HostListener Decorators in Angular 17
Last Updated :
05 Apr, 2024
Decorators are declarative functions in Angular that are used to change classes, methods, or properties. They serve as a source of metadata that instructs Angular on how to handle a class.
What is HostListener Decorator?
The @HostListener
decorator in Angular provides a convenient way to listen for events on the host element of a component. It allows to define event handlers directly within the component class. In order to listen to events on the host element of the directive or component, one uses Angular’s @HostListener decorator. This decorator function takes an event name as a parameter. The related function is called by the host element when that event is fired.
Syntax :
@component({
selector: ['appExampleDirective']
})
export class ExampleDirective {
@HostListener('event') methodName() {
//code to execute when the event occurs
}
}
Features of HostListener
- Better Type Inference: Angular 17 reduce the requirement for manual type annotations by providing improved type inference for event arguments in @HostListener decorators.
- Event Modifier Support: To provide more streamlined event processing, it might be improved to include event modifiers like .prevent, .stop in @HostListener decorators.
- Dynamic Event Binding: Using @HostListener, Angular 17 may bring the capability to dynamically bind event listeners, opening up more adaptable event handling scenarios.
- Conditional Event Handling: Depending on specific requirements inside the component or directive, modifications may be made to enable conditional event handling.
Uses of HostListener
- Event Parameterization: Event data like coordinates, target element, key codes, etc. may be accessed by capturing event objects with @HostListener and giving them as parameters to event handler functions.
- Type Safety: TypeScript’s @HostListener reduces the possibility of runtime errors by enabling you to declare the type of event objects being supplied to the event handler methods.
- Integration with Directives: To attach event listeners to host elements and define associated behavior based on user interactions or other events, @HostListener is frequently used within directives.
- Dynamic Event Binding: To handle events more adaptably, @HostListener can be used to dynamically bind event listeners based on component state or other criteria.
Steps to Create Angular Project
Step 1: Create the angular project using the following command.
ng new app_name
- Which stylesheet format would you like to use ? – CSS
- Do you want to enable Server-Side Rendering (SSR) and Static Site Generation (SSG/Prerendering) ? – yes .
Step 2: Open Your project and go to terminal to start the application.
ng serve
Folder Structure:
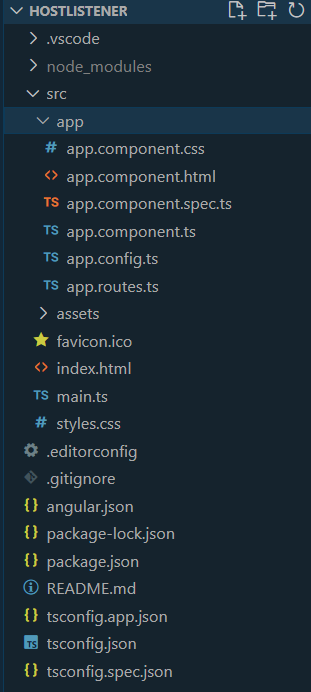
HostListener Decorators in Angular 17
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Example: This example shows the implementation of hostListners decorators.
HTML
<!-- app.component.html -->
<div style="text-align: left;
color: brown; background-color: yellowgreen">
<h1>Welcome to learn about Decorators</h1>
</div>
<button [disabled]="buttonDisabled">Click me</button>
JavaScript
//app.component.ts
import { Component, HostListener } from '@angular/core';
import { RouterOutlet } from '@angular/router';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
export class AppComponent {
title = 'decorator';
buttonDisabled = false;
@HostListener('click', ['$event.target'])
onClick(target: HTMLElement) {
if (target.tagName === 'BUTTON') {
this.toggleButton();
}
}
toggleButton() {
this.buttonDisabled = !this.buttonDisabled;
}
}
Output :
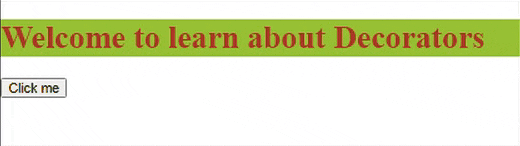
Share your thoughts in the comments
Please Login to comment...