Selenium is an open-source program that automates web browsers. Selenium Webdriver is mainly used to execute the scripts according to the browser we are using.
Prerequisites
1. Install the Selenium Python Package.
Use the following command to install the Selenium Python package:
pip install selenium
2. Install Chrome Webdriver.
Install the Chrome WebDriver following the steps in the link.
What is a Selenium WebElement?
An HTML element on a website serves as the Selenium WebElement. HTML elements are used in HTML documents. The Web Element can be recognized using various attributes like id, class name, link text, XPath, etc.
- Every HTML element contains both a start tag and an end tag.
- Between the tags is the content.
Example:
Paragraph tag:
<p>This is a paragraph</p>.
The content of the paragraph lies between its opening and closing brackets.
WebElements can be initialized and accessed in many different ways. There are different functions for finding the element on the webpage using its various attributes like id, class name, link text, XPath, etc. For example, find_element_by_link_text(), find_element_by_id(), find_element_by_tag_name(), find_element_by_xpath(), find_element_by_class_name(), etc.
WebElement Commands
Some of the major WebElement commands are as follows:
1. sendKeys() Command
The sendKeys method is used to send any keyboard key to an element on the webpage. This command is used to type something in a text box/input and also press any keys like enter. The command expects an argument of the keys to be sent and does not return anything.
Syntax:
element.send_keys(Keys);
Example:
element.send_keys(Keys.F5);
This will send F5 key, i.e. refresh the webpage.
Now, to find an element and then type a value for the element implementation can be done like this:
