Selenium WebDriver – Browser Commands
Last Updated :
11 Oct, 2023
Selenium WebDriver is an extremely useful tool for automating web applications and browser interactions. Through its collection of browser commands, developers and testers can control web browsers in a programmatic fashion. Browser commands that enable tasks such as web scraping, simplifying everyday operations on the web, and testing web applications. As Selenium WebDriver is compatible with a range of programming languages, it is highly accessible among developers of varying levels. The article focuses on discussing Selenium WebDriver Browser Commands in detail.
What are Browser Commands?
Selenium WebDriver provides predefined methods and functions that enable developers to interact directly with browsers.
- These commands provide exact control over the browser’s actions, like loading specific pages, extracting details such as page titles and URLs, accessing page source code, and controlling browser windows.
- Browser commands provide an easy way to interact with web applications and to perform automation, and scraping data from web pages.
- The Browser Command provides methods: get(), getTitle(), getCurrentUrl(), getPageSource() , close() and quit().
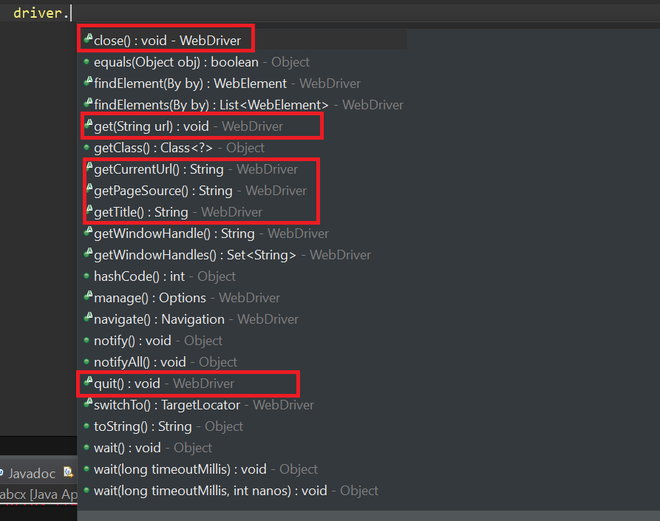
Prerequisites
1. Java Development Kit (JDK): Install the JDK to write and execute Java code.
2. Integrated Development Environment (IDE): Choose an IDE like Eclipse, IntelliJ IDEA, or Visual Studio Code for writing and managing your Java projects.
3. Selenium WebDriver Java Client JAR Files: These files enable you to interact with the Selenium WebDriver API using Java.
4. WebDriver Executable: Download the WebDriver executable for your preferred browser (e.g., ChromeDriver, GeckoDriver) to automate browser actions.
5. Dependencies: Configure the required dependencies in your Java project to use Selenium WebDriver.
6. Import the required packages.
org.openqa.selenium.WebDriver
org.openqa.selenium.chrome.ChromeDriver
7. Set the system property for ChromeDriver (path to chromedriver executable).
System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver.exe”);
8. Create the instance of the ChormeDriver.
WebDriver driver = new ChromeDriver();
For detailed installation steps, refer to this article.
Brower Commands
1. get(String url) command
It loads a new web page in the current browser window and accepts a string parameter that specifies the URL of the web page to be loaded.
Method:
driver.get(“https://www.geeksforgeeks.org/”);
or
String URL = “https://www.geeksforgeeks.org/”;
driver.get(URL);
Output:
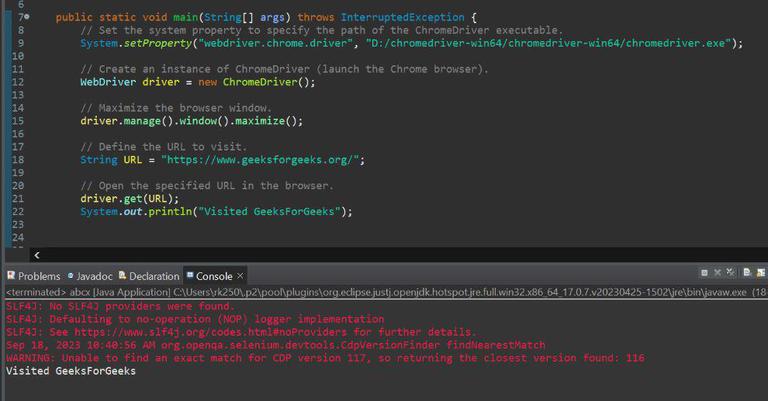
Explanation:
- This command will start the loading of a new web page in the web browser that is currently open.
