Selenium WebDriver – Navigation Commands
Last Updated :
16 Oct, 2023
Selenium WebDriver is quite a popular open-source framework used for automating web browsers. It helps the developers to automate web-based operations and interactions by creating code in Java, Python, C#, and others. When automating tests, Selenium WebDriver offers a set of navigation commands that let you interact with and manage a web browser’s navigation. In this article, we will learn about the navigation commands in detail.
What are Navigation Commands?
Navigation commands in Selenium WebDriver perform operations that involve navigating through web pages and controlling browser behavior. These commands provide an efficient way to manage a browser’s history and perform actions like going back and forward between pages and refreshing the current page.
The Navigation Command provides four methods: to(), back(), forward(), and refresh(). These methods allow the WebDriver to perform the following operations:
1. to() Command
Loads a new web page in the current browser window. It accepts a string parameter that specifies the URL of the web page to be loaded.
2. back() Command
Moves back one step in the browser’s history stack. It does not accept any parameters and does not return anything.
3. forward() Command
Moves forward one step in the browser’s history stack. It does not accept any parameters and does not return anything.
4. refresh() Command
Reloads the current web page in the browser window. It does not accept any parameters and does not return anything.
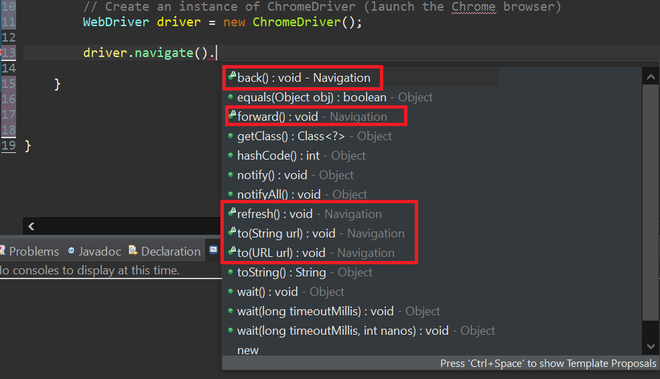
Prerequisites
1. Java Development Kit (JDK): Install the JDK to write and execute Java code.
2. Integrated Development Environment (IDE): Choose an IDE like Eclipse, IntelliJ IDEA, or Visual Studio Code for writing and managing your Java projects.
3. Selenium WebDriver Java Client JAR Files: These files enable you to interact with the Selenium WebDriver API using Java.
4. WebDriver Executable: Download the WebDriver executable for your preferred browser (e.g., ChromeDriver, GeckoDriver) to automate browser actions.
5. Dependencies: Configure the required dependencies in your Java project to use Selenium WebDriver.
6. Import the required packages.
org.openqa.selenium.WebDriver
org.openqa.selenium.chrome.ChromeDriver
7. Set the system property for ChromeDriver (path to chromedriver executable).
System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver.exe”);
8. Create the instance of the ChormeDriver.
WebDriver driver = new ChromeDriver();
For detailed installation steps, refer to this article.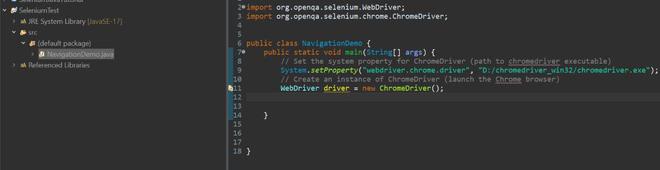
Navigation Commands
1. Navigate to() command
Method:
driver.get(“https://www.geeksforgeeks.org/”);
OR
driver.navigate().to(“https://www.geeksforgeeks.org/”);
Output:
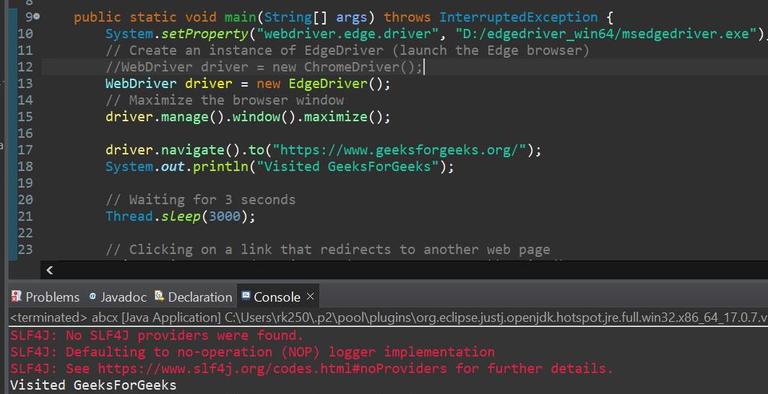
Explanation:
- This method loads a new web page in the current browser window.
