CSS Viewer Cube in React without Three.js
Last Updated :
12 Mar, 2024
A CSS viewer cube is a visually appealing and interactive way to display images or content on a webpage. It resembles a 3D cube that can be rotated along its axes to reveal different faces. While traditionally, libraries like Three.js have been used to achieve this effect.
In this article, we’ll explore how to create a CSS viewer cube in React without relying on Three.js.
We will discuss about the approaches of creating a CSS viewer cube without Three.js using React:
Steps to Create a React Application and Installing Module:
Step 1: Create a React App using the following command.
npx create-react-app my-react-app
Step 2: Switch to the project directory
cd my-react-app
Step 3: Installing the required packages:
npm install styled-components react-icons
Updated Dependencies in package.json File:
"dependencies": {
"react": "^17.0.2",
"react-dom": "^17.0.2",
"styled-components": "^5.3.3",
"react-icons": "^4.3.1"
}
Example: This approach involves defining CSS animations for the cube’s rotation and using CSS transforms to manipulate its orientation.
Javascriptimport React from 'react';
import styled, {
keyframes
} from 'styled-components';
const rotateAnimation = keyframes`
from {
transform: rotateX(0deg) rotateY(0deg);
}
to {
transform: rotateX(360deg) rotateY(360deg);
}
`;
const CubeContainer = styled.div`
width: 200px;
height: 200px;
position: relative;
perspective: 800px;
`;
const Cube = styled.div`
width: 100%;
height: 100%;
position: absolute;
transform-style: preserve-3d;
animation: ${rotateAnimation} 10s infinite linear;
`;
const CubeFace = styled.div`
width: 200px;
height: 200px;
position: absolute;
background-color: ${({ color }) => color};
border: 2px solid black;
`;
const FrontFace = styled(CubeFace)`
transform: translateZ(100px);
`;
const BackFace = styled(CubeFace)`
transform: translateZ(-100px) rotateY(180deg);
`;
const RightFace = styled(CubeFace)`
transform: rotateY(90deg) translateZ(100px);
`;
const LeftFace = styled(CubeFace)`
transform: rotateY(-90deg) translateZ(100px);
`;
const TopFace = styled(CubeFace)`
transform: rotateX(90deg) translateZ(100px);
`;
const BottomFace = styled(CubeFace)`
transform: rotateX(-90deg) translateZ(100px);
`;
const CubeViewer = () => {
return (
<CubeContainer>
<Cube>
<FrontFace color="red"></FrontFace>
<BackFace color="blue"></BackFace>
<RightFace color="green"></RightFace>
<LeftFace color="yellow"></LeftFace>
<TopFace color="orange"></TopFace>
<BottomFace color="purple"></BottomFace>
</Cube>
</CubeContainer>
);
};
export default CubeViewer;
Output:
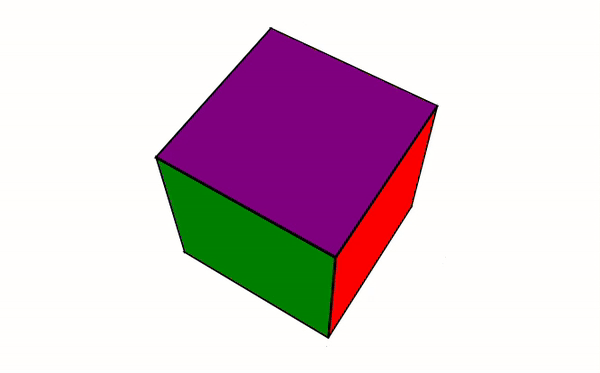
CSS Viewer Cube React State and Event Handling:
Example: Here, we’ll use React state to store the current rotation state of the cube and handle events to update it accordingly.
Javascriptimport React, { useState, useEffect } from 'react';
import styled from 'styled-components';
const CubeContainer = styled.div`
width: 200px;
height: 200px;
position: relative;
perspective: 800px;
margin:250px;
`;
const Cube = styled.div`
width: 100%;
height: 100%;
position: absolute;
transform-style: preserve-3d;
transform: ${({ rotation }) => rotation};
`;
const CubeFace = styled.div`
width: 200px;
height: 200px;
position: absolute;
background-color: ${({ color }) => color};
border: 2px solid black;
`;
const FrontFace = styled(CubeFace)`
transform: translateZ(100px);
`;
const BackFace = styled(CubeFace)`
transform: translateZ(-100px) rotateY(180deg);
`;
const RightFace = styled(CubeFace)`
transform: rotateY(90deg) translateZ(100px);
`;
const LeftFace = styled(CubeFace)`
transform: rotateY(-90deg) translateZ(100px);
`;
const TopFace = styled(CubeFace)`
transform: rotateX(90deg) translateZ(100px);
`;
const BottomFace = styled(CubeFace)`
transform: rotateX(-90deg) translateZ(100px);
`;
const CubeViewer = () => {
const [rotation, setRotation] = useState({ x: 0, y: 0 });
useEffect(() => {
const handleMouseMove = (e) => {