Difference between res.send() and res.json() in Express.js?
Last Updated :
13 Nov, 2023
In this article, we will learn about res.send() & res.json(), along with discussing the significant distinction that differentiates between res.send() & res.json().
Let us first understand what is res.send() and res.json() in Express.js?
res.send() – The res.send() function is used for sending the response for the HTTP request. It takes a parameter body. The parameter can be a String, Buffer object, an object, Boolean, or an Array.
Syntax:
res.send( [body] )
Parameter: The body parameter takes a single argument that describes the body that is to be sent in the response.
Content Type: The Express sets the content type of the parameter according to the type of the body parameter.
- String & HTML – “text/html”
- Buffer – “application/octet-stream”
- Array & object – “application/json”
Return Type: It is used to send a response to the client and then ends the response process. The method itself doesn’t return anything.
Example: This example demonstrate the simple res.send().
Javascript
const express = require( 'express' );
const app = express();
const PORT = 8000;
app.get( '/' , (req, res)=>{
res.send( 'Hello World' )
});
app.listen(PORT, ()=>{
console.log(`Server is listening to port: ${PORT}`)
});
|
Output:
Server is listening to port: 8000
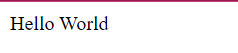
Simple example of res.send()
res.json() – The res.json() function sends the response as JSON format. It automatically converts the JavaScript object into a JSON-formatted string. The parameter body can be of any JSON type, which includes object, array, string, Boolean, number, or null.
res.json() will also convert non-objects, such as null and undefined, which are not valid JSON.
Syntax:
res.json( [body] )
Parameter: The body parameter takes JavaScript Object as its argument.
Content Type: Its content-type is set to – “application/json”.
Returns: It is used to send JSON response to the client and then ends the response process.
Example: This example demonstrate the simple res.send().
Javascript
const express = require( 'express' );
const app = express();
const PORT = 8000;
const data = [
{id: 1, name: 'GFG' },
{id: 2, name: 'Express' }
]
app.get( '/' , (req, res)=>{
res.json(data)
});
app.listen(PORT, ()=>{
console.log(`Server is listening to port: ${PORT}`)
});
|
Output:
Server is listening to port: 8000
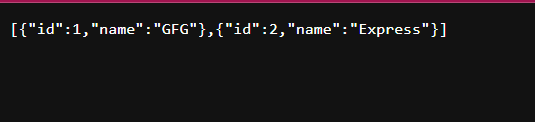
Simple example of res.json()
Difference between res.send() and res.json():
Through both res.send() and res.json() seems to identical and fulfill similar purposes but still there is significant contrast that differentiates both res.send() and res.json(), which is given below.
The Content-Type header is automatically set based on the type of body parameter being sent.
|
The Content-Type header is explicitly set to ‘application/json’, indicating that the response contains JSON data.
|
This method is used as general-purpose and can be used to handle various types of data and the content type will also set automatically according to the data.
|
This method is specially designed to send JSON response means it will convert all type of body params into JSON object.
|
This method doesn’t perform any automatic JSON stringification.
|
This method automatically converts the JavaScript Object passed into it to JSON-formatted string.
|
It doesn’t convert or format the data given to them.
|
You can format the returned JSON data by using json replacer, json spaces etc.
|
More versatile and can be used when you want to send different types of data without explicitly indicating that it’s JSON.
|
Specifically used when you want to send JSON-formatted data and want to be explicit about it.
|
Conclusion:
Both res.send() and res.json() serves similar purposes with some difference. So it depends on the data type which we are working with. Choose res.json() when you are specifically working with JSON data. Use res.send() when you need versatility and control over the content type or when dealing with various data types in your responses.
Share your thoughts in the comments
Please Login to comment...