How to display static JSON data in table in Angular ?
Last Updated :
06 Dec, 2023
In this article, we will see How to display static JSON data in the table in Angular. We will be displaying static data objects in Angular Table. We need to iterate over the object keys and values using key values and then handle them in the table. We will be using the bootstrap table and Angular PrimeNG Table template
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2:Â After creating your project folder i.e. appname, move to it using the following command.
cd appname
Project StructureÂ
It will look like the following:
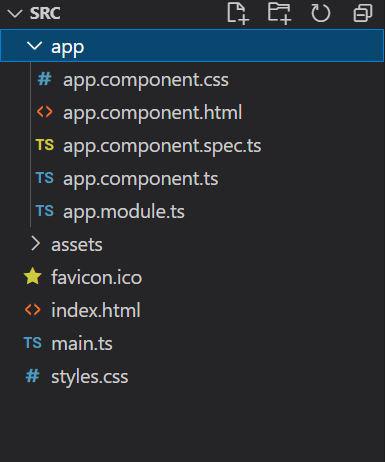
Example 1: In this example, we will use a normal bootstrap table to display the static JSON data. We will be iterating over the JSON object using keyvalue.
HTML
< head >
< link href =
rel = "stylesheet" >
</ head >
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h2 >
How to display static JSON data in table in Angular
</ h2 >
< table class = "table table-striped" style = "width:50%" >
< thead >
< tr >
< th >
Sr No.
</ th >
< th >
Course
</ th >
< th >
Cost
</ th >
</ tr >
</ thead >
< tbody >
< tr * ngFor = "let item of gfg|keyvalue" >
< td >{{item.key}}</ td >
{{set(item.value)}}
< td * ngFor = "let element of gfg2|keyvalue" >
{{element.value}}
</ td >
</ tr >
</ tbody >
</ table >
|
Javascript
import { Component, OnInit } from '@angular/core' ;
import { KeyValue } from '@angular/common' ;
import { Pipe, PipeTransform } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: "./app.component.html" ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
gfg: any =
{
"3" :
{
"course" : "Java" ,
"cost" : "1000"
},
"4" :
{
"course" : "React" ,
"cost" : "1500"
},
"2" :
{
"course" : "Angular" ,
"cost" : "1700"
}
,
"1" :
{
"course" : "CSS" ,
"cost" : "800"
}
}
gfg2: any
set(obj: any) {
this .gfg2 = obj
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { HttpClientModule }
from '@angular/common/http' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output:
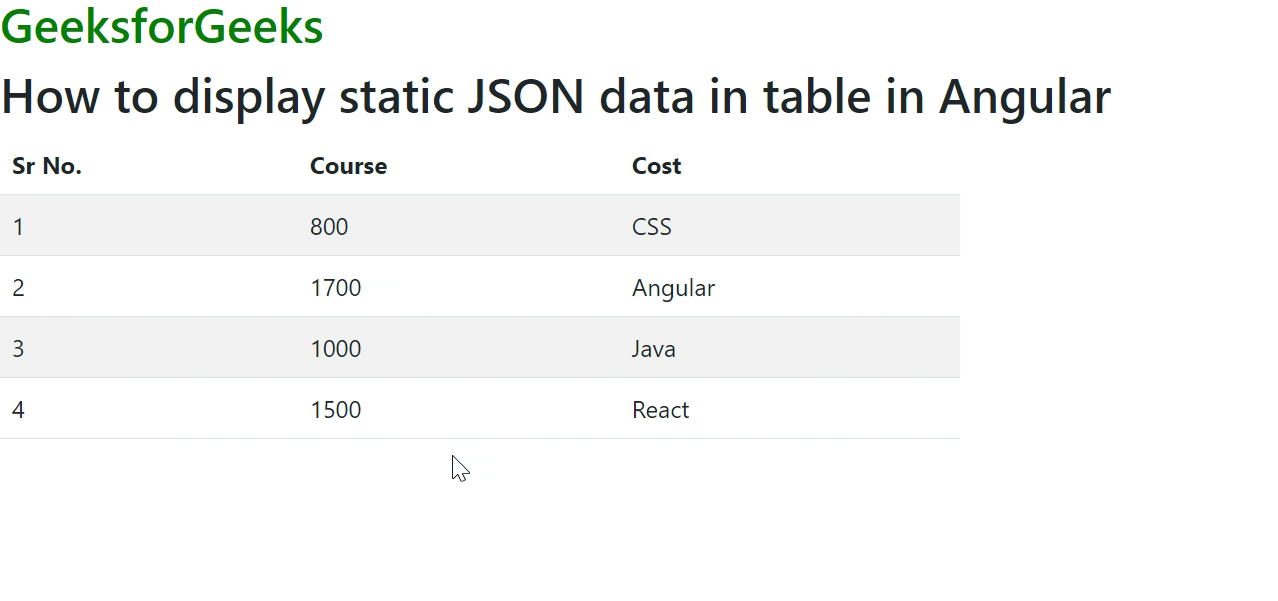
Example 2: In this example, we will be rendering static JSON data in Angular.
