Save UploadFile in FastAPI
Last Updated :
27 Oct, 2023
FastAPI is an easy, fast, and robust web framework for creating API’s with Python 3.7+. It was created by Sebastian Ramirez. It is built on top of Starlette and Pydantic, both of which are asynchronous libraries i.e. they use async and await to serve the requests efficiently. FastAPI makes routing, session management, authentication, and file uploads very easy.
What is UploadFile in FastAPI?
UploadFile is a class provided by the FastAPI for handling files. You can define UploadFile as an argument to a function for an HTTP endpoint. So when a user sends an HTTP request containing a file, all information regarding that file will be passed to the function using the UploadFile instance. UploadFile class has the following attributes for getting information about the file submitted by the user:
- filename: It is of type string that stores the name of the file uploaded by the user.
- content_type: It stores information about the content type in the uploaded file. It is also of type string.
- file: The file object which stores the content of the file uploaded by the user.
- write(data): write the data to the file
- read(size) : read size number of bytes from the file
- close() : Close the file
We are going to define the “/upload/” endpoint which will allow a user to send a file. For that, we are going to create an HTML form asking for a text, PDF, or JPG file. This form will be submitted at the “/upload/” endpoint. For that endpoint, we are going to define the decorator function which will take the UploadFile class instance containing all information regarding the uploaded file.
Setting up the Project
You should have Python version 3.7 or higher installed on your machine as well as the fast API and uvicorn package.
To install FastAPI using pip run the below command in the terminal :
pip install fastapi
Uvicorn is an ASGI(Asynchronous Server Gateway Interface) server which is needed to run the code developed using fastAPI. For installing uvicorn run the below command in the terminal :
pip install "uvicorn[standard]"
The directory structure should look like this
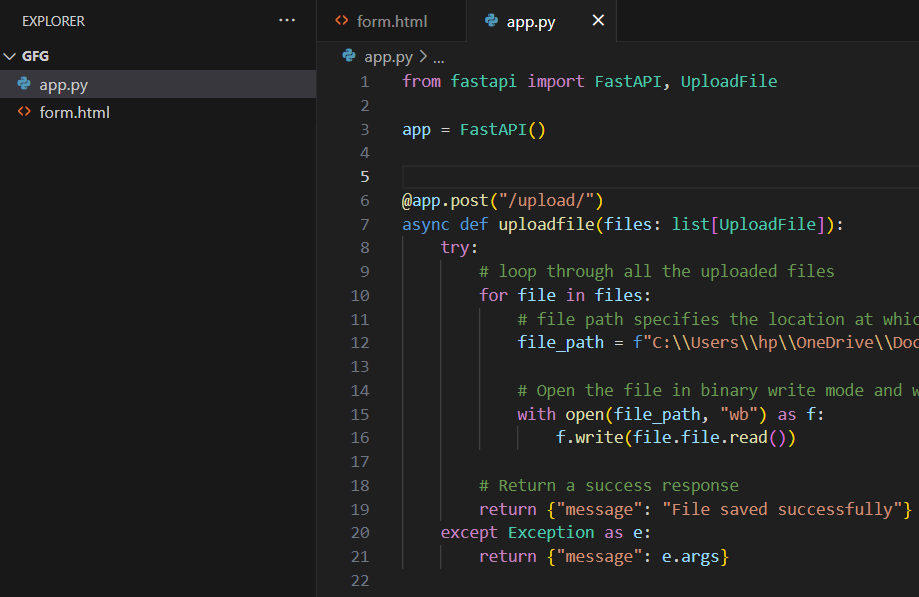
Uploading Single Files in FastAPI
form.html: Below is a simple HTML code snippet for taking a file as input and submit it to the localhost /upload endpoint. Create a folder named gfg and create a file named form.html in it. Copy the below code and paste it in the form.html file
HTML
<!DOCTYPE html>
< html >
< head >
< title >GFG</ title >
</ head >
< body >
< h2 >Geeks for Geeks </ h2 >
< label for = "file" >Choosen file will be saved on server :</ label >
< input type = "file" id = "file" name = "file" accept = ".txt, .pdf, .jpg" >
< br >< br >
< input type = "submit" value = "Upload" >
</ form >
</ body >
</ html >
|
When you submit html form data which does not include any file, the data is encoded as application/x-www-form-urlencoded, and when it include files it is encoded as multipart/form-data . To be able to take the html form file input we first need to install python-mutipart package using pip. Run the below command in the terminal :
pip install python-multipart
app.py: We first imported the fastAPI and UploadFile class from the fastapi package.Then we created an instance of the fastAPI class which will be used to define the various endpoints of the application.Then we define the path operation decorator in which we specified the type of http request as well as the http endpoint.Then we declared the function which is going to be executed when the upload endpoint is called. The function is asynchronous and takes in an UploadFile instance.
