How does Selenium Webdriver Handle Proxy Authentication?
Last Updated :
03 Jan, 2024
Selenium is an open-source tool that provides us with the libraries that are used for browser automation the selenium browser allows us to test different functionalities in our website, thus the Selenium web driver comes very handy in day life if we are regularly testing web applications or doing automation tasks.
What is a Proxy?
In general, a proxy is a server application or interface acting as a middleman between a client making a request and the server providing the requested resource. In the context of Selenium, a proxy serves as an intermediary between the user (client) and the server from which data is requested.
- Proxies enhance privacy and are powerful tools for managing communication between the client and server.
- The two commonly used types are HTTP proxy and SOCKS proxy. These proxies play a crucial role in ensuring efficient and secure data exchange.
What is Proxy Authentication?
Proxy authentication involves the process of gaining access to a specific resource behind a proxy server. The HTTP proxy authenticate response header defines it as a method used to access a resource available behind a proxy. In proxy authentication, requests are made to the proxy server, and upon successful authentication, the proxy server allows the transmission of the request to access the desired resource.
- When dealing with data that requires user credentials or logging in, it falls under the category of proxy authentication.
- Once proxy authentication is completed, the proxy server forwards the request, granting access to the data or account information.
- The Selenium WebDriver can be used to handle proxy authentication seamlessly, enabling the automation of tasks involving authenticated access to resources.
SOCKS vs HTTP Proxy
SOCKS proxy uses tunneling encryption between the client and the proxy server.
|
HTTP proxy gives an extra layer of protection between the client and proxy server.
|
SOCKS proxies offer more speed which is why they are better for transferring data.
|
Privatized HTTP proxies offer lower speeds but are decent enough to handle more requests.
|
SOCK proxies do not use the HTTP protocol directly, it’s used for more common content, i.e. content streaming.
|