HTML
< div style = "width:50%" >
< h2 style = "color: green" >
GeeksforGeeks
</ h2 >
< h2 >
How to display static JSON
data in table in Angular
</ h2 >
< p-table #myTab [value]="tableData">
< ng-template pTemplate = "header" >
< tr >
< th >First Name</ th >
< th >Last Name</ th >
< th >Age</ th >
</ tr >
</ ng-template >
< ng-template pTemplate = "body" let-people>
< tr >
< td >
{{ people.firstname }}
</ td >
< td >
{{ people.lastname }}
</ td >
< td >
{{ people.age }}
</ td >
</ tr >
</ ng-template >
</ p-table >
</ div >
|
Javascript
import { Component } from '@angular/core' ;
interface People {
firstname?: string;
lastname?: string;
age?: string;
}
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
})
export class AppComponent {
tableData: People[] = [];
cols: any[] = [];
constructor() { }
ngOnInit() {
this .cols = [
{
field: 'firstname' ,
header: 'First Name'
},
{
field: 'lastname' ,
header: 'Last Name'
},
{
field: 'age' ,
header: 'Age'
},
];
this .tableData = [
{
firstname: 'David' ,
lastname: 'ace' ,
age: '40' ,
},
{
firstname: 'AJne' ,
lastname: 'west' ,
age: '40' ,
},
{
firstname: 'Mak' ,
lastname: 'Lame' ,
age: '40' ,
},
{
firstname: 'Peter' ,
lastname: 'raw' ,
age: '40' ,
}
];
}
}
|
Javascript
import { NgModule }
from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { FormsModule }
from '@angular/forms' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent }
from './app.component' ;
import { TableModule }
from 'primeng/table' ;
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule,
TableModule,
FormsModule,
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
Output:
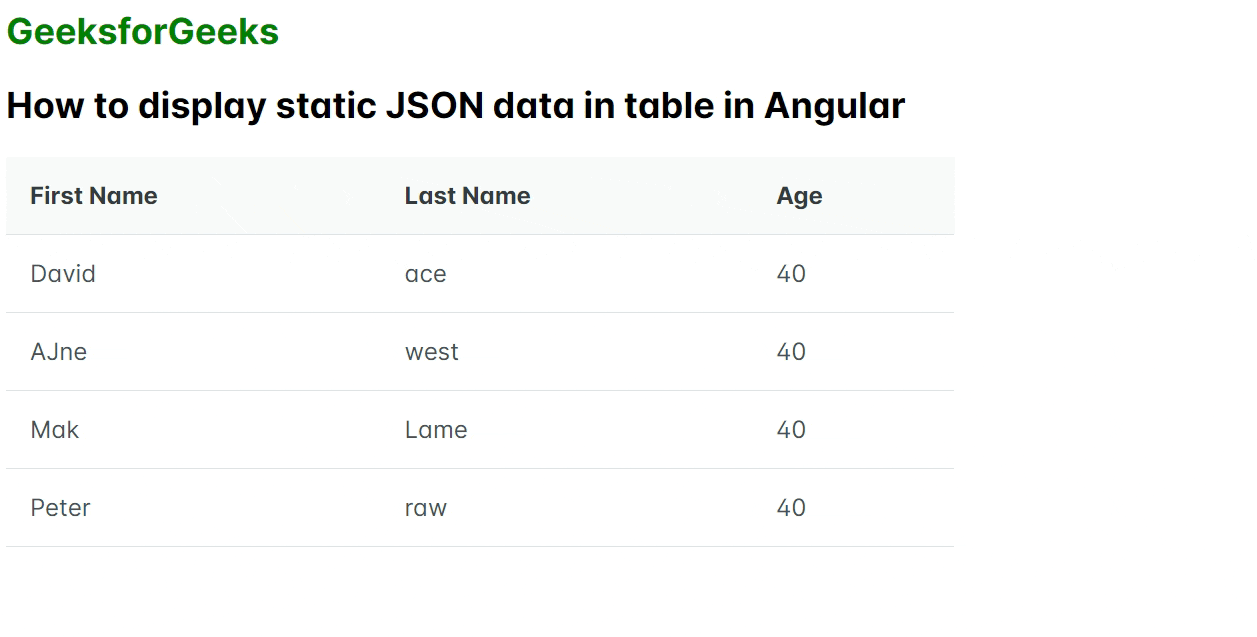
Share your thoughts in the comments
Please Login to comment...