element = browser.find_element_by_id(“email”);
element.send_keys(“vvahuja2000@gmail.com”);
Output:
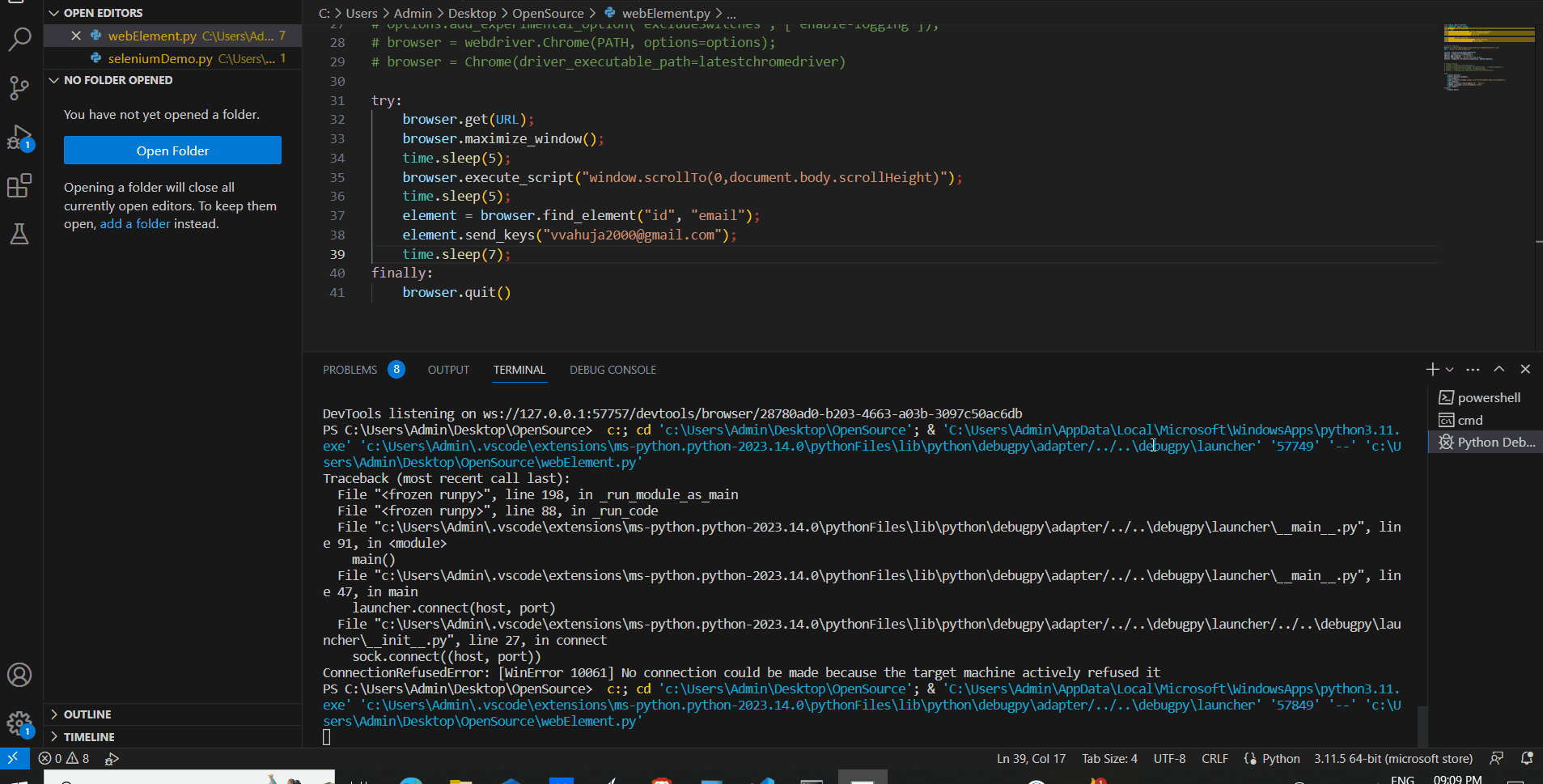
Send Keys command
2. isDisplayed() Command
This method tells if an element is present or not and returns a boolean value of “true” if the element is present and throws a NoSuchElement exception if not present.
Syntax:
element.is_displayed()
Example:
To give examples of both the scenarios. that is if the element is present and absent. The code can be as follows:
displayedElement = browser.find_element_by_id(“email”);
print(displayedElement.is_displayed())
notDisplayedElement = browser.find_element_by_id(“wrongemail”);
print(notDisplayedElement.is_displayed());
Output:
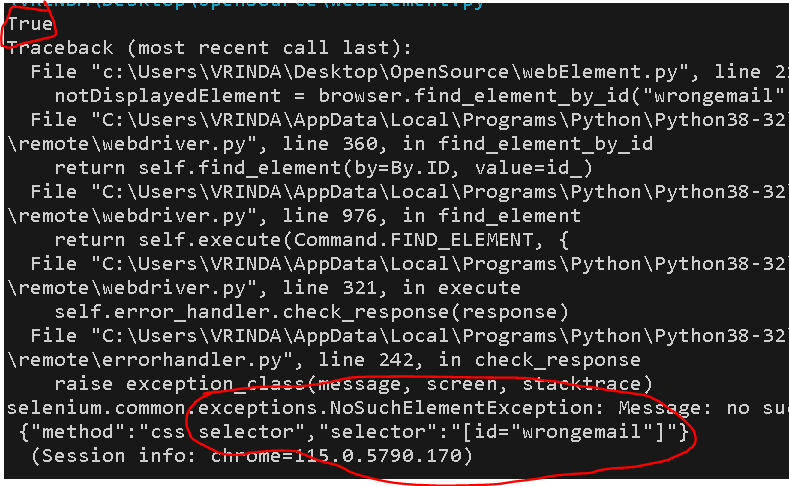
isDisplayed Command
3. isSelected() Command
This method tells if an element is selected or not. It returns a boolean value of “true” if element is selected and “false” if it is not selected. It can be used to check if some link or radio button or checkbox is selected or not.
Syntax:
element.is_selected();
Example:
Below is an example for finding a href element using link text and verifying if it is selected:
element = browser.find_element_by_link_text(“Resume”);
print(element.is_selected());
Output:

isSelected command
4. submit() Command
This command works like a submit button in forms. It is used to submit the form. Here, we find the button element and then submit it.
Syntax:
element.submit();
Example:
Below is an example to find the element(button) to submit the form using the id, and submitting the form:
element = browser.find_element_by_id(“js_send”);
element.submit();
5. isEnabled() Command
This method tells if the element is enabled or not and returns a boolean value depending on that. It returns “true” if the element is enabled.
