How to iterate over JSON object fetched from API in Angular ?
Last Updated :
23 Nov, 2023
JSON stands for JavaScript Object Notation. It is a format for structuring data. This format is used by different web applications to communicate with each other. It is the replacement of the XML data exchange format. In this article, we will learn How to Iterate over JSON objects fetched from API in Angular.
Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
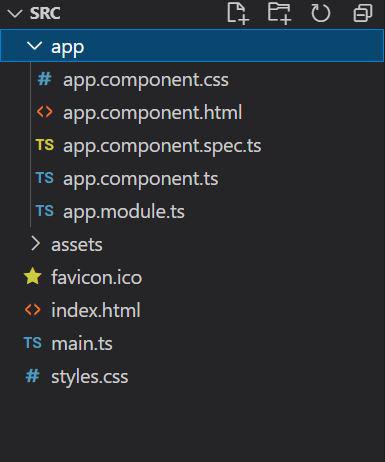
Example 1: In this example, we will call a public API, & once the promise is resolved, & then we will store the value of this promise in a variable called promiseValue. Here we will iterate over a single object and display it in HTML
HTML
< div >
< h2 style = "color:green" >GeeksforGeeks</ h2 >
< h2 >How to Iterate over JSON object fetched
from API in Angular ?
</ h2 >
< p >< strong >ID:</ strong > {{ promiseValue.id }}</ p >
< p >< strong >Title: </ strong >{{ promiseValue.title }}</ p >
< p >< strong >Body: </ strong >{{ promiseValue.body }}</ p >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { HttpClient }
from '@angular/common/http' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
promiseValue: any;
constructor(private http: HttpClient) { }
ngOnInit() {
this .http.get(
(result) => {
this .promiseValue = result;
}
)
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
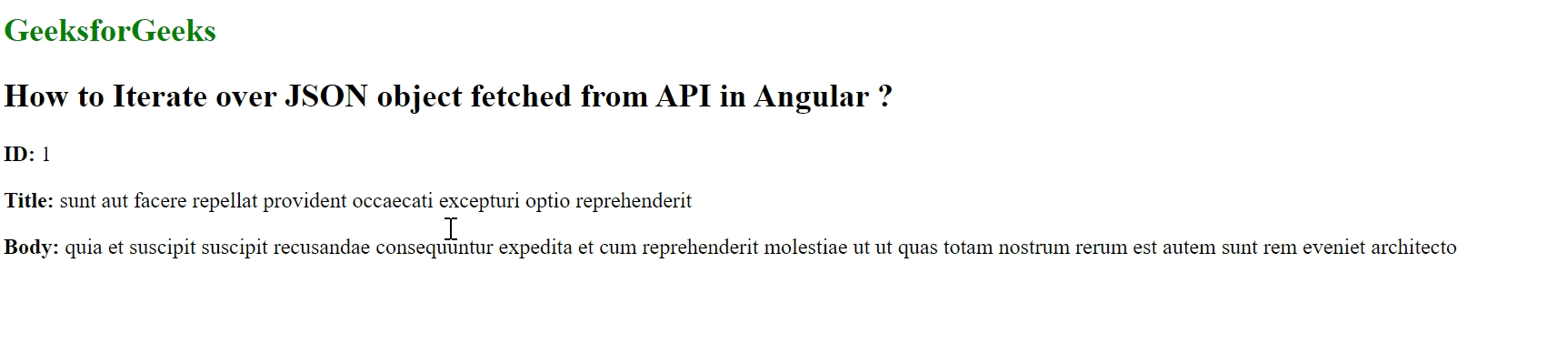
Example 2: In this example, we will fetch many objects from API, & iterate over them, and display them in HTML.
HTML
< div >
< h2 style = "color:green" >GeeksforGeeks</ h2 >
< h2 >
How to Iterate over JSON object
fetched from API in Angular ?
</ h2 >
< ol >
< li * ngFor = "let result of promiseValue" >
< p >Title : {{result.title}}</ p >
< p >Album name: {{result.title}}</ p >
</ li >
</ ol >
</ div >
|
Javascript
import { Component, OnInit }
from '@angular/core' ;
import { HttpClient }
from '@angular/common/http' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
promiseValue: any;
constructor(private http: HttpClient) { }
ngOnInit() {
this .http.get(
(result) => {
this .promiseValue = result;
}
)
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...