clicking on the button. React, a widely embraced JavaScript library for crafting user interfaces provides several approaches to seamlessly achieve this functionality. The key lies in manipulating the component’s state and adjusting its rendering logic to reflect the updated button text based on user interactions.
We will discuss the following two approaches to change button text on click in React:
Prerequisites
Steps to Create React Application:
Step 1: Create a react application by using this command
npx create-react-app <<Project-Name>>
Step 2: After creating your project folder, use the following command to navigate to it
cd <<Project-Name>>
Project Structure:
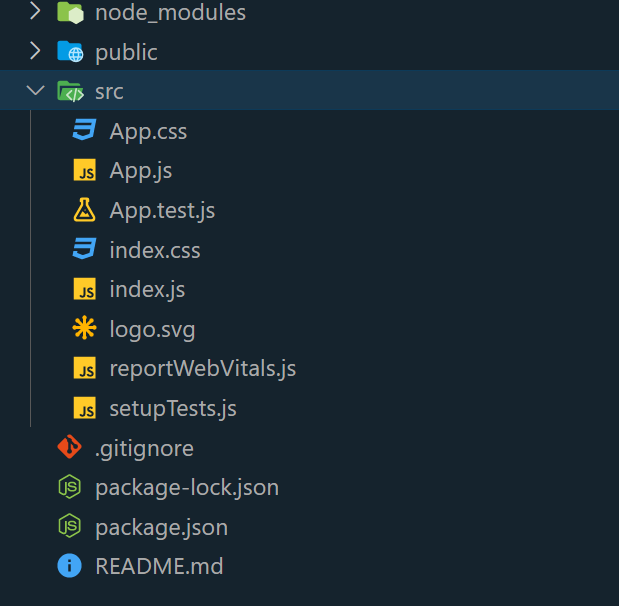
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Approach 1: Using setTimeout() Method
In this method, the button text swiftly changes to “Loading…” on click using `setTimeout()` and `setButtonText()`. After a deliberate 2-second delay, the original text is restored, creating a brief loading state for enhanced user experience.
Example: Below is the code example of the above explained approach
Javascript
import React, { useState } from 'react' ;
function App() {
const [buttonText, setButtonText] = useState( 'Submit' );
const handleClick = () => {
setButtonText( 'Loading...' );
setTimeout(() => {
setButtonText( 'Submit' );
}, 2000);
};
return (
<div style={styles.container}>
<h1 style={styles.heading}>
Geeksforgeeks
</h1>
<button onClick={handleClick}
style={styles.btn}>
{buttonText}
</button>
</div>
);
}
export default App;
const styles = {
container: {
textAlign: 'center' ,
margin: 'auto' ,
padding: '20px' ,
width: 400,
},
heading: {
fontSize: '34px' ,
marginBottom: '10px' ,
color: "green" ,
borderBottom: "3px solid crimson" ,
paddingBottom: 20,
borderRadius: "8px" ,
},
btn: {
backgroundColor: 'green' ,
fontSize: 20,
color: 'white' ,
cursor: 'pointer' ,
margin: 10,
padding: 15,
borderRadius: "8px" ,
border: "none" ,
boxShadow: "0px 0px 10px 0px grey" ,
},
};
|
Output:
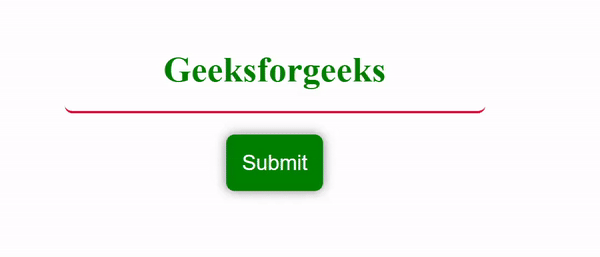
Approach 2: Using Ternary Operator
Streamline a button’s text change using a ternary operator by modifying the “buttonText” state in the handleClick function. The operator evaluates the current value and switches between “Submit” and “Loading…”, prompting React to re-render the updated text on each click.
Example: Below is the code example of the above explained approach
Javascript
import React, { useState } from 'react' ;
function App() {
const [buttonText, setButtonText] = useState( 'Submit' );
const handleClick = () => {
setButtonText(buttonText === 'Submit' ? 'Loading...' : 'Submit' );
};
return (
<div style={styles.container}>
<h1 style={styles.heading}>Geeksforgeeks</h1>
<button onClick={handleClick}
style={styles.btn}>
{buttonText}
</button>
</div>
);
}
export default App;
const styles = {
container: {
textAlign: 'center' ,
margin: 'auto' ,
padding: '20px' ,
width: 400,
},
heading: {
fontSize: '34px' ,
marginBottom: '10px' ,
color: "green" ,
borderBottom: "3px solid crimson" ,
paddingBottom: 20,
borderRadius: "8px" ,
},
btn: {
backgroundColor: 'green' ,
fontSize: 20,
color: 'white' ,
cursor: 'pointer' ,
margin: 10,
padding: 15,
borderRadius: "8px" ,
border: "none" ,
boxShadow: "0px 0px 10px 0px grey" ,
},
};
|
Output:
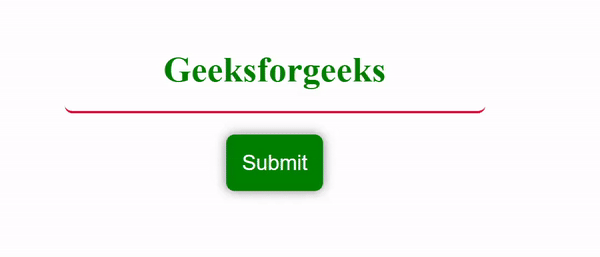
Output
Share your thoughts in the comments
Please Login to comment...