Syntax:
element.is_enabled();
Example:
Below is an example for finding an element using link text and checking if it is enabled:
element = browser.find_element_by_link_text(“Resume”);
print(element.is_enabled());
Output:

isEnabled() command
6. getLocation() Command
This command gets the location of the element in the form of x and y coordinates in the webpage. It returns the coordinates as a dictionary itself.
Syntax:
element.location;
Example:
Below is an example to get an element by its link text and then get its location:
element = browser.find_element_by_link_text(“VA”);
print(element.location)
Output:
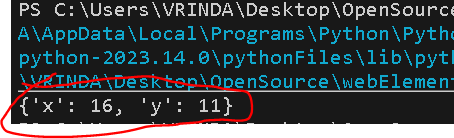
getLocation command
7. clear() Command
Clear command deletes the value of a text element. It only affects if the element is an input or a text area.
Syntax:
element.clear();
Example:
Below is an example to find the element by specific id and then clear the value. Before clearing the value, we add some sleep time to write something in the text box element and then the clear command clears it.
element = browser.find_element_by_id(“email”);
element.clear()
Output:
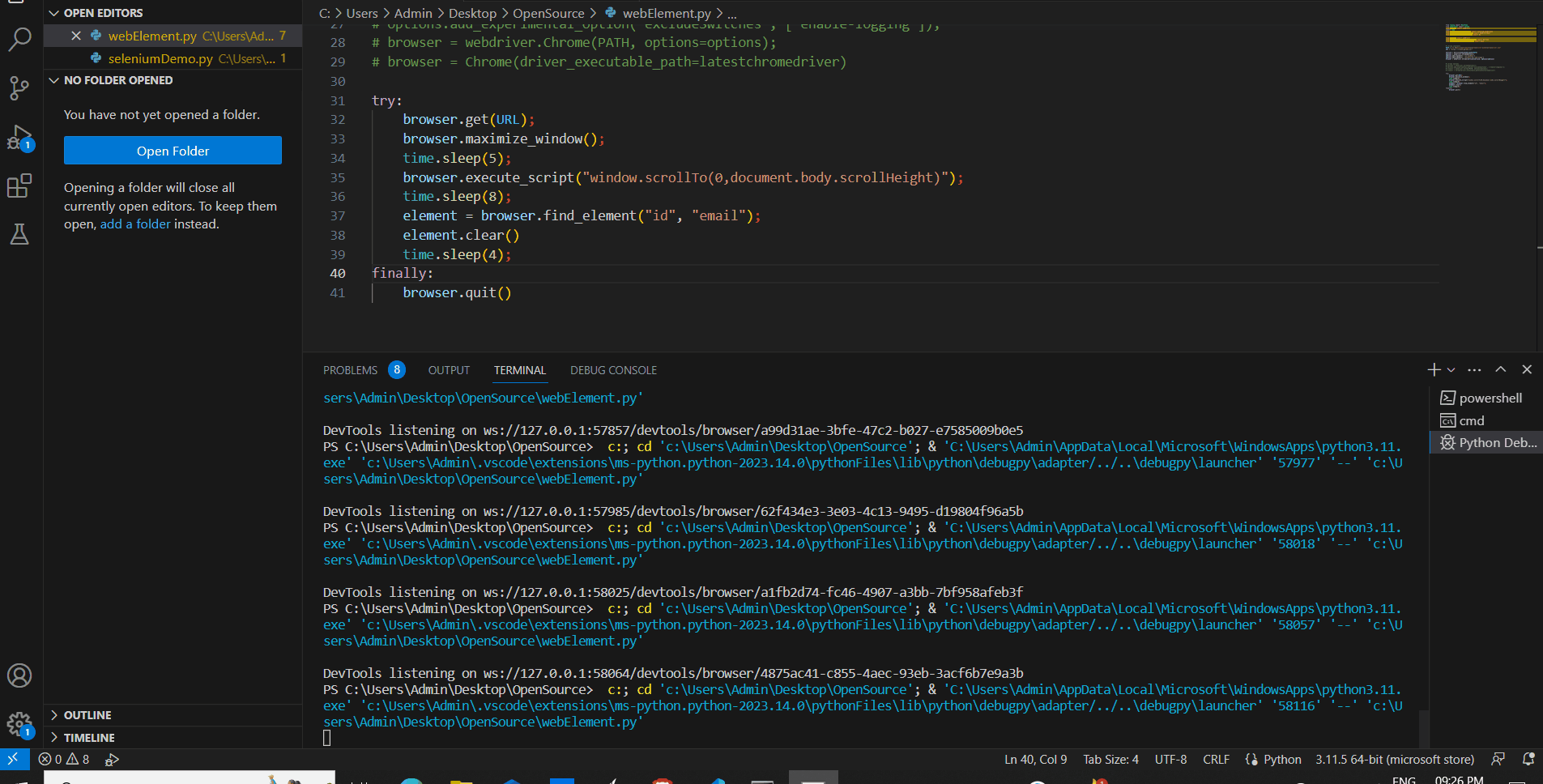
Clear command
8. getText() Command
This command gives us the text of the particular element visible on the webpage.
