A fast and modern Python web framework for building API is known as FastAPI. Like various instances in Python, FastAPI could also give some errors on performing a certain action, for which the user needs to be notified. In this article, we will discuss various ways to handle errors in FastAPI.
What is Error Handling in FastAPI?
Error handling is a crucial aspect of web development when building robust and reliable applications. In the context of FastAPI, a modern web framework for building APIs with Python, understanding and implementing error handling is essential to ensure your API responds gracefully to various situations.
Ways to Handle Errors in FastAPI
- Using HTTP status codes
- Using built-in exception handlers
- Using custom exception handlers
- Using middleware
- Using logging
Error Handling using HTTP Status Codes
The FastAPI provides you with some HTTP status codes that give users information on how the server handled a client request. In this method, we will see how we can handle errors in FastAPI using HTTP status codes. The HTTP status codes are basically grouped into five classes:
Informational responses
|
100-199
|
Successful responses
|
200-299
|
Redirection messages
|
300-399
|
Client error responses
|
400-499
|
Server error responses
|
500-599
|
Syntax: def function_name() -> Item:
if condition:
raise HTTPException(status_code=HTTP_Code_1, detail=f”error_message_1″)
else:
raise HTTPException(status_code=HTTP_Code_2, detail=f”error_message_2″)
Here,
- function_name: It is the function which you want to call while testing.
- condition: It is the check which you want to add to return the error.
- error_message_1, error_message_2: These are the messages shown to user when there is an error.
- HTTP_Code_1, HTTP_Code_2: These are the HTTP status codes which we want to display to user along with error message.
Example: In this example, we are handling errors through two status codes, i.e., 200 for success, and 404 for error. The code 200 with message is displayed if roll number exist in students list, while the code 404 with message is displayed if roll number does not exist in students list.
Python3
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
app = FastAPI()
class Student(BaseModel):
name: str
roll_number: int
students = {
1 : Student(name = "Abhishek" , roll_number = 1 ),
2 : Student(name = "Ishita" , roll_number = 2 ),
3 : Student(name = "Vinayak" , roll_number = 3 ),
}
@app .get( "/items/{roll_number}" )
def query_student_by_roll_number(roll_number: int ) - > Student:
if roll_number not in students:
raise HTTPException(status_code = 404 , detail = f "Student with {roll_number=} does not exist." )
else :
raise HTTPException(status_code = 200 , detail = f "Student details are as follows: {students[roll_number]}" )
|
Output: On calling the roll number 2 which exists in the student list, it displays an error message as follows:
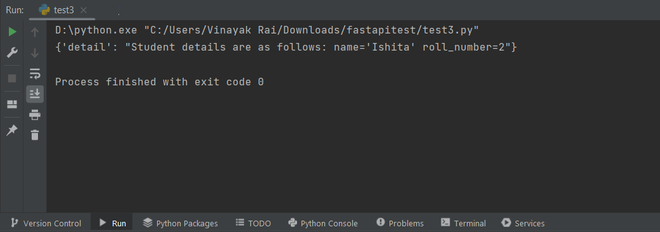
On calling the roll number 4 which doesn’t exist in the student list, it displays an error message as follows:
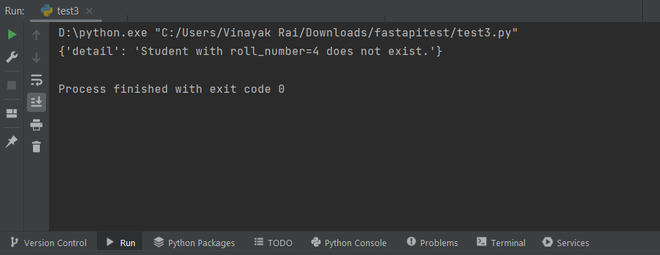
Built-In Exception Handlers in FastAPI
The built-in exception handler in FastAPI is using HTTP exception, which is normal Python exception with some additional data. In this method, we will see how we can handle error in FastAPI using built-in exception handlers.
Syntax: def function_name() -> Item:
if condition:
raise HTTPException(status_code=HTTP_Code, detail=f”error_message”)
Here,
- function_name: It is the function which you want to call while testing.
- condition: It is the check which you want to add to return the error.
- error_message: It is the message shown to user when there is an error.
- HTTP_Code: It is the HTTP status code which we want to display to user along with error message.
Example: In this example, we are checking if the roll number of a student exists in the list of students, then that student value is returned else an error message is displayed.
