FastAPI – Cookie Parameters
Last Updated :
13 Oct, 2023
FastAPI is a cutting-edge Python web framework that simplifies the process of building robust REST APIs. In this beginner-friendly guide, we’ll walk you through the steps on how to use Cookie in FastAPI.
What are Cookies?
Cookies are pieces of data stored on the client browser by the server when the user is browsing the web page. Multiple cookies can be stored on the user’s browser by the server during the session. Cookies can be of different types such as session cookies, persistent cookies, etc.
Use of Cookies
- Session management: Cookies can store session IDs to track a user’s session on a website.
- User authentication: Cookies may contain authentication tokens or user information to authenticate and remember users.
- Personalization: Cookies can store your preferences and settings.
Some Concepts Related to Cookies
Before Setting Cookies in FastAPI, let us discuss and get familiar with some of the terminology and concepts:
Response: Response is a class present in the FastAPI module which is used to customize the HTTP response that is sent to the client by the server. It consists of various attributes such as status_code that can be used to set the status of the HTTP response as well as methods send_json for sending JSON data to the client.
set_cookie: set_cookie is a method present in the Response class that can be used to set the cookie on the user’s browser. It takes a key and value. The key is used to identify the cookie and the value is the information that is used by the server.
response.set_cookie(key="cookie_key", value="cookie_value")
Response Parameter: You can define a parameter of type Response in the decorator function when declaring an endpoint. The response object can then be used to set the cookie. The fastAPI will use this temporary response object to set the various HTTP response information setter by using its methods and attributes and then the HTTP response is send to the client.
def func(response: Response)
Setting Cookies in FastAPI
Create a folder named ‘gfg_fastapi’ and create a file named app.py and copy paste the below code:
In the below code we first created the app using FastAPI class and the defined the “/” endpoint which will have a response parameter. The response instance is used to set the cookie using the ‘set_cookie’ method present in it.
Python3
from fastapi import FastAPI, Response, Cookie
from typing import Annotated
app = FastAPI()
@app .get( "/" )
def func(response : Response):
response.set_cookie(key = "gfg_cookie_key" , value = "gfg_cookie_value" )
return { "message" : "Cookie is set on the browser" }
|
Running the Application
To run the above application copy paste the below command and run in terminal
python -m uvicorn app:app --reload
Output
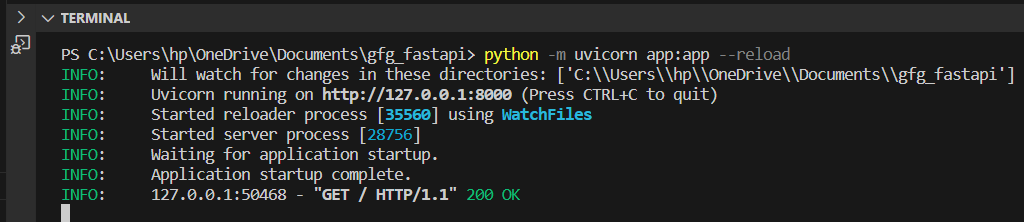
The server will be up and running and will be available at the below URL
http://127.0.0.1:8000
When you open the above url on web browser of your choice, the “/” endpoint defined in the application is hit and the func function is executed which set the cookie on the browser.
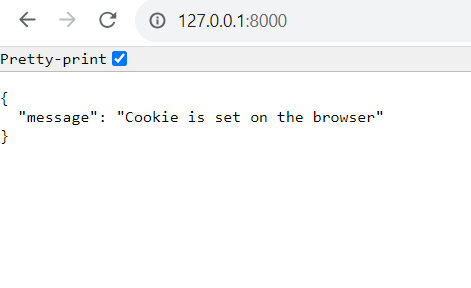
Application “/” endpoint
You can see the cookie being set in the browser using the developer tool.