HTTP is a proxy that handles HTTP(S) traffic which is more often used for retrieving information in the web browsers more securely.
|
SOCK proxies are known as low-level proxies.
|
HTTP proxies are known as high-level proxies.
|
SOCK proxy can handle any program/protocol with a high amount of traffic.
|
HTTP proxy can not handle large data programs such as streaming or P2P file-sharing programs as it is built for information retrieval.
|
SOCK proxy connects with a limited number of tools.
|
HTTP proxy can easily be connected to practically every tool.
|
Steps to handle Proxy Authentication using Selenium Webdriver
Prerequisites:
Example 1: Manual Proxy Authentication Handling
We have cretated a PHP file (selenium_auth.php) that accepts a proxy authentication request of user name and password:
PHP
<?php
$php_variable = "Hello from GeeksforGeeks!" ;
echo '<html>
<head>
<title>Selenium Authentication</title>
</head>
<body>
<head>
</head>
<div style= "margin-left:20px;" >
<script>
var username = prompt( "Enter username-" , "" );
var password = prompt( "Enter Password" , "" );
if (username == "gfg" && password == "gfg" ) {
document.write( "Proxy Authentication Passed." );
}
if (!username || !password){
document.write( "Not successful" );
}
if (username !== "gfg" && password !== "gfg" && username !== "" && password !== "" ) {
alert( "Wrong User Name and Passowrd" );
}
</script>
<?php
echo <p>Hello from GeeksforGeeks!</p>
</div>
</body>
</html>';
?>
|
Once we load it into any server such as the localhost, we can see that it is providing the following output:
Output:
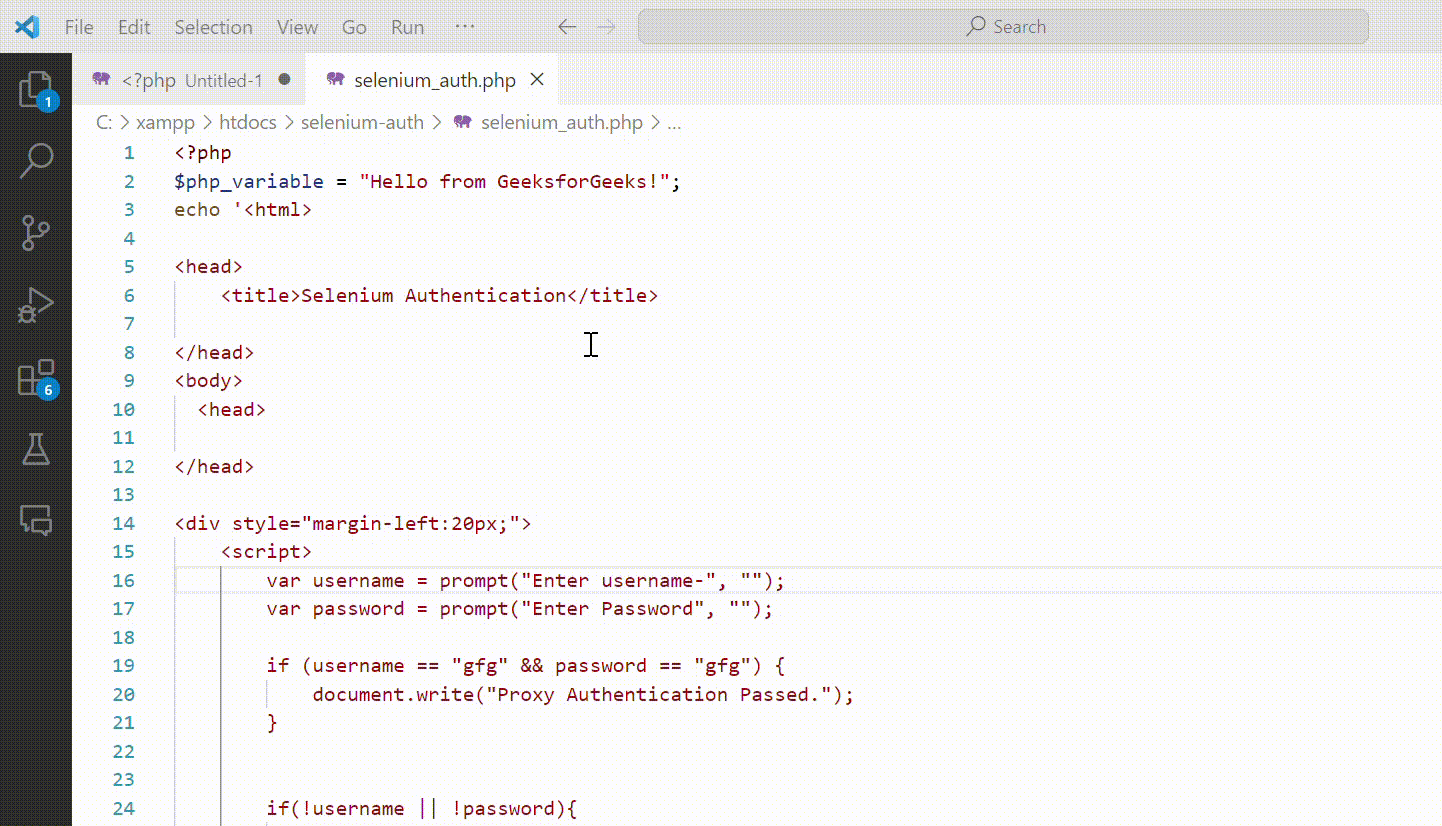
As we can see if we are manually handling the proxy authentication once we provide the username and password as “gfg” it says “proxy authentication passed“, Now let’s learn how to do that using the selenium webdriver automatically once a proxy authentication request has been prompted on our screen.
Example 2: Selenium Webdriver Proxy Authentication
Step 1: Use any JDK-enabled Java editor and load the Selenium JAR files. We will be using the IntelliJ editor to execute the code.
Note: To handle proxy authentication without third-party apps in Selenium, use built-in alerts. Switch to the HTTP proxy authentication alert and pass credentials using “sendKeys” method.
