How to add Color Picker in React Native ?
Last Updated :
06 Sep, 2023
React Native is a popular cross-platform framework for mobile app development using JavaScript. It enables code reuse for iOS and Android platforms and frequently involves incorporating a color picker component. In this article, we’ll see how to add a color picker in React native application by using the react-native-color-picker library
A color picker component is commonly used in mobile app development to allow users to choose and interact with colors visually. The `react-native-color-picker` library offers a customizable solution for implementing a color picker component in React Native apps.
Prerequisites
Creating React Native App
Step 1: Install Expo CLI globally by running this command in your terminal: It only works on version v46.0.0
npm install -g expo-cli@4.6.0
Step 2: Create React Native Application With Expo CLI
expo init color-picker-native
Step 3: ​Navigate to the project directory by using this command:
cd color-picker-native
Project Structure
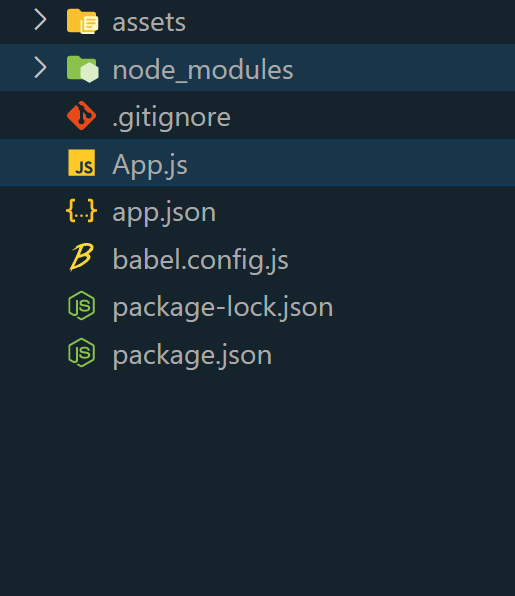
Step 4: Install Required Dependencies
npm install react-native-color-picker
Approach
To add a color picker in a React Native app, follow these steps: Set up a React Native project, install the `react-native-color-picker` library, import components and define the `ColorPicker` component, create a reference with `useRef`, implement color selection and clipboard functions, render the color picker and run the app.
Javascript
import React, { useRef } from 'react' ;
import { StyleSheet, Text, View, Clipboard,
TextInput } from 'react-native' ;
import { ColorPicker } from 'react-native-color-picker' ;
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#f5f5f5' ,
alignItems: 'center' ,
justifyContent: 'center' ,
},
title: {
fontSize: 24,
fontWeight: 'bold' ,
marginBottom: 20,
},
colorPicker: {
width: 300,
height: 500,
borderRadius: 10,
marginBottom: 20,
},
input: {
color: 'black' ,
fontWeight: 'bold' ,
fontSize: 20,
},
});
export default function App() {
const colorPickerRef = useRef( null );
const handleColorSelected = (color) => {
Clipboard.setString(color);
alert(`Color selected: ${color} (Copied to clipboard)`);
};
return (
<View style={styles.container}>
<Text style={styles.title}>Color Picker</Text>
<ColorPicker
ref={colorPickerRef}
onColorSelected={handleColorSelected}
style={styles.colorPicker}
/>
<TextInput
style={styles.input}
placeholder= "Paste The Color Code"
/>
</View>
);
}
|
Step 6: To run the react native application, open the Terminal and enter the command listed below.
npx expo start
To run on Android:
npx react-native run-android
To run on Ios:
npx react-native run-ios
Output:
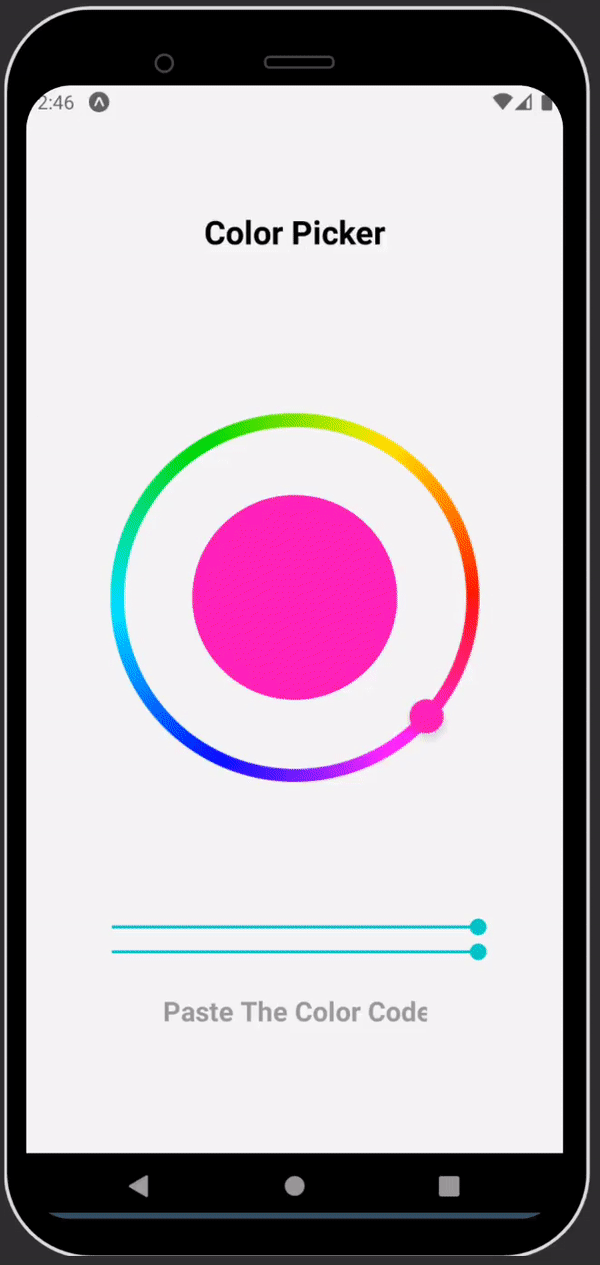
Share your thoughts in the comments
Please Login to comment...