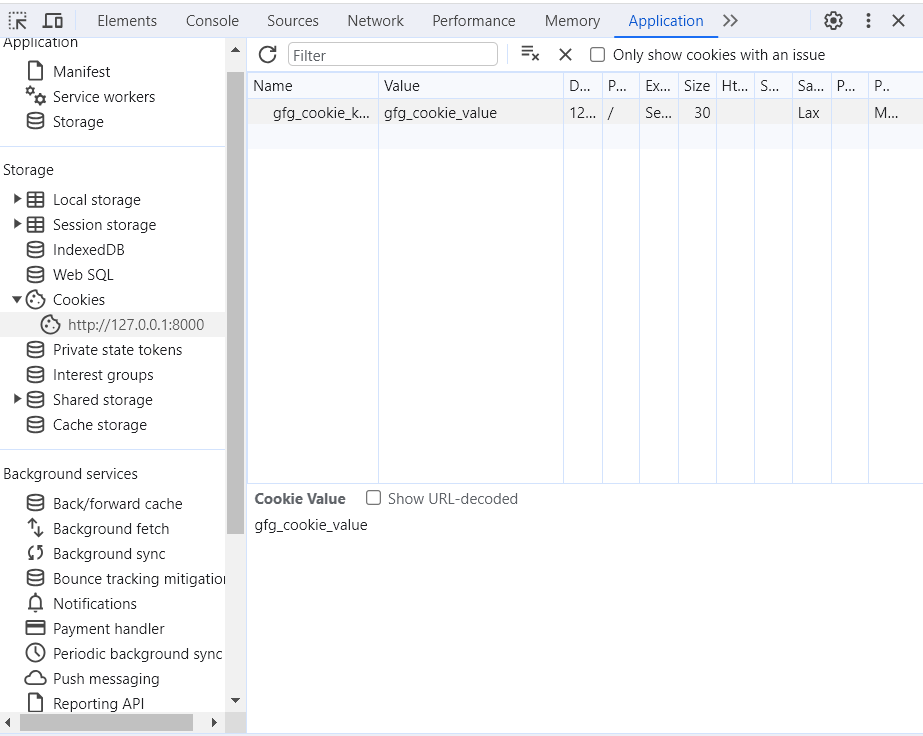
Cookies
Getting Cookies in FastAPI
Cookie parameter is used to get the cookie sent by the client to the server in the HTTP request. To use the cookie parameter in the decorator function you first need to import Cookie
from fastapi import Cookie
Now you can use it to get the cookie sent by the client in the decorator function as shown below :
@app.get("/cookie")
def func(get_cookie_key : Annotated[str | None, Cookie()] = None):
When the user sends an HTTP request to the client at the “/cookie” endpoint the server first parse the cookie “get_cookie_key” and send it as a parameter to the decorator function func. Copy the below code and paste it in app.py file created above :
Python3
@app .get( "/cookie" )
def func(gfg_cookie_key : Annotated[ str | None , Cookie()] = None ):
return { "gfg_cookie" : gfg_cookie_key}
|
Copy the below URL and paste it in browser
http://127.0.0.1:8000/cookie
When you hit the above url the browser sends the HTTP request to the server along the Cookies that is set by the server previously. When the request hits the server the server then parse the cookies sent by the browser and get the value of the ‘gfg_cookie_key’ and pass it to the decorator function defined for the “/cookie” endpoint. The server then just returns the cookie value as the response.
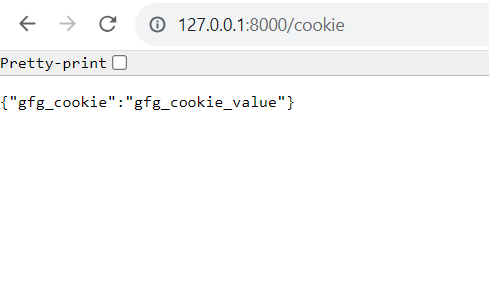
“/cookie” endpoint
Share your thoughts in the comments
Please Login to comment...