Difference between @Injectable and @Inject Decorators
Last Updated :
01 Apr, 2024
In Angular, decorators play an important role in defining and managing dependencies within the application. Two commonly used decorators are @Injectable
and @Inject
. While they both relate to dependency injection, they serve different purposes and are applied to different elements within an Angular application.
What are @Injectable Decorators ?
@Injectable
is a decorator in Angular used to mark a class as an injectable that allows it to be provided and injected as a dependency within other Angular components, services, or modules.
Syntax:
@Injectable({
providedIn: 'root',
})
export class ServiceClass {
constructor() {}
}
Features of @Injectable decorators
- Singleton Pattern: Creates a single instance of a service to be shared across the application.
- Reusability: Services are designed to encapsulate certain capabilities, making integration and reuse simple.
- Lazy Loading: Optimizes performance by loading services only when needed, reducing initial load times.
Steps to Create Angular Project
Step 1: Create a new Angular Project using the following command
ng new gfg-decorators
Step 2: Create a new service
ng generate service gfg
Folder Structure:
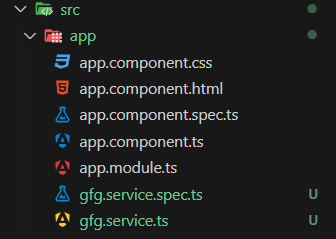
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Example:
JavaScript
// gfg.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class GfgService {
constructor() { }
greetUser(user: string): string {
return `Hello ${user} from GfgService`;
}
}
We created a method greetUser(user: string), which accepts the username as a parameter and will return a greeting message with the username.
Output:
The ‘@Injectable’ decorator makes no apparent changes to itself. Instead, it acts as a label in Angular, which means that a particular class can be assigned to other parts of the code. As a result, you won’t get any output if you only use ‘@Injectable’. It’s more like telling Angular “Hey, this class is there to be used elsewhere!”
What are @Inject Decorators ?
@Inject
is a decorator used to specify the token (dependency) to be injected into a class constructor or a provider definition. It specifies dependencies explicitly for Angular’s DI system when automatic resolution is not possible, helping to resolve dependencies based on the specified token.
Features of @Inject decorators
- Decoupling: Enables dependencies and components to be coupled less tightly.
- Parameter Injection: Injecting dependencies into constructors or methods without relying on TypeScript’s type inference.
- Clearer Intent: Enhances readability by indicating which dependencies are being injected into a component or service.
Syntax:
export class MyComponent {
constructor(@Inject(ServiceClass) private myService: ServiceClass) {}
}
Now Create a new component:-
ng generate component gfgcomponent
Folder Structure:
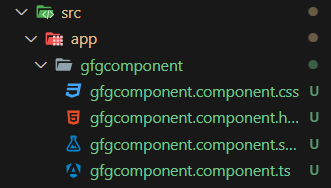
A new component will be created in app folder
Example:
HTML
<!-- gfgcomponent.component.html -->
<p>{{greetingMessage}}</p>
JavaScript
// gfgcomponent.component.ts
import { Component, Inject } from '@angular/core';
import { GfgService } from '../gfg.service';
@Component({
selector: 'app-gfgcomponent',
templateUrl: './gfgcomponent.component.html',
styleUrls: ['./gfgcomponent.component.css']
})
export class GfgcomponentComponent {
constructor(@Inject(GfgService) private gfgService: GfgService) { }
greetingMessage = "";
ngOnInit() {
this.greetingMessage = this.gfgService.greetUser("GFGUser")
}
}
- The GfgService is injected into the component using the @Inject decorator in the constructor.
- Next, we can use greetUser() method from GfgService, and we save the resultant greeting message in the greetingMessage variable.
- You can now access and see this welcome message in the HTML template of the component.
Output:
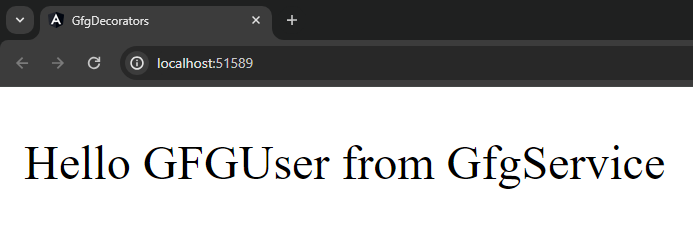
Output on browser
Difference between @Injectable and @Inject decorators
Feature
| @Injectable Decorator
| @Inject Decorator
|
---|
Purpose
| Marks a class as eligible for dependency injection.
| Specifies a dependency token for manual injection.
|
Usage
| Applied to service classes or providers.
| Applied to constructor parameters to specify injection dependencies.
|
Dependency Scope
| Defines the metadata needed for DI resolution.
| Specifies the exact dependency to be injected.
|
Dependency Type
| Marks a class as a candidate for DI resolution.
| Directs DI to inject a specific dependency instance.
|
Example
| @Injectable({ providedIn: ‘root’ })
| constructor(@Inject(MyDependency) private dependency: MyDependency)
|
Conclusion
In summary, @Injectable
is used to mark a class as injectable, that allows it to be managed by Angular’s dependency injection system, while @Inject
is used to specify the token (dependency) to be injected into a class constructor or a provider definition. Understanding the difference between these decorators is important for effectively managing dependencies and implementing dependency injection in Angular applications.
Share your thoughts in the comments
Please Login to comment...