What is the purpose of the componentDidUpdate lifecycle method ?
Last Updated :
09 Feb, 2024
React web apps are actually a collection of independent components that run according to the interactions made with them. Every React Component has a lifecycle of its own, the lifecycle of a component can be defined as the series of methods that are invoked in different stages of the component’s existence.
Prerequisites:
What is componentDidUpdate lifecycle method?
The componentDidUpdate() method allows us to execute the React code when the component is updated. All the network requests that are to be made when the props are passed to the component changes are coded here.
Syntax:
componentDidUpdate(prevProps, prevState, snapshot)
- prevProps: Previous props passed to the component
- prevState: Previous state of the component
- snapshot: Value returned by getSnapshotBeforeUpdate() method
Purpose of the componentDidUpdate lifecycle method
1. Responding to Prop or State Changes: One of the primary purposes of componentDidUpdate
is to respond to changes in props or state. After a component’s props or state are updated, componentDidUpdate
allows you to perform actions based on these changes.
2. Performing Side Effects: componentDidUpdate
is commonly used for performing side effects that are dependent on component updates. This will include updating the document title, triggering imperative animations, integrating with third-party libraries, or interacting with the DOM directly.
3. Managing Subscriptions and Event Listeners: When working with subscriptions or event listeners, componentDidUpdate
can be used to subscribe to new data streams, update subscriptions, or attach event listeners. It ensures that these subscriptions are updated after each render, preventing memory leaks or stale data.
Steps for Creating React Application:
Step 1: Create a react application using the following command.
npx create-react-app foldername
Step 2: Once it is done, change your directory to the newly created application using the following command.
cd foldername
Project Structure:
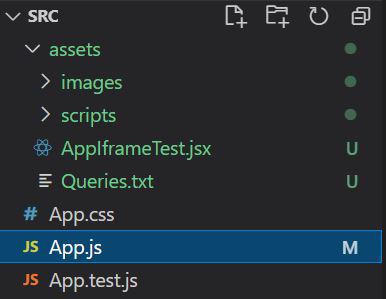
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: In this example, we are going to build a React application that changes the text in the heading when the state of the component is updated.
Javascript
import React from 'react' ;
class App extends React.Component {
state = {
company: 'GFG'
};
componentDidMount() {
setTimeout(() => {
this .setState({ company: 'GeeksForGeeks' });
}, 600);
}
componentDidUpdate() {
document.getElementById( 'disclaimer' ).innerHTML =
'P.s: GFG is also known as ' + this .state.company;
}
render() {
return (
<div>
<h2 style={{ color: 'green' }}>GeeksForGeeks</h2>
<h2>
Explain the purpose of the
componentDidUpdate lifecycle method.
</h2>
<div>
<h1 style={{
margin: 'auto' ,
width: '50%' ,
padding: 20,
marginTop: '10%' ,
border: 'solid 1px black' ,
textAlign: 'center' ,
fontSize: 18,
}}>
Greatest Science Portal For Geeks :
{ this .state.company}
<div id= "disclaimer" ></div>
</h1>
</div>
</div>
)
}
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...