FastAPI – Query Parameters
Last Updated :
28 Dec, 2023
In this article, we will learn about FastAPI Query Parameters, what are query parameters, what they do in FastAPI, and how to use them. Additionally, we will see examples of query parameters for best practices.
What is FastAPI – Query Parameters?
Query parameters stand as a formidable asset in bolstering the flexibility and usability of an API. Their utility lies in the ability to filter, sort, paginate, and search data seamlessly, all without the need to create dedicated endpoints for each specific task.
In the FastAPI framework, these optional parameters, aptly named query parameters, can be transmitted to the API endpoint directly through the URL. The mechanism involves including individual parameters after a question mark (?), with each parameter being demarcated by an ampersand (&). This approach not only streamlines the process of interacting with the API but also empowers developers to dynamically tailor their queries, making the API more versatile and user-friendly. There are two main types of query parameters in FastAPI:
- Path parameters: Path parameters are defined in the path to the endpoint, preceded by a question mark (?). They are usually set within curly braces ({}). Path parameters are required to successfully call the endpoint and must be provided in the URL.
- Query string parameters: The query string parameter is defined after the question mark (?) in the endpoint’s query string. They are optional and can be provided if desired.
Why Do We Need Query Parameters?
- Flexibility: The endpoint’s results can be customized to the client’s specific requirements thanks to query parameters. For example, a consumer can restrict product searches by category, price, or rating by using query parameters.
- Usability: Both clients and developers can simply utilize query parameters. Customers may add query parameters to the endpoint’s URL with ease, and developers can verify and validate query parameters within their code very quickly.
- Performance: The number of endpoints that need to be created and updated can be reduced and API speed can be improved with the help of query parameters. For example, instead of setting up separate endpoints for each product category, use a single endpoint with the category query parameter.
Here’s how query parameters can be used in FastAPI:
FastAPI – Query Parameters Examples
Here, we will explore commonly used examples of FastAPI to illustrate its functionality. The examples are outlined below.
FastAPI Greeting API
In this example , code defines a FastAPI application with a single endpoint `/hello` that accepts an optional query parameter `name` and returns a greeting message. The `hello` function handles the logic, generating a personalized greeting if a name is provided, otherwise a default welcome message. If the name argument is given the endpoint will return a greeting message with the user’s name. Otherwise, the endpoint will return a generic default message.
Python3
from fastapi import FastAPI
app = FastAPI()
@app .get( "/hello" )
async def hello(name: str = None ):
if name is None :
return "Hello, Welcome to GeeksForGeeks!"
else :
return f "Hello, {name} Welcome to GeeksForGeeks!"
|
Here’s how to call the endpoint:
Endpoint Example 1:
http://localhost:8000/hello
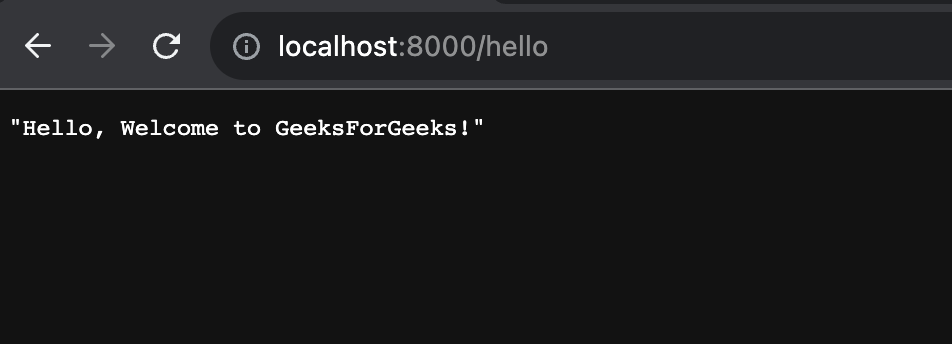
Endpoint Example 2:
http://localhost:8000/hello?name=Alice
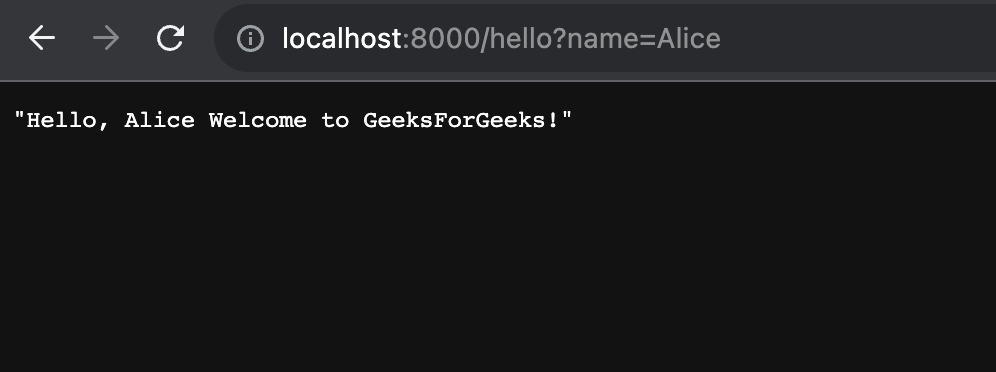
FastAPI Product Search Endpoint
In this example FastAPI code defines a simple web application with a single endpoint /products
. The endpoint supports a query parameter search_query
for filtering products. The function get_products
retrieves a list of products and filters them based on the provided search query, returning the result as a JSON response
Python3
from fastapi import FastAPI
app = FastAPI()
@app .get( "/products" )
async def get_products(search_query: str = None ):
products = [ "shoes" , "electronics" , "clothing" , "running shoes" , "mobile phones" , "sports shoes" ]
if search_query:
products = [product for product in products if search_query in product]
return { "products" : products}
|
This will return a list of all products, whether they contain the search term or not.