Python3
from fastapi import FastAPI, UploadFile
app = FastAPI()
@app .post( "/upload/" )
async def uploadfile( file : UploadFile):
try :
file_path = f "C:\\Users\\hp\\OneDrive\\Documents\\gfg/{file.filename}"
with open (file_path, "wb" ) as f:
f.write( file . file .read())
return { "message" : "File saved successfully" }
except Exception as e:
return { "message" : e.args}
|
Now to be able to access the /upload endpoint we need to use uvicorn. Copy paste the below commmand in the terminal :
python -m uvicorn app::app –reload
In the above line we specified the file name using app, and the fastAPI instance we created in the file with the name app. The reload in the end is used to automatically restart the server in case the app.py file is changed. The above command will start the server . The above command will start the server on local machine on port 8000 which will be accessible at the below url :
http://127.0.0.1:8000/
Now open the form.html file in the browser and select a file to save and submit it. The file will be stored on the server at the path specified in the code.
Uploading Multiple Files in FastAPI
When you create an html form which can take multiple file inputs in that case all the files will be send to the server and the “/upload” endpoint will no longer be valid because the decorator function for that endpoint only takes single UploadFile, for the above endpoint to work with multiple files we need to pass list[UploadFile] as an argument to the “/upload” endpoint decorator function. So all the files uploaded to the /upload endpoint will be stored in the list.
Change the Above HTML code to take multiple file inputs:
HTML
<!DOCTYPE html>
< html >
< head >
< title >GFG</ title >
</ head >
< body >
< h2 >Geeks for Geeks </ h2 >
< label for = "file" >Choosen file will be saved on server :</ label >
< input type = "file" name = "files" accept = ".jpg" multiple>
< br >< br >
< input type = "submit" value = "Upload" >
</ form >
</ body >
</ html >
|
app.py: In the above code we specified the data type in the uploadfile function to be a list[UploadFile]. Then we loop through each of the file submitted by the user and saved it on the server.
Python3
from fastapi import FastAPI, UploadFile
app = FastAPI()
@app .post( "/upload/" )
async def uploadfile(files: list [UploadFile]):
try :
for file in files:
file_path = f "C:\\Users\\hp\\OneDrive\\Documents\\gfg/{file.filename}"
with open (file_path, "wb" ) as f:
f.write( file . file .read())
return { "message" : "File saved successfully" }
except Exception as e:
return { "message" : e.args}
|
Output:
Now to be able to access the /upload endpoint we need to use uvicorn. Copy paste the below commmand in the terminal :
python -m uvicorn app::app –reload
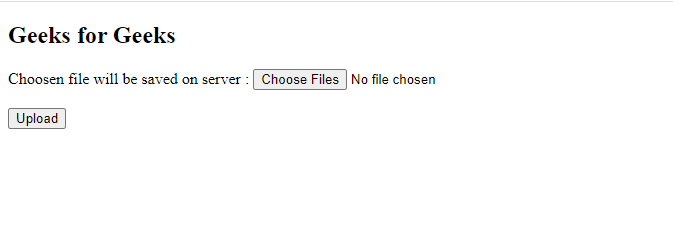
Form.html
In the above image. You have to Choose the Files and then Click on the Upload Button. It Directly Redirect to the /Upload Url and return a dictionary with {“message”:”File saved successfully”}. After that You can check. the file has successfully saved on your localhost server.
Share your thoughts in the comments
Please Login to comment...