- It takes one argument in the form of a string which contains the URL of the page desired to be retrieved.
- This method is identical to the driver.navigate().to() which works for the same purpose.
- But, the driver.get() method waits until the page is completely finished loading before returning control back to the script whereas driver.navigate().the to() does not wait for the page to load and terminates quickly.
2. getTitle() Command
It gets the title of the current web page displayed in the browser. It does not accept any parameters. It returns the title of the specified URL as a string.
Method:
String pageTitle = driver.getTitle();
System.out.println(pageTitle);//it will print the title of the specified URL.
Output:
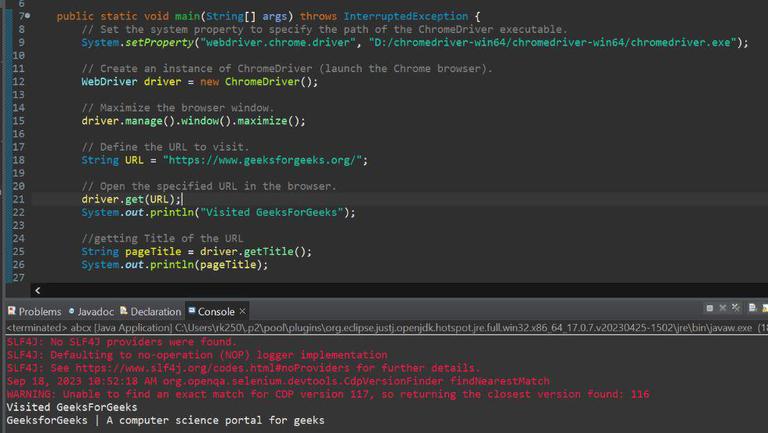
Explanation:
- This command obtains the title of the active webpage which will be same as displayed in the browser.
- It does not take any arguments and it return the title of the specified URL as a string.
- It can be utilized to access the title of the URL, which is be convenient for the purpose of confirmation in automated tests and for logging as well as reporting.
3. getCurrentUrl() Command
It gets the URL of the current web page shown in the browser. It does not accept any parameters and returns the URL as a string.
Method:
String currentURL = driver.getCurrentUrl();
Output:
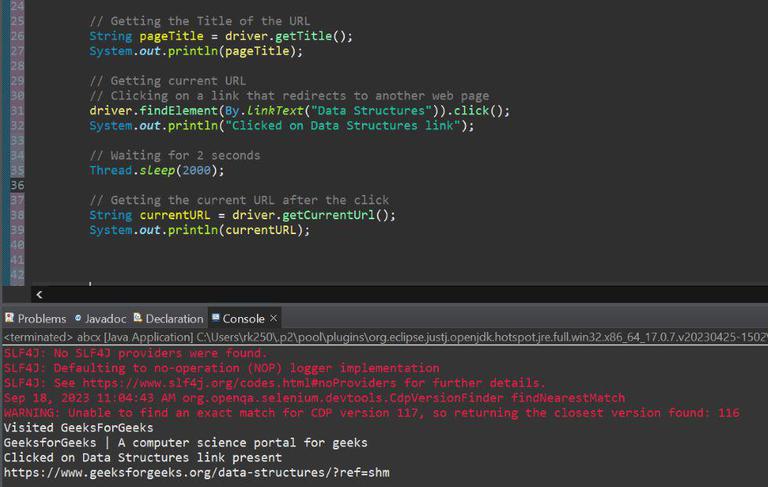
Explanation:
- This technique obtains the web address of the present page.
- No arguments are accepted and it return the URL of the webpage as a string.
- This technique is similar to clicking within the search field of the browser and copying the link from the search area.
- It can be utilized to validate the present URL, which is very expedient for the function of corroboration in automated tests and for logging as well as reporting.
4. getPageSource() Command
It gets the entire page source of the current web page loaded in the browser. It does not accept any parameters but it do returns the page source as a string.