Step 2: After this, we will need to write the Java code that will enter the credentials automatically. We can use the Example 1 authentication file (selenium_auth.php) for demonstration.
Java
package gfg;
import java.io.IOException;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class AlertsDemo {
public static void main(String args[])
throws IOException
{
System.setProperty(
"webdriver.chrome.driver" ,
"C:\\Users\\kisha\\Downloads\\chromedriver_win32\\chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.get(
driver.switchTo().alert().sendKeys( "gfg" );
driver.switchTo().alert().accept();
driver.switchTo().alert().sendKeys( "gfg" );
driver.switchTo().alert().accept();
}
}
|
In this above code, we will only have to make the necessary changes on the driver.get the URL, and the System.set Property so have a look at the URL on your computer and set the path to where you have downloaded the Chromium webdriver.
Step 3: Now when we run will run the Java program in any compiler. This will send two alert keys “gfg” and “gfg“, which will be fetched into the browser and the browser will successfully redirect us to the page where we will see the notification that we successfully managed to do the proxy authentication.
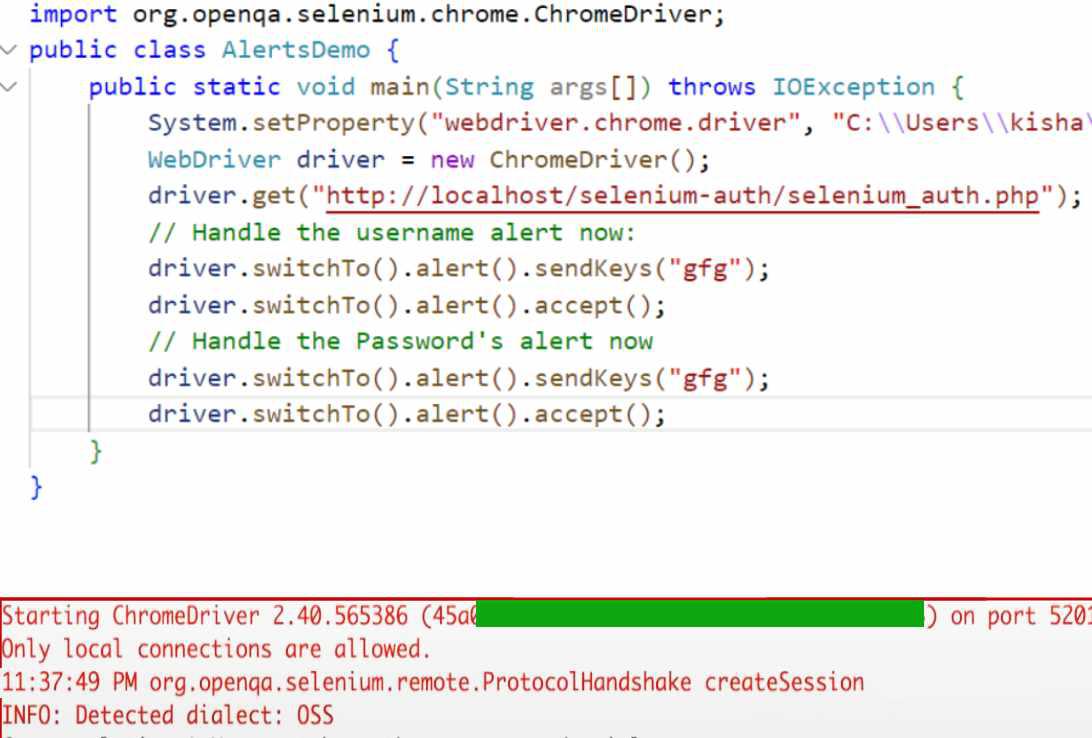
Step 4: We can now see in the output below we have successfully used the alert function provided by the Selenium web driver to handle proxy authentication:
Output:
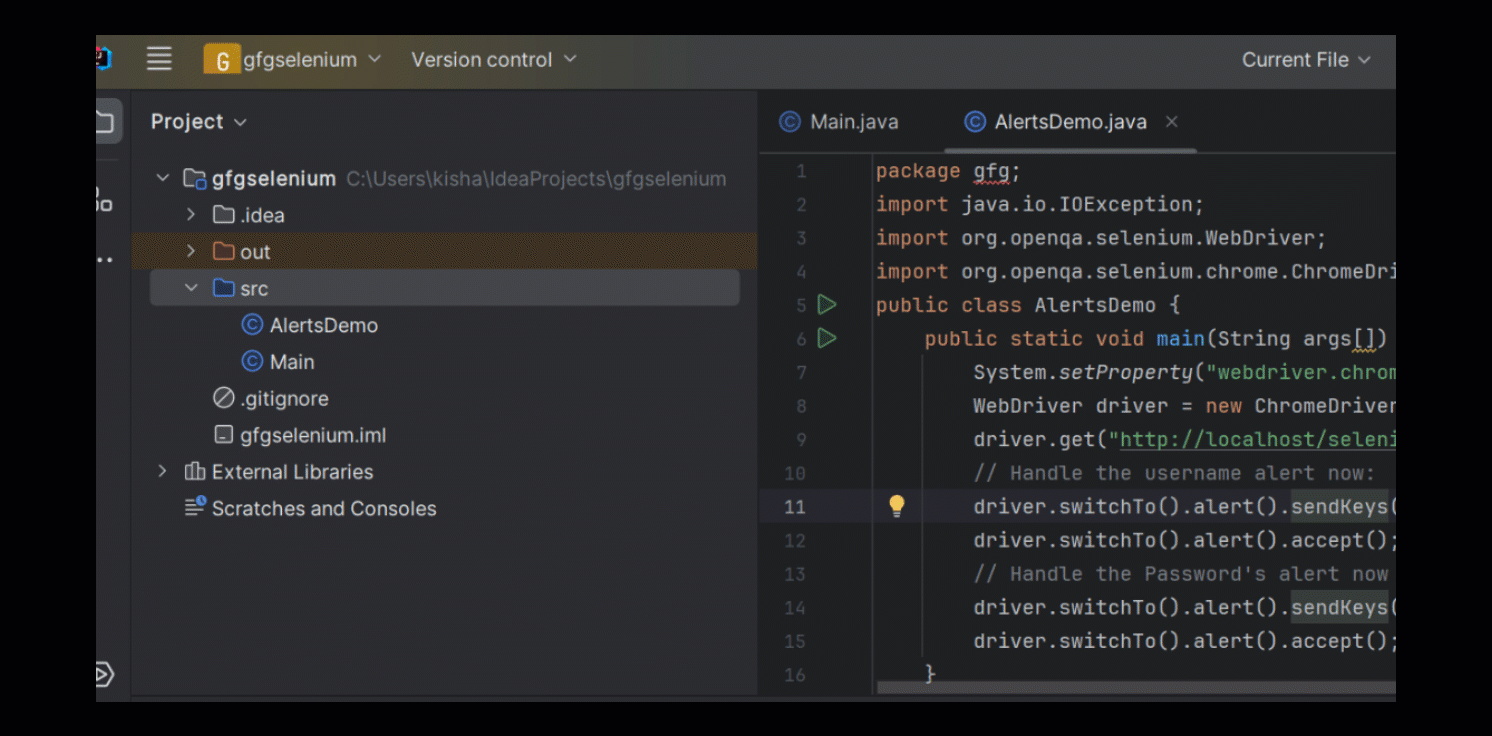
Conclusion
In conclusion, we’ve explored the capabilities of Selenium WebDriver in handling proxy authentication within a web browser using any Java editor with JDK installed. The power of Selenium extends beyond proxy authentication, proving valuable for software web application testing and automation. We encourage you to follow the entire article to avoid any confusion about handling proxy authentication using Selenium WebDriver’s features. If you have any questions or doubts, please feel free to ask in the discussion section of this article.
Share your thoughts in the comments
Please Login to comment...