Syntax:
element.get_attribute(‘text’);
Example:
Here, we find an element using link text and then get the test of the element. This returns the text value itself which is printed on the terminal.
element = browser.find_element_by_link_text(“Resume”);
print(element.get_attribute(‘text’))
Output:

getText command
9. getTagName() Command
This command gives the tag used in the particular element and returns its string value. For example, if an element is an input in a form the tag value will be input.
Syntax:
element.tag_name;
Example:
Here, we have printed the tag value for two different elements:
element = browser.find_element_by_id(“subject”);
print(element.tag_name)
element = browser.find_element_by_id(“about”);
print(element.tag_name)
Output:
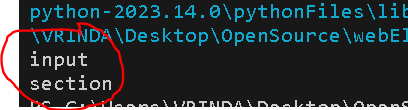
getTagName command
10. getCssValue() Command
This command helps to get the css value of a property of the particular element like color, border, padding, etc. It takes argument of the css property we want value for and returns the value of the CSS property itself.
Syntax:
element.value_of_css_property(CSS_Property);
Example:
Here, we get the value of the css property of “padding-top” for the element:
element = browser.find_element_by_id(“about”);
print(element.value_of_css_property(‘padding-top’));
Output:
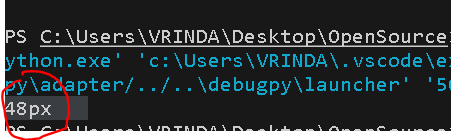
getCssValue command
11. getAttribute() Command
This method is used to get the specific attribute values of the element. It can be used to get the text, href value, some css atribute value, class name, or any attribute of the element. It takes argument of the attribute whose value we need and returns the attribute value itself.
Syntax:
element.get_attribute(Attribute);
Example:
Below is an example to find an element using link text and get the “href” attribute of the element:
element = browser.find_element_by_link_text(“Resume”);
print(“Href for resume: ” + element.get_attribute(“href”));
Output:

getAttribute command
12. click() Command
The click command is used to click on the element. It can be used to click a link, button, or a checkbox.
Syntax:
element.click();
Example:
Here, we find the element with the particular link text and then click on it.