Method:
String pageSource = driver.getPageSource();
Output:
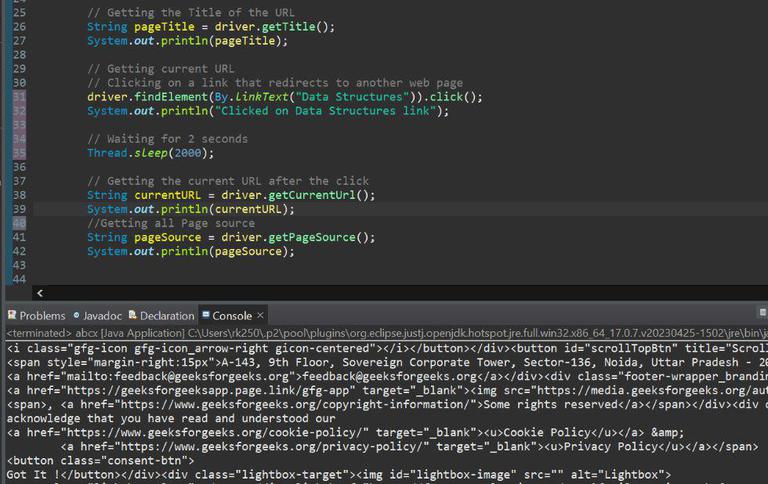
Explanation:
- This technique returns the entirety of the page source code of the existing URL.
- Taking in no parameters, it returns the page source as a string.
- This method corresponds to clicking anywhere on the current tab or browser window then picking ‘View page source’ or hitting CTRL + U on the keyboard.
- It’s mostly used for extracting data (web scraping), diagnosing issues, and verifying page content.
5. close() Command
It closes the current browser window or tab. Also, this command does not accept any types of parameters. It also does not return anything.
Method:
driver.close();
Output:
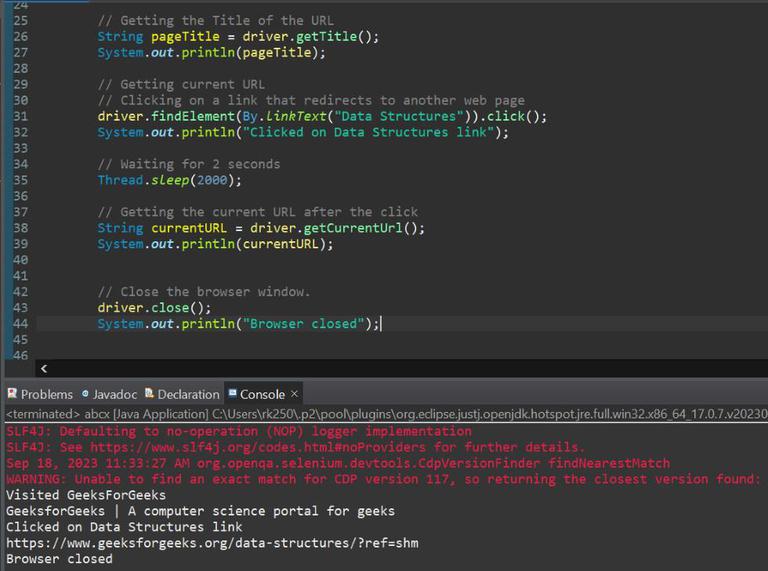
Explanation:
- This approach shuts down the present window or tab of the browser.
- No parameters are accepted and nothing is returned.
- The said method is equal to clicking on the X of the active tabs or pressing CTRL + W on the keyboard.
- It is available to be utilized for closing specific browser windows or tabs while operating with several tabs in a single WebDriver session.
6. quit() Command
It closes all the browser windows and tabs for a particular WebDriver session. This command does not accept any parameters and not return anything.