http://localhost:8000/products
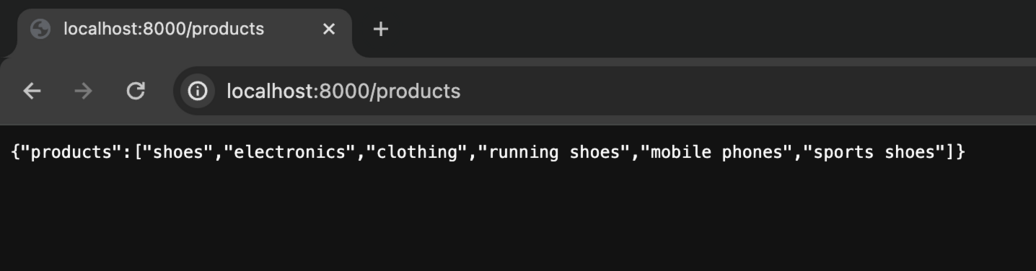
Use the search_query parameter to filter results by endpoint. For example, use the following URL to filter results to include only products that contain the search term “electronics”:
http://localhost:8000/products?search_query=electronics
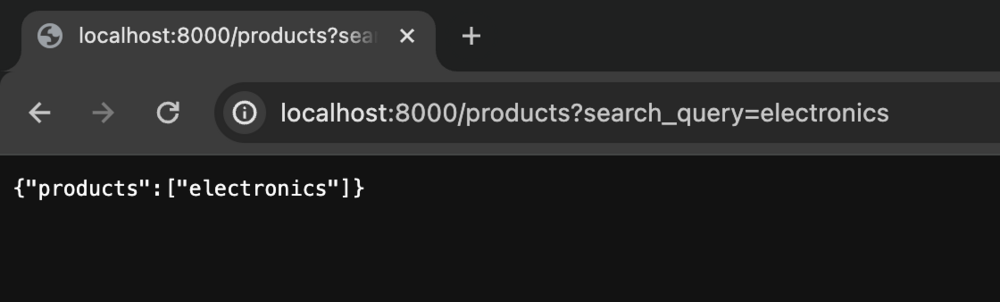
Best Practices For Using Query Parameters in FastAPI
When working with query parameters in FastAPI, adopting best practices is essential to ensure the clarity, reliability, and security of your API. Here are some key guidelines to enhance your use of query parameters:
- Choose Meaningful Parameter Names: Ensure that your query parameters have names that are not only relevant but also convey their purpose clearly. Opt for names that enhance the readability and understandability of the API. For instance, use “search_query” instead of a generic “q” parameter.
- Define Parameter Types: Specify the type of each query parameter to streamline the API and reduce the likelihood of errors. Clearly define whether a parameter is intended to be a string, integer, or another data type. For example, explicitly set the type of the “search_query” option as str if it is meant to represent a string.
- Set Default Values for Optional Parameters: When dealing with optional query parameters, assign default values. This practice minimizes errors and enhances the overall usability of the API. For instance, give the “search_query” argument a default value of “” to handle cases where users might not provide a specific search term.
- Validate Query Parameters: Implement validation mechanisms for query parameters to prevent unauthorized users from misusing the API. For instance, ensure that the length of the “search_query” argument is within acceptable limits to avoid potential security vulnerabilities.
- Enable Sorting and Filtering: Leverage query parameters to empower users to sort and filter data, providing them with the ability to customize API results. For example, enable product searches to filter results based on the “search_query” option, offering a tailored experience to users.
Conclusion
In conclusion, FastAPI’s support for query parameters enhances the development of robust and flexible APIs. The ability to seamlessly integrate parameters into API routes provides a convenient way to handle client inputs. Whether filtering data, setting defaults, or handling optional parameters, FastAPI’s query parameters simplify the development process. Leveraging automatic validation, documentation generation, and support for complex types, FastAPI empowers developers to create efficient and self-documenting APIs with ease. The versatility and performance of FastAPI, coupled with its intuitive handling of query parameters, make it a powerful framework for building modern web APIs.
Share your thoughts in the comments
Please Login to comment...