How to implement “Remember Me” Feature in React JS ?
Last Updated :
19 Mar, 2024
In React JS, the implementation of the “Remember Me” functionality consists of using local storage to store user credentials between sessions. By using this functionality, the users can automate the login process. In this article, we will explore the practical implementation of Remember Me in ReactJS.
Approach to implement “Remember me” Feature:
- The Remember Me Functionality is implemented using the localStorage.
- There is a Login form and the Remember Me Checkbox to store the states from the stored values in localStorage during the component mounting.
- When the user logs in with the credentials that are checked, and if the Remember Me is enabled, the username and password are automatically stored in the localStorage.
- When the user revisits the application, the user is automatically logged into the application.
Steps to Create React Application And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: After setting up the react environment on your system, we can start by creating an App.js file and create a directory by the name of the components in which we will write our desired function.
Project Structure:
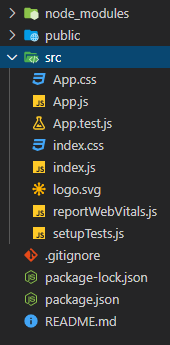
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: The below example demonstrates the Implement remember me in React JS.
CSS
.app-container {
max-width: 400px;
margin: 50px auto;
padding: 20px;
border: 1px solid #ddd;
border-radius: 5px;
background-color: #f8f8f8;
text-align: center;
}
.geeks-header {
color: #4caf50;
font-size: 2em;
margin-bottom: 20px;
}
.login-header {
color: #333;
}
.input-field {
width: 100%;
padding: 8px;
margin: 8px 0;
box-sizing: border-box;
}
.remember-me-text {
color: #555;
margin-right: 8px;
display: inline-block;
}
.login-button,
.logout-button {
background-color: #4caf50;
color: white;
padding: 10px;
margin: 10px 0;
border: none;
border-radius: 5px;
cursor: pointer;
}
.login-button:hover,
.logout-button:hover {
background-color: #45a049;
}
.welcome-text {
color: #4caf50;
margin-top: 10px;
margin-bottom: 20px;
}
Javascript
