FastAPI – OpenAPI
Last Updated :
04 Nov, 2023
FastAPI is a modern, fast, and web framework for creating APIs with Python. It is built on top of the popular web framework Starlette and includes built-in support for OpenAPI. In this article, we’ll learn about OpenAPI and FastAPI and how to use them to create robust, widely documented APIs.
What is OpenAPI?
OpenAPI (formerly Swagger) is a specification for describing APIs in a standard and machine-readable format. It allows you to define your API’s endpoints, parameters, responses, and security parameters. We’ll learn how to use OpenAPI to set API endpoints, parameters, response, and security parameters, as well as how to use FastAPI to implement APIs.
- OpenAPI schema: An application programming interface (API) is encapsulated in a JSON object called an OpenAPI schema. It includes details of the API endpoints, responses, and security requirements of the API.
- Path operation: A path operation is a mapping of an HTTP method to a path. For example, the GET /users path operation would be used to retrieve a list of all users.
- Parameter: A parameter is a piece of data that is passed to the API endpoint. Parameters can be passed in a path, query string, request body, or header.
- Response: The response is the data that is returned by the API endpoint. Responses can be in any format, such as JSON, XML, or HTML.
- Security requirement: Security requirements are a way to protect API from unauthorized access. FastAPI supports various security schemes, such as OAuth2 and HTTP Basic Auth.
Benefits of using FastAPI and OpenAPI
- OpenAPI documentation helps developers understand and use the API more easily. It can also help developers identify and fix errors in their API code.
- OpenAPI client libraries can save developers time from writing code to interact with the API.
- OpenAPI is a widely supported standard, so there are many tools available that can be used with OpenAPI-powered APIs.
Document a FastAPI App with OpenAPI
The following example shows a simple FastAPI API with a single path operation:
Document FastAPI Endpoint
This code creates a simple FastAPI application with a Endpoint at the root URL that returns a JSON message “Hello, GeeksforGeeks!” when you run the script and access http://localhost:8000 in your web browser.
Python3
from fastapi import FastAPI
import uvicorn
route = FastAPI()
@route .get( "/" )
async def root():
return { "message" : "Hello, GeeksforGeeks!" }
if __name__ = = "__main__" :
uvicorn.run(route, host = "0.0.0.0" , port = 8000 )
|
Deployement of the Project
Execute your FastAPI application and make sure it is running on your local machine.uvicorn your_app_name:app –reload
uvicorn <app_name>:app --reload
Replace <app_name> with the name of your FastAPI application file. For example, run the following command, assuming that geeksforgeeks_fastapi_openapi.py is the name of the FastAPI application file:
uvicorn geeksforgeeks_fastapi_openapi:route --reload
The –reload flag tells Uvicorn to automatically reload when the application code changes.
Output
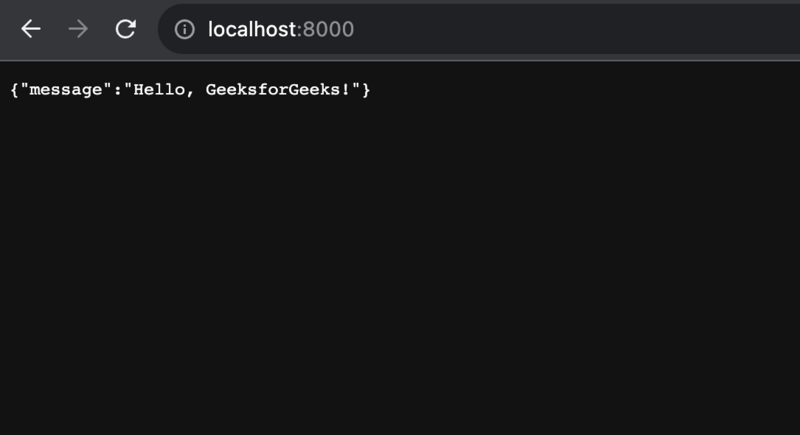
This is a simple FastAPI application that creates an /endpoint. This endpoint handles GET requests, and the application responds with “Hello, GeeksforGeeks!”. And sends messages in return. After running the FastAPI application, open your browser and visit http://127.0.0.1:8000/docs to access the automatically created documentation and Swagger UI.
.png)
FastAPI – Swagger UI
This is a basic FastAPI API OpenAPI schema for a GET request to the root path, which returns the message “Hello, GeeksforGeeks!” in a JSON response.
Share your thoughts in the comments
Please Login to comment...