- It accepts a string parameter that specifies the URL of the web page to be loaded.
- This method is equivalent to the driver.get() method, which also loads a new web page in the current browser window.
- However, the driver.get() method waits for the page to load completely before returning control to the script, while the driver.navigate().to() method does not wait for the page to load and returns immediately.
Therefore, the driver.navigate().to() method is faster than the driver.get() method, but it may cause synchronization issues if the script tries to interact with elements that are not yet loaded on the page.
2. back() Command
Method:
driver.navigate().back();
Output:
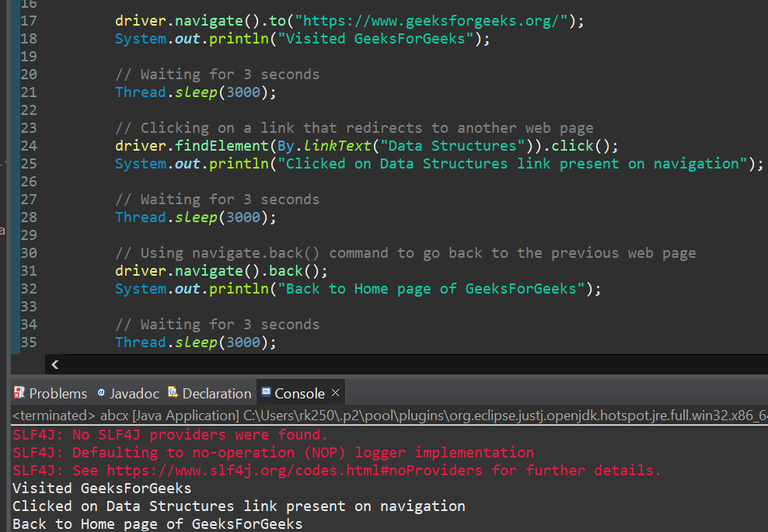
Explanation:
- This method moves back one step in the browser’s history stack.
- It does not accept any parameters and does not return anything.
- This method is equivalent to clicking on the back button of the browser.
- It can be used to return to the previous web page that was visited in the current browser session.
3. forward() Command
Method:
driver.navigate().forward();
Output:
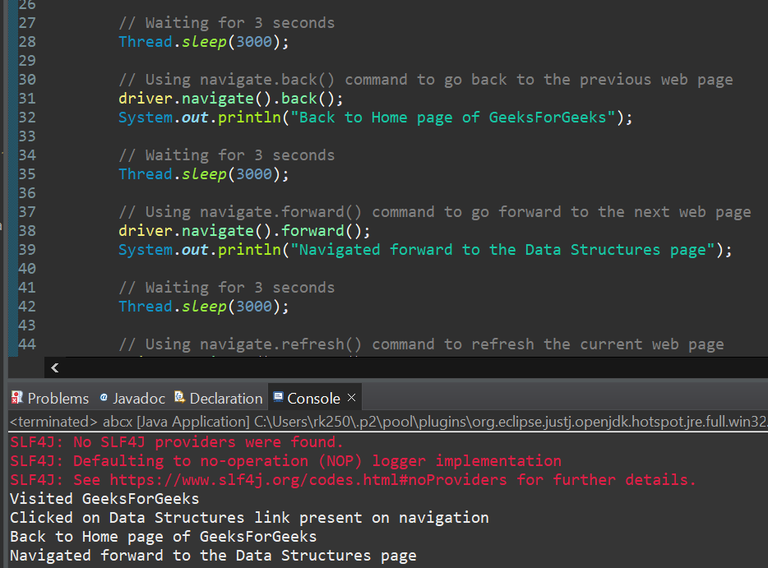
Explanation:
- This method moves forward one step in the browser’s history stack.
- It does not accept any parameters and does not return anything.
- This method is equivalent to clicking on the forward button of the browser.
- It can be used to go to the next web page that was visited in the current browser session after using the back() method.