import React, { useState, useEffect } from "react";
import "./App.css";
const App = () => {
const [uName, setuName] = useState("admin");
const [uPass, setuPass] = useState("admin");
const [rMe, setrMe] = useState(false);
const [isLog, setisLog] = useState(false);
useEffect(() => {
const userStore = localStorage.getItem("username");
const passStore = localStorage.getItem("password");
const rmeStore = localStorage.getItem("rememberMe");
if (
rmeStore === "true" &&
userStore === "admin" &&
passStore === "admin"
) {
setisLog(true);
}
if (userStore) {
setuName(userStore);
}
if (passStore) {
setuPass(passStore);
}
}, []);
const loginFn = () => {
if (uName === "admin" && uPass === "admin") {
setisLog(true);
if (rMe) {
localStorage.setItem("username", uName);
localStorage.setItem("password", uPass);
localStorage.setItem("rememberMe", true);
} else {
localStorage.removeItem("username");
localStorage.removeItem("password");
localStorage.removeItem("rememberMe");
}
}
};
const logoutFn = () => {
setisLog(false);
localStorage.removeItem("username");
localStorage.removeItem("password");
localStorage.removeItem("rememberMe");
};
return (
<div className="app-container">
<h1 className="geeks-header">GeeksforGeeks</h1>
{isLog ? (
<div>
<h2 className="welcome-text">
Welcome to GeeksforGeeks
</h2>
<button onClick={logoutFn}
className="logout-button">
Logout
</button>
</div>
) : (
<div>
<h2 className="login-header">Login</h2>
<label>
Username:
<input
type="text"
value={uName}
onChange={(e) => setuName(e.target.value)}
className="input-field"
/>
</label>
<br />
<label>
Password:
<input
type="password"
value={uPass}
onChange={(e) => setuPass(e.target.value)}
className="input-field"
/>
</label>
<br />
<label>
<h3 className="remember-me-text">
Remember Me:
</h3>
<input
type="checkbox"
checked={rMe}
onChange={() => setrMe(!rMe)}
/>
</label>
<br />
<button onClick={loginFn} \
className="login-button">
Login
</button>
</div>
)}
</div>
);
};
export default App;
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
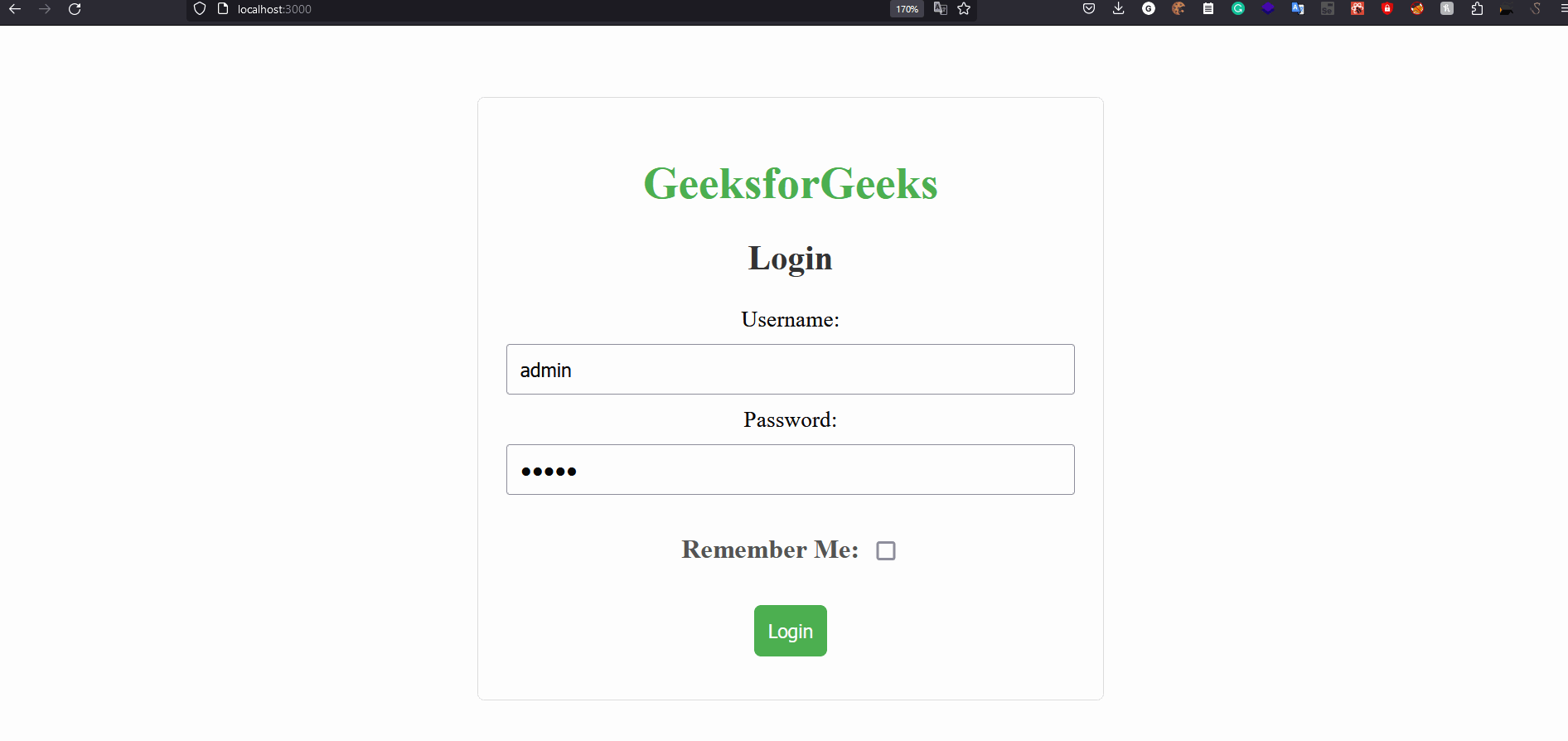
Share your thoughts in the comments
Please Login to comment...