Python3
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
app = FastAPI()
class Student(BaseModel):
name: str
roll_number: int
students = {
1 : Student(name = "Abhishek" , roll_number = 1 ),
2 : Student(name = "Ishita" , roll_number = 2 ),
3 : Student(name = "Vinayak" , roll_number = 3 ),
}
@app .get( "/items/{roll_number}" )
def query_student_by_roll_number(roll_number: int ) - > Student:
if roll_number not in students:
raise HTTPException(status_code = 404 , detail = f "Student with {roll_number=} does not exist." )
return students[roll_number]
|
Output: On calling the roll number 4 which doesn’t exist in the student list, it displays an error message as follows:
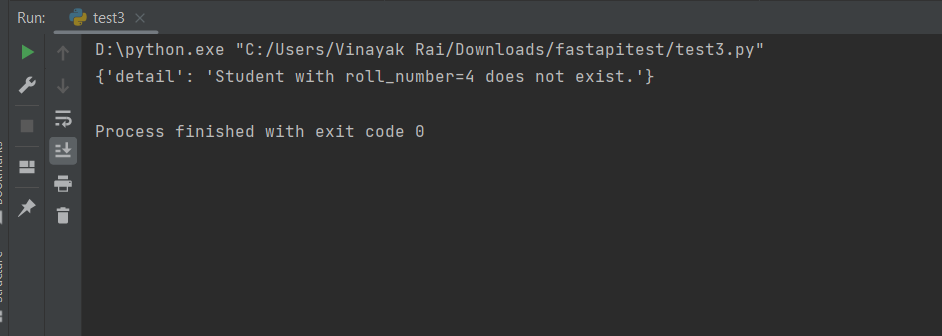
Custom Exception Handlers in FastAPI
One of the crucial library in Python which handles exceptions in Starlette. It is also used to raise the custom exceptions in FastAPI. In this method, we will see how we can handle errors using custom exception handlers.
Syntax:
class UnicornException(Exception):
def __init__(self, value: str):
self.value = value
#Create a custom exception
@app.exception_handler(UnicornException)
async def unicorn_exception_handler(request: Request, exc: UnicornException):
return JSONResponse(status_code=404, content={“message”: f”error_message”}, )
async def function_name(roll_number: int):
if condition:
raise UnicornException(value=condition_value)
Here,
- function_name: It is the function which you want to call while testing.
- condition: It is the check which you want to add to return the error.
- error_message: It is the message shown to user when there is an error.
- condition_value: It is the value on which condition needs to be checked.
Example: In this example, we are checking if the roll number of a student exists in the list of students, then that student value is returned else an error message is displayed.
Python3
from fastapi import FastAPI, Request
from pydantic import BaseModel
from fastapi.responses import JSONResponse
app = FastAPI()
class UnicornException(Exception):
def __init__( self , value: str ):
self .value = value
class Student(BaseModel):
name: str
roll_number: int
students = {
1 : Student(name = "Abhishek" , roll_number = 1 ),
2 : Student(name = "Ishita" , roll_number = 2 ),
3 : Student(name = "Vinayak" , roll_number = 3 ),
}
@app .exception_handler(UnicornException)
async def unicorn_exception_handler(request: Request, exc: UnicornException):
return JSONResponse(
status_code = 404 ,
content = { "message" : f "Student with particular roll number does not exist." },
)
@app .get( "/items/{roll_number}" )
async def read_unicorn(roll_number: int ):
if roll_number not in students:
raise UnicornException(value = roll_number)
return students[roll_number]
|
Output:
On calling the roll number 4 which doesn’t exist in the student list, it displays an error message as follows:
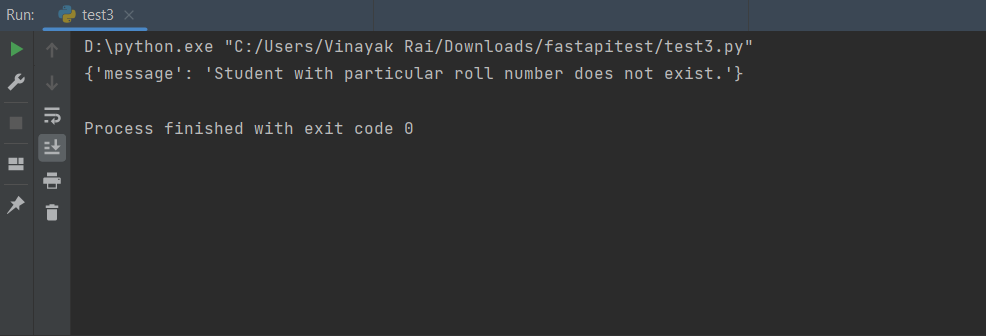
Middleware in FastAPI
A function in FastAPI that works with each request before it is processed is known as middleware. In this method, we will see how we can handle errors in FastAPI using middleware.
Syntax: app.add_middleware(GZipMiddleware)
async def function_name():
if condition:
return (f”error_message”)
Here,
- function_name: It is the function which you want to call while testing.
- condition: It is the check which you want to add to return the error.
- error_message: It is the message shown to user when there is an error.
