Creating an injectable service in Angular
Last Updated :
22 Mar, 2024
In Angular, services are a great way to share data, functionality, and state across different components in your application. Services are typically injected into components and other services as dependencies, making them easily accessible and maintainable. Mostly services are used to create functions that initiate HTTP calls.
What is an Injectable Service?
An injectable service in Angular is a TypeScript class decorated with the @Injectable()
decorator. This decorator provides metadata that allows Angular’s dependency injection system to create and manage instances of the service class. Injectable services play a fundamental role in Angular development as they provide a way to share functionality, data, and state across different parts of the application.
Syntax:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root' // or 'any' or specific module
})
export class MyService {
constructor() { }
// Service logic here
}
Steps to Create An Injectable Service:
Step 1: Setting Up Angular Project
Install Angular CLI globally (if not already installed)
npm install -g @angular/cli
Step 2: Create a new Angular project:
ng new injectible-service-demo
Step 3: Once the project is set up, navigate to the src/app directory. Inside it, create a new folder called “core”. Within the “core” folder, create another folder named “services”.
Step 4: Generate a new service using the angular cli after going into services folder using the below command:
ng generate service my-service
Folder Structure:
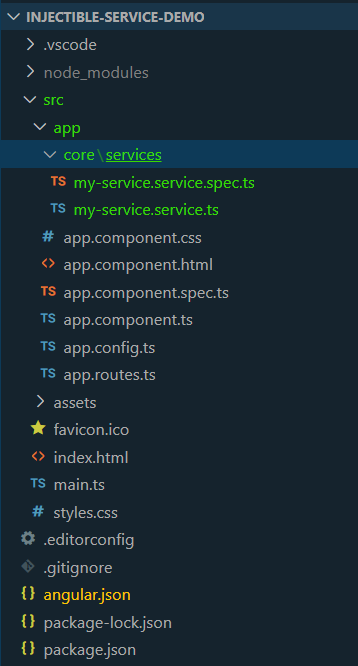
Folder Structure
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
"devDependencies": {
"@angular-devkit/build-angular": "^17.3.0",
"@angular/cli": "^17.3.0",
"@angular/compiler-cli": "^17.3.0",
"@types/jasmine": "~5.1.0",
"jasmine-core": "~5.1.0",
"karma": "~6.4.0",
"karma-chrome-launcher": "~3.2.0",
"karma-coverage": "~2.2.0",
"karma-jasmine": "~5.1.0",
"karma-jasmine-html-reporter": "~2.1.0",
"typescript": "~5.4.2"
}
Example of Injectable Services in Angular
Code: Add the following codes in respective files.
HTML
<!-- app.component.html -->
<div>
<button (click)="addItem()">Add Item</button>
<ul>
<li *ngFor="let item of items">{{ item | json }}</li>
</ul>
</div>
JavaScript
//app.component.ts
import { Component } from '@angular/core';
import { RouterOutlet } from '@angular/router';
import { MyService } from './core/services/my-service.service';
import { CommonModule } from '@angular/common';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, CommonModule],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
export class AppComponent {
title = 'injectible-service-demo';
items: any[] = [];
constructor(private myService: MyService) {
this.items = this.myService.getData();
}
addItem() {
const newItem = { id: Date.now(), value: 'New Item' };
this.myService.addData(newItem);
this.items = this.myService.getData();
}
}
JavaScript
//my-service.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class MyService {
private data: any[] = [];
getData() {
return this.data;
}
addData(item: any) {
this.data.push(item);
}
}
Output:
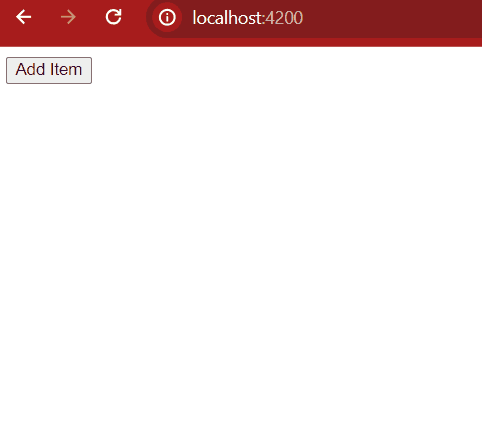
Creating an injectable service – Angular
Like Article
Suggest improvement
Share your thoughts in the comments
Please Login to comment...