Return an Image in FastAPI
Last Updated :
16 Oct, 2023
FastAPI is a Python web framework that makes it easy to build APIs quickly and efficiently. Returning an image is a common requirement in web development, and FastAPI makes it straightforward to do. another famous framework for doing the same is Flask, which is also Python-based. In this article, we will focus on creating API for images using FastAPI.
Return an Image in FastAPI
Installation of the necessary Library
For creating API using FastAPI we need fastAPI, also for running the server we also need a uvicorn. so before starting our API coding part, we need to the installation of fastAPI and uvicorn. We can install them by running the below command:
pip install fastapi
pip install uvicorn
To return an image in FastAPI, we will first need to have an image file that we want to serve. Let’s assume we have an image file named “gfglogo.jpg” that we want to return as a response to the client who is requesting the API. Now let’s start creating API using FastAPI
Importing FastAPI and Additional Libraries
First of all create one folder with the name you want, eg GFGAPI, inside that folder create a file with the name ‘main.py‘. add the image you want to return as a response in that folder eg, “gfglogo.jpg“. The code in main.py file is the to import FastAPI and Additional Libraries which we need to create API as required.
Python3
from fastapi import FastAPI
from fastapi.responses import FileResponse
from pathlib import Path
|
Creating a FastAPI App
Now to use fastAPI we need to create it’s app, it’s very simpe as we just need to call FastAPI().
Defining a Route to Serve the Image
We define a route ‘/get_image‘ using the @app.get decorator. This route will handle GET requests. In this step we need to make route to our app, as when any API request will come to our app first of all it will try to match with the url we mentioned inside app.get(), here get because client want the image in response so, client will send the get request.
We define the path to the image file. You should replace “gfglogo.jpg“ with the actual path to your image file, if your file is inside the folder where the main.py file then simply write “filename.jpg”.
We then check if the file exists using image_path.is_file(). If the image file is not found, we return an error message, otherwise we return the image as a FileResponse. FastAPI will automatically handle the response headers and content type for us, making it easy to serve images
Python3
@app .get( "/get_image" )
async def get_image():
image_path = Path( "gfglogo.jpg" )
if not image_path.is_file():
return { "error" : "Image not found on the server" }
return FileResponse(image_path)
|
Code Implemenataion
In this example, we’ve created a FastAPI app and defined a route at “/get_image” that returns the “gfglogo.jpg“ file as a response using FileResponse. If the file doesn’t exist, it returns an error message.
Here you found that async keyword is used because reading a file (in this case, an image file) is typically an I/O-bound operation that can benefit from asynchronous handling. By marking the route as async, FastAPI can take advantage of asynchronous I/O operations, making the server more efficient and responsive when serving the file such as image file.
Python3
from fastapi import FastAPI
from fastapi.responses import FileResponse
from pathlib import Path
app = FastAPI()
@app .get( "/get_image" )
async def get_image():
image_path = Path( "gfglogo.jpg" )
if not image_path.is_file():
return { "error" : "Image not found on the server" }
return FileResponse(image_path)
|
Deployement of the Project
You can run the FastAPI app using a web server such as uvicorn:
uvicorn your_app_name:app --reload
Replace ‘your_app_name’ with the name of the file we given, here we had given file name as ‘main.py’ so use main.
uvicorn main:app --reload
Now, when you access ‘http://localhost:8000/get_image‘ or ‘http://127.0.0.1:8000/get_image‘ in your web browser or make a GET request to that URL using a tool like postman, the FastAPI app will return the image as a response.
Output
.jpg)
VS code editor terminal code & output
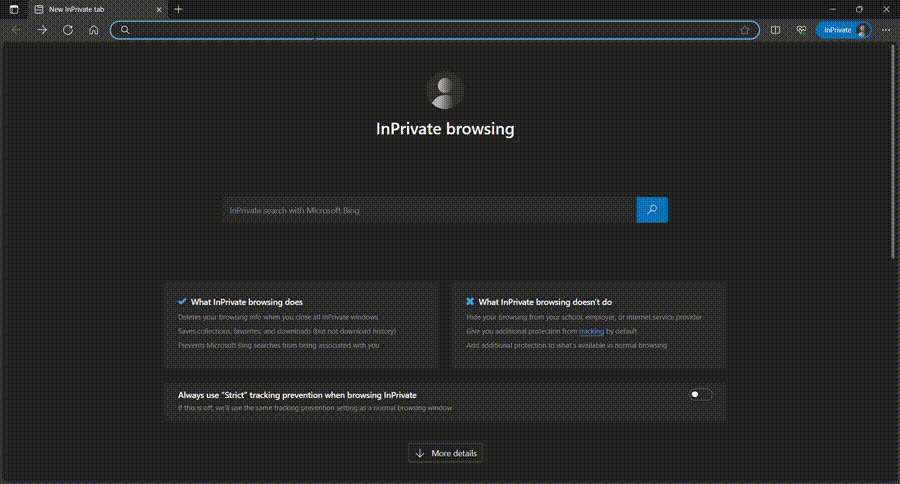
output of API in browser
You can also test this api by Postman by just sending GET request on “http://127.0.0.1:8000/get_image“. This is all about how we can return an image using FatsAPI. By following these steps, you can create a FastAPI route that serves images to clients. This is a simplified example to do this.
Share your thoughts in the comments
Please Login to comment...