How to transforms JSON object to pretty-printed JSON using Angular Pipe ?
Last Updated :
29 Nov, 2023
JSON stands for JavaScript Object Notation. It is a format for structuring data. This format is used by different web applications to communicate with each other. In this article, we will see How to use pipe to transform a JSON object to pretty-printed JSON using Angular Pipe. It meaning that it will take a JSON object string and return it pretty-printed/formatted to display to the user.
For example, it would take this:
{ "id": 1, "number": "K3483483344"}
Final Output:
{
"id": 1,
"number": "K3483483344"
}
And return something that looks like this when displayed in HTML.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project Structure
It will look like the following:
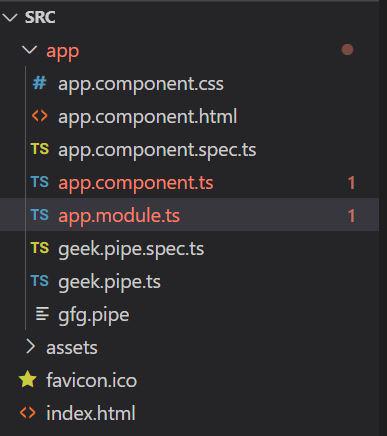
Example 1: In this article, we will transform JSON object to pretty-printed using stringify.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
Angular 2 pipe that transforms JSON
object to pretty-printed JSON
</ h2 >
< pre [innerHTML]="gfg | pretty"></ pre >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent {
gfg = {
course: 'GPL' ,
description: 'Geeks Premier League 2023' ,
vote: "Vote for me"
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
import { FormsModule }
from '@angular/forms' ;
import { GeekPipe }
from './geek.pipe' ;
@NgModule({
declarations: [
AppComponent,
GeekPipe
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Javascript
import { Pipe, PipeTransform } from '@angular/core' ;
@Pipe({
name: 'pretty'
})
export class GeekPipe implements PipeTransform {
transform(val: any) {
return JSON.stringify(val, undefined, 4)
.replace(/ /g, ' ' )
.replace(/\n/g, '<br/>' );
}
}
|
Output:
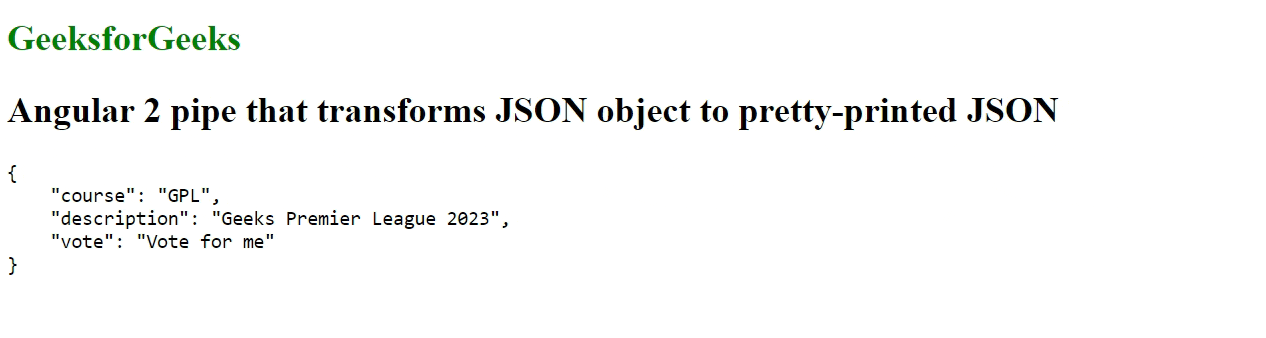
Example 2: This is another example, we will pass JSON object which has nested JSON object in it.
HTML
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
Angular 2 pipe that transforms JSON
object to pretty-printed JSON
</ h2 >
< pre [innerHTML]="gfg | pretty"></ pre >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html'
})
export class AppComponent {
gfg = {
course: 'FrontEnd' ,
description: 'Geeks Front End Courses' ,
parts: {
value1: 'HTML' ,
value2: 'CSS' ,
value3: 'Javascript' ,
value4: 'Angular'
}
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
import { FormsModule }
from '@angular/forms' ;
import { GeekPipe }
from './geek.pipe' ;
@NgModule({
declarations: [
AppComponent,
GeekPipe
],
imports: [
BrowserModule,
HttpClientModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Javascript
import { Pipe, PipeTransform } from '@angular/core' ;
@Pipe({
name: 'pretty'
})
export class GeekPipe implements PipeTransform {
transform(val: any) {
return JSON.stringify(val, undefined, 4)
.replace(/ /g, ' ' )
.replace(/\n/g, '<br/>' );
}
}
|
Output:
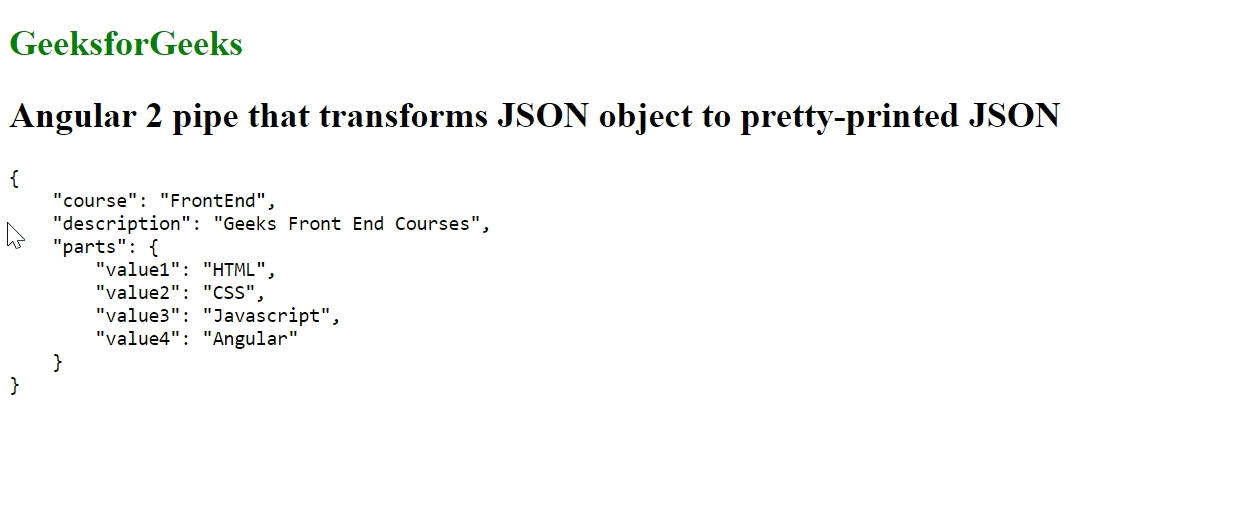
Share your thoughts in the comments
Please Login to comment...