element = browser.find_element_by_link_text(“Work”);
element.click()
Output:
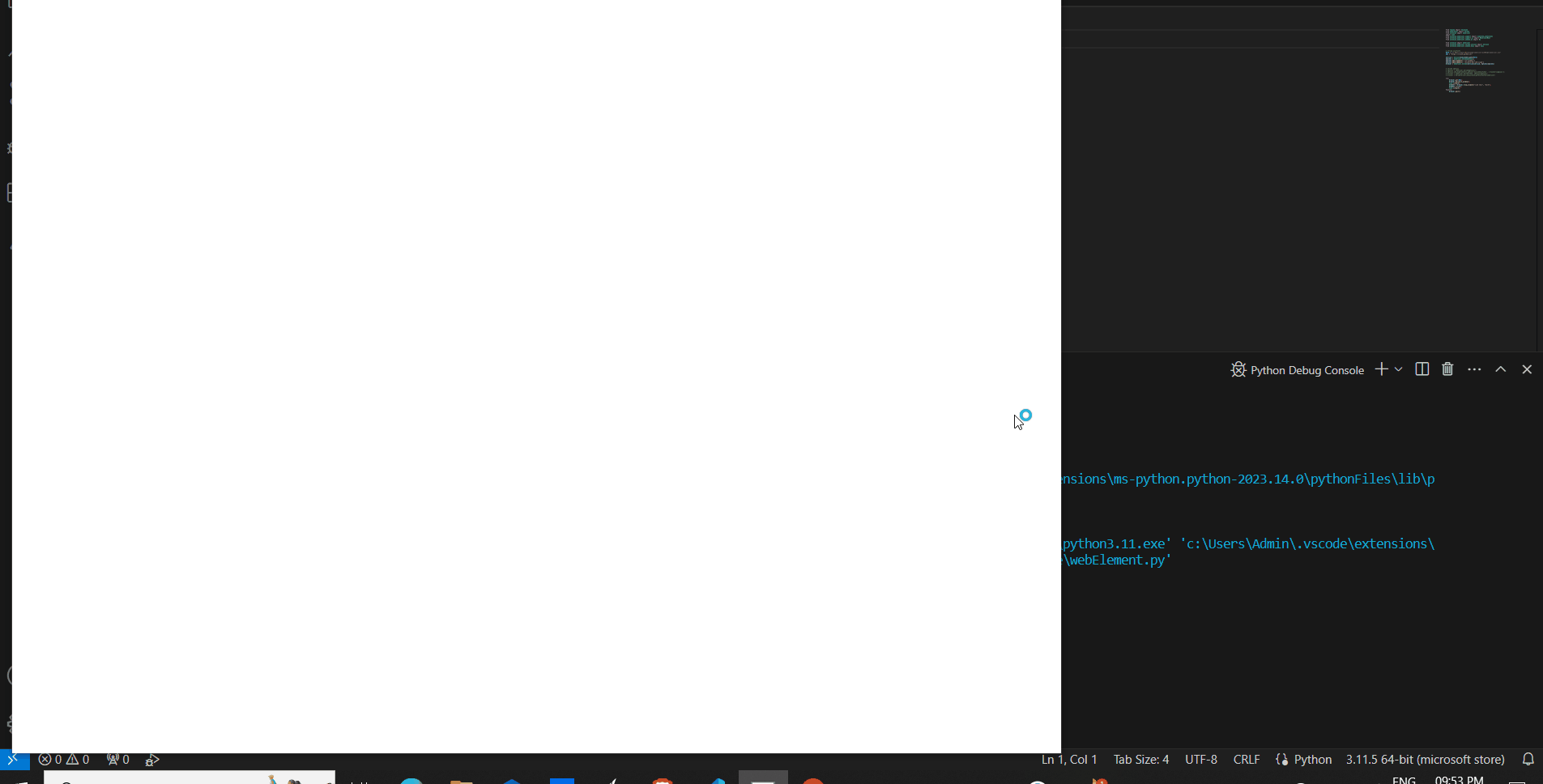
Click Command
13. getSize() Command
This command gives us the size of the specific element in terms of its height and width in pixels. It returns the size as the dictionary of these attributes.
Syntax:
element.size;
Example:
Here, we find the element using link text and print the size of the element.
element = browser.find_element_by_link_text(“VA”);
print(element.size)
Output:
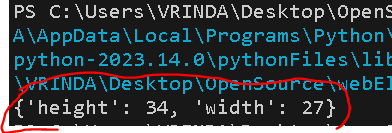
getSize command
Below is the complete Python program to implement WebElement commands:
Python
PATH = r "C:\Users\VRINDA\Desktop\chromedriver-win64\chromedriver.exe"
service = Service(executable_path = PATH)
options = webdriver.ChromeOptions()
options.add_argument( '--no-sandbox' )
options.add_argument( '--disable-dev-shm-usage' )
browser = webdriver.Chrome(service = service, options = options)
try :
browser.get(URL);
browser.maximize_window();
time.sleep( 5 );
time.sleep( 5 );
finally :
browser.quit()
|
Share your thoughts in the comments
Please Login to comment...