GFact | Why s.lower_bound(x) is faster than lower_bound(s.begin(), s.end(), x) in C++ STL?
Last Updated :
22 Sep, 2023
Have you ever got TLE on solving a question using function lower_bound (s.begin(), s.end(), x) and when you use s.lower_bound(x) it runs just fine (here s is a Data structure and x is the value we are searching for) !! If “Yes” then why this happens?
Well, we are going to find out this in the following article, but before that let’s know about the function, lower_bound () in C++, first.
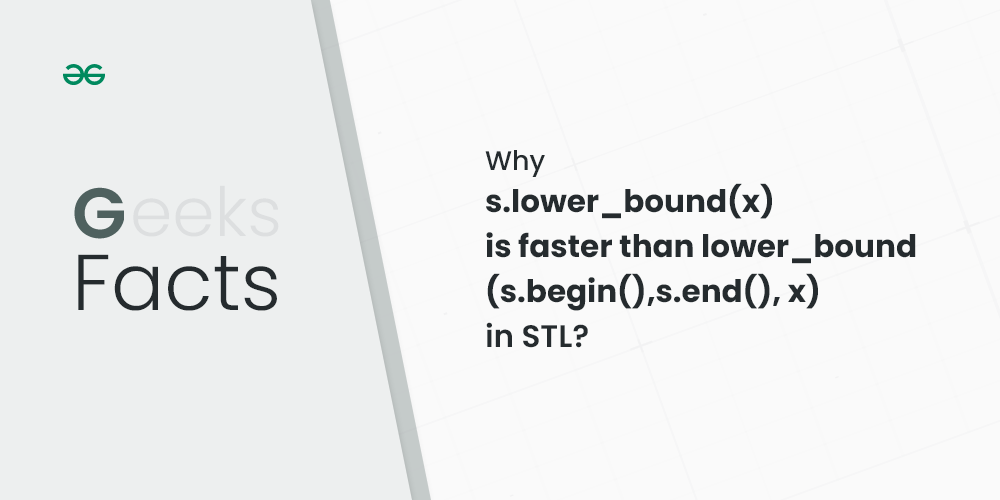
Why s.lower_bound(x) is faster than lower_bound(s.begin(), s.end(), x) in C++ STL?
What is lower_bound ()?
In C++, lower_bound is a function provided by the Standard Template Library (STL) that is used for binary searching in a sorted sequence. It is defined in the <algorithm> header and is typically used with containers like vectors, arrays, or other data structures that support random access.
lower_bound function is used to find the iterator pointing to the first element in a sorted sequence that is not less than a specified value. It is commonly used with sorted containers to quickly locate an element or determine the position where an element should be inserted while maintaining the sorted order.
Syntax of lower_bound:
template <class ForwardIterator, class T>
ForwardIterator lower_bound(ForwardIterator first, ForwardIterator last, const T& value);
- ‘first’ and ‘last’ are iterators defining the range in which you want to search for the value. and ‘value‘ is the value that you want to find or insert.
- The lower_bound function returns an iterator pointing to the first element in the range [first, last) that is not less than value. If no such element is found, it returns last.
Difference between s.lower_bound(x) and lower_bound(s.begin(), s.end(), x):
The difference between s.lower_bound(x) and lower_bound(s.begin(), s.end(), x) lies in their usage and behaviour, depending on the context of the container s and how they are called.
s.lower_bound(x):
- This is a member function of certain standard C++ containers like set, map, multiset, and multimap.
- It is specifically designed for these containers and utilizes their internal data structures (usually binary search trees) to efficiently find the lower bound of x.
- This method is more convenient and often more efficient when working with these containers, as it leverages their inherent organization and optimizations.
- It returns an iterator to the first element in the container that is not less than x. If no such element is found, it returns an iterator to the end of the container.
Code for s.lower_bound(x):
C++
std::set< int > s = {1, 2, 3, 4, 5};
auto it = s.lower_bound(3);
|
lower_bound(s.begin(), s.end(), x):
- This is a generic algorithm from the C++ Standard Template Library (STL) and can be used with any container that provides forward iterators.
- It is not container-specific and does not take advantage of any internal optimizations or structures of the container s.
- It requires providing the range of elements [s.begin(), s.end()) explicitly, and it performs a binary search within that range.
- It returns an iterator to the first element in the specified range that is not less than x. If no such element is found, it returns an iterator to the end of the specified range.
Code for lower_bound(s.begin(), s.end(), x):
C++
std::vector< int > s = {1, 2, 3, 4, 5};
auto it = lower_bound(s.begin(), s.end(), 3);
|
Why s.lower_bound(x) is faster than lower_bound(s.begin(), s.end(), x)?
- So now we know s.lower_bound(x) is a member function that’s container-specific and generally more efficient for containers that support it ( like set, map, multiset, and multimap).
- While lower_bound(s.begin(), s.end(), x) is a generic algorithm that can be used with a wider range of containers but doesn’t take advantage of container-specific optimizations. The choice between them depends on the container type and your specific requirements.
In summary, using s.lower_bound(x) is often faster than lower_bound(s.begin(), s.end(), x) because it leverages container-specific optimizations, reduces function call overhead, and benefits from better type inference. However, it’s important to note that the performance difference may not be significant in all cases, and it’s essential to choose the appropriate method based on your specific use case and requirements.
Share your thoughts in the comments
Please Login to comment...