4. refresh() Command
Method:
driver.navigate().refresh();
Output:
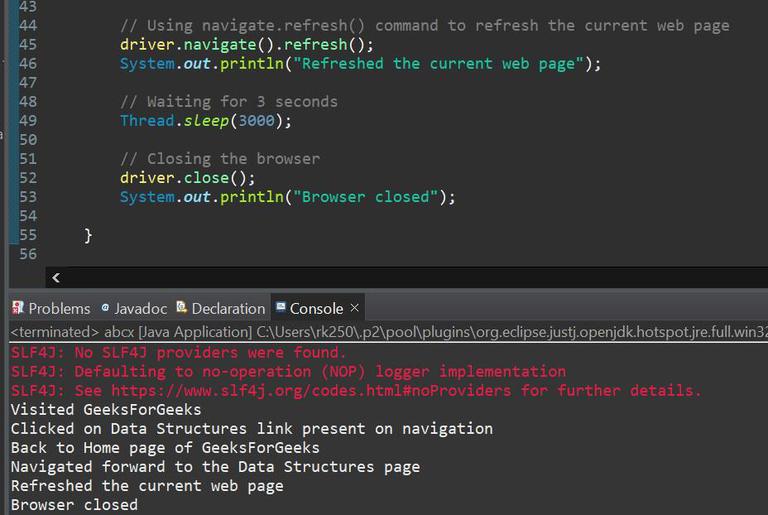
Explanation:
- This method reloads the current web page in the browser window.
- It does not accept any parameters and does not return anything.
- This method is equivalent to clicking on the refresh button of the browser or pressing F5 key on the keyboard.
- It can be used to refresh the content of the web page or to retry a failed operation.
Implementation
Interaction with GeeksForGeeks Website using Navigation Commands:
- It starts by initializing the ChromeDriver and navigating to the GFG Home page.
- After waiting for 3 seconds, it clicks on the “Data Structures” link on the GeeksforGeeks website, which redirects to another web page.
- The program then waits for another 3 seconds before using the navigate().back() command to go back to the previous page (Home page of GeeksforGeeks).
- After waiting for 3 seconds, the navigate().forward() command is used to navigate forward to the “Data Structures” page again.
- The program waits for another 3 seconds and then uses the navigate().refresh() command to refresh the current web page.
- Lastly, the program closes the browser using the driver.close() command and displays “Browser closed” in the console.
Below is the Java program to implement the above approach:
Java
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class NavigationDemo {
public static void main(String[] args)
throws InterruptedException
{
System.setProperty(
"webdriver.chrome.driver" ,
"D:/chromedriver_win32/chromedriver.exe" );
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
driver.navigate().to(
System.out.println( "Visited GeeksForGeeks" );
Thread.sleep( 3000 );
driver.findElement(By.linkText( "Data Structures" ))
.click();
System.out.println(
"Clicked on Data Structures link present on navigation" );
Thread.sleep( 3000 );
driver.navigate().back();
System.out.println(
"Back to Home page of GeeksForGeeks" );
Thread.sleep( 3000 );
driver.navigate().forward();
System.out.println(
"Navigated forward to the Data Structures page" );
Thread.sleep( 3000 );
driver.navigate().refresh();
System.out.println(
"Refreshed the current web page" );
Thread.sleep( 3000 );
driver.close();
System.out.println( "Browser closed" );
}
}
|
Output:
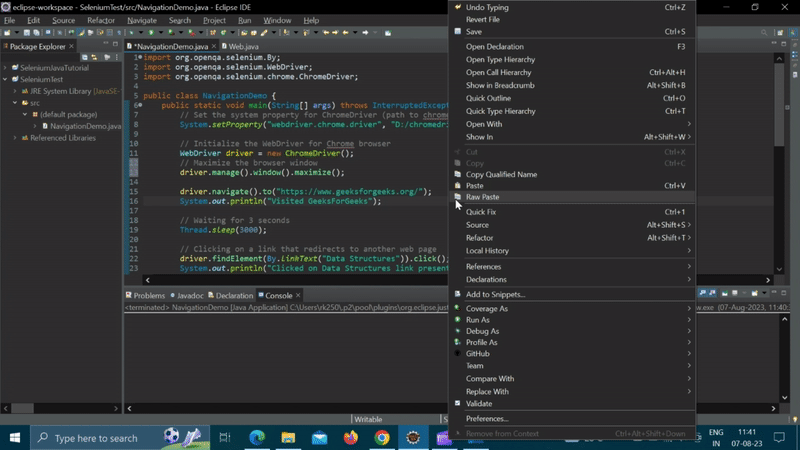
Conclusion
Navigation commands available in Selenium WebDriver, including to(), back(), forward(), and refresh() are crucial for controlling the browser’s history and movements while automating web tasks, ensuring accurate and dependable web testing results.
Share your thoughts in the comments
Please Login to comment...