Explain the componentDidCatch Lifecycle method in the Context of Error Handling ?
Last Updated :
09 Apr, 2024
The componentDidCatch is a lifecycle method in React that helps in handling errors that occur during the rendering of a component’s tree. It allows components to catch errors generated by their children components, log those errors, and display a fallback UI instead of crashing the entire application.
Prerequisites
How does the componentDidCatch method work?
The componentDidCatch lifecycle method allows a React component to catch and handle errors that occur while rendering its child components. Here’s how it works:
- Error Boundary Component: To use componentDidCatch, you need to create an error boundary component. An error boundary component is a regular React component that implements the componentDidCatch method.
- Placement: You place the error boundary component around the part of your application where you want to catch errors. This can be around a specific component subtree or at the top level of your application to catch errors globally.
- Error Handling: When an error occurs during the rendering of any component within the error boundary, React will call the componentDidCatch method of the nearest error boundary component in the component tree.
- Error Logging: Inside the componentDidCatch method, you can log the error and any additional information about the error, such as the component stack trace.
- Fallback UI: After catching the error, the error boundary component has the opportunity to render a fallback UI instead of the component tree that caused the error. This helps prevent the entire application from crashing and provides a better user experience.
- Continued Rendering: Despite the error occurring within the error boundary, the rest of the application continues to function normally. Only the component subtree within the error boundary is affected, allowing the user to interact with other parts of the application.
So componentDidCatch provides a way to gracefully handle errors in React applications by isolating them to specific parts of the UI and preventing them from breaking the entire application. It promotes better error management and improves the reliability of React applications.
How to use componentDidCatch in React Applications?
Step 1: Create a new React application using Create React App.
npx create-react-app error-handling-app
Step 2: Navigate to the project directory.
cd error-handling-app
Approach:
- Define a component that extends React’s Component class and implements componentDidCatch to handle errors within its child components.
- Initialize a state variable in the Error Boundary Component to track whether an error has occurred (
hasError: false
). - Inside componentDidCatch, update the state to indicate that an error has occurred (
this.setState({ hasError: true })
) and handle the error (e.g., log it to the console or send it to an error tracking service). - In the render method of the Error Boundary Component, check if an error has occurred (
this.state.hasError
) and render a fallback UI (e.g., an error message) instead of the child components if needed. - Surround components or parts of your application where you want to catch errors with the Error Boundary Component. This ensures that any errors occurring within that boundary are caught and handled gracefully, preventing the entire app from crashing.
Example: Implementation to explain the use of componentDidCatch lifecycle method in the context of error handling.
Javascript
import { useEffect, useState } from "react";
export default function FetchPract() {
const [data, setData] = useState();
const [loading, setLoading] = useState(true);
useEffect(() => {
async function fetchData() {
try {
const response = await fetch(
'https://api.github.com/repos/tannerlinsley/react-query');
const jsonData = await response.json();
setData(jsonData);
} catch (error) {
console.log(error);
} finally {
setLoading(false);
}
}
fetchData();
}, []);
if (loading) {
return <div>Loading...</div>;
}
return (
<div>
<h1>{data.name}</h1>
<p>{data.description}</p>
<strong>👀 {data.subscribers_count}</strong>{' '}
<strong>✨ {data.stargazers_count}</strong>{' '}
<strong>🍴 {data.forks_count}</strong>
</div>
);
}
Step 4: Start the development server.
npm start
Output:
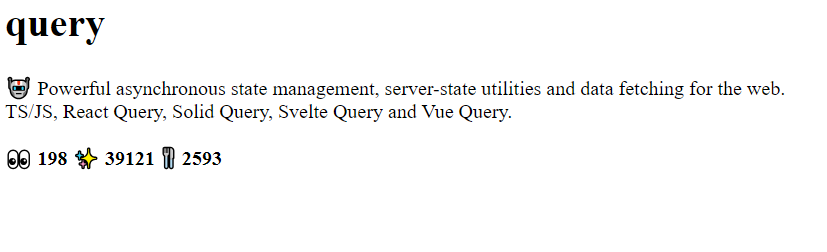
When componentDidCatch is useful?
componentDidCatch is useful in various situations where you want to gracefully handle errors that occur during the rendering of a React component tree. Some common scenarios where componentDidCatch is beneficial include:
- Third-party Integrations: Handles errors from third-party libraries or components to prevent application crashes.
- Asynchronous Data Fetching: Manages errors during data fetching operations like API requests, ensuring a smooth user experience.
- Dynamic Content Rendering: Intercepts errors in dynamically rendered content due to malformed data, preventing application breaks.
- Rendering Complex UIs: Captures errors in nested components within complex UI structures, aiding in debugging and maintenance.
- Cross-browser Compatibility: Addresses browser-specific errors for consistent functionality across different platforms and browsers.
Overall, componentDidCatch is a valuable tool for improving the robustness and reliability of React applications by gracefully handling errors and preventing them from disrupting the user experience.
Share your thoughts in the comments
Please Login to comment...