Method:
driver.quit();
Output:
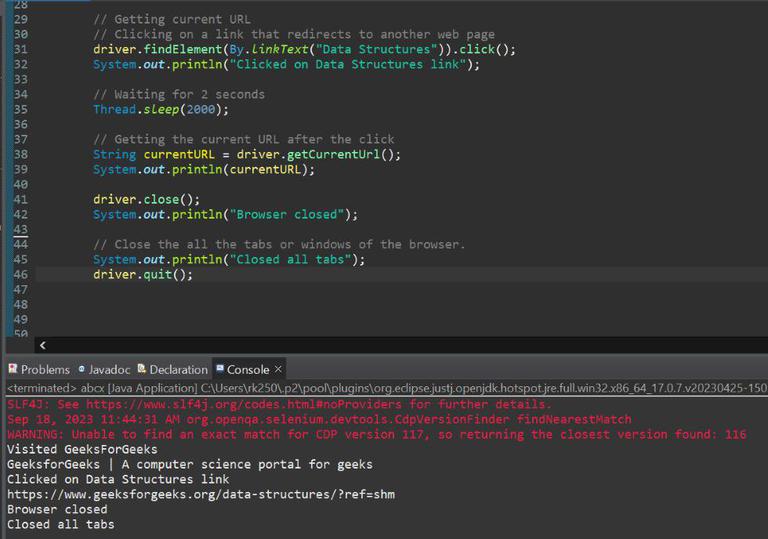
Explanation:
- This method quits the WebDriver session, closing all open browser windows and tabs associated with that session.
- It does not accept any parameters and does not return anything.
- This method is equivalent to clicking on the cross mark present in the top rightmost corner or pressing the ALT + F4 key on the keyboard.
- It can be used to end a WebDriver session and release browser resources.
Implementation
Interaction with the GeeksForGeeks Website using Brower Commands:
- It starts by initializing the ChromeDriver and navigating to the GFG Home page.
- After waiting for 2 seconds, get the Title of the current URL.
- The program then waits for another 2 seconds, it clicks on the “Data Structures” link on the GeeksforGeeks website, which redirects to another web page.
- After waiting for 2 seconds, it will get the URL of the redirected link.
- The program waits for another 2 seconds and closes the current tab or window only, using the driver.close() command and display “Browser closed” in the console.
- Lastly, the program quits the Webdriver session and closes all the open tabs in the browser.
Below is the Java program to implement the above approach:
Java
package Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class BrowerCommands {
public static void main(String[] args)
throws InterruptedException
{
System.setProperty(
"webdriver.chrome.driver" ,
"D:/chromedriver-win64/chromedriver-win64/chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
Thread.sleep( 2000 );
driver.get(URL);
System.out.println( "Visited GeeksForGeeks" );
Thread.sleep( 2000 );
String pageTitle = driver.getTitle();
System.out.println(pageTitle);
Thread.sleep( 2000 );
driver.findElement(By.linkText( "Data Structures" ))
.click();
System.out.println(
"Clicked on Data Structures link" );
Thread.sleep( 2000 );
String currentURL = driver.getCurrentUrl();
System.out.println(currentURL);
Thread.sleep( 2000 );
driver.close();
System.out.println( "Browser closed" );
System.out.println( "Closed all tabs" );
driver.quit();
}
}
|
Output:
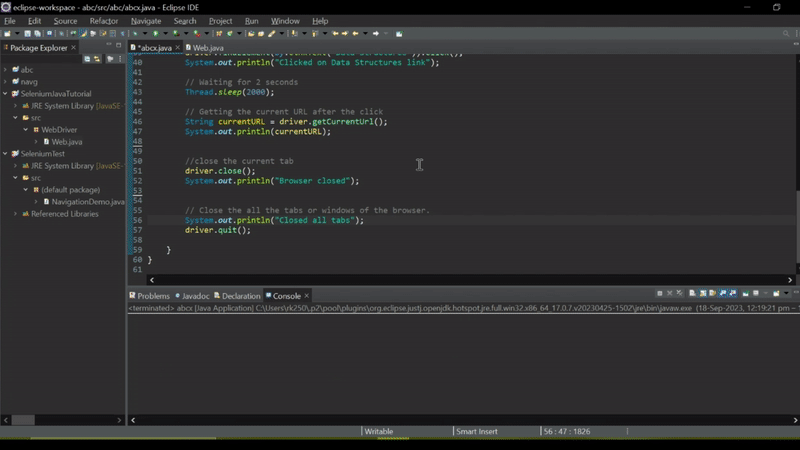
Conclusion
Browser commands available in Selenium WebDriver, including get(), getTitle(), getCurrentUrl(), getPageSource(), close(), and quit() are important for retrieving the information and also control the browser behaviour. Browser commands provide easy way to interact with web application and to perform automation, and scraping data on the web pages.
Share your thoughts in the comments
Please Login to comment...