Example: In this example, we are checking if the roll number of a student exists in the list of students, then that student value is returned else an error message is displayed.
Python3
from fastapi import FastAPI, Request
from pydantic import BaseModel
from fastapi.middleware.gzip import GZipMiddleware
app = FastAPI()
app.add_middleware(GZipMiddleware)
class Student(BaseModel):
name: str
roll_number: int
students = {
1 : Student(name = "Abhishek" , roll_number = 1 ),
2 : Student(name = "Ishita" , roll_number = 2 ),
3 : Student(name = "Vinayak" , roll_number = 3 ),
}
@app .get( "/items/{roll_number}" )
async def query_student_by_roll_number(roll_number: int ):
if roll_number not in students:
return (f "Student with {roll_number=} does not exist." )
return students[roll_number]
|
Output:
On calling the roll number 4 which doesn’t exist in the student list, it displays an error message as follows:
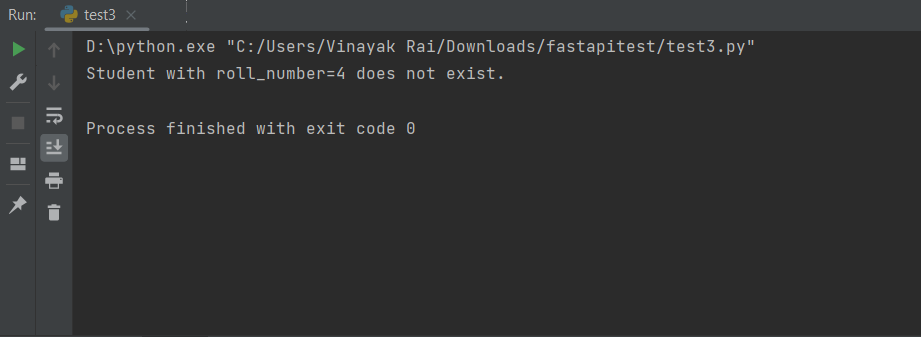
Logging in FastAPI
The crucial set of functions, such as debug, catch are provided by the logging in Python. In this method, we will see how we can handle errors in FastAPI using logging.
Syntax: logger = logging.getLogger(__name__)
async def function_name():
try:
if condition:
return (f”error_message”)
return students[roll_number]
except Exception as e:
logger.exception(e)
raise HTTPException(status_code=500, detail=”Internal server error”)
Here,
- function_name: It is the function which you want to call while testing.
- condition: It is the check which you want to add to return the error.
- error_message: It is the message shown to user when there is an error.
Example: In this example, we are checking if the roll number of a student exists in the list of students, then that student value is returned else an error message is displayed.
Python3
from fastapi import FastAPI, Request, HTTPException
from pydantic import BaseModel
import logging
app = FastAPI()
logger = logging.getLogger(__name__)
class Student(BaseModel):
name: str
roll_number: int
students = {
1 : Student(name = "Abhishek" , roll_number = 1 ),
2 : Student(name = "Ishita" , roll_number = 2 ),
3 : Student(name = "Vinayak" , roll_number = 3 ),
}
@app .get( "/items/{roll_number}" )
async def query_student_by_roll_number(roll_number: int ):
try :
if roll_number not in students:
return (f "Student with {roll_number=} does not exist." )
return students[roll_number]
except Exception as e:
logger.exception(e)
raise HTTPException(status_code = 500 , detail = "Internal server error" )
|
Output:
On calling the roll number 4 which doesn’t exist in the student list, it displays an error message as follows:
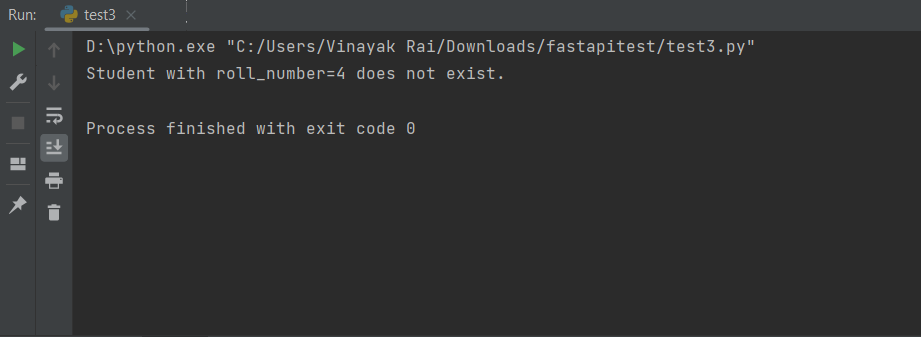
Conclusion:
It is not in our hands to stop the user from doing a certain action which can raise an error, but what is in our hands is to handle those errors so that user can get to know that they have done something wrong. The numerous methods defined in this article will help you to handle certain errors in FastAPI.
Share your thoughts in the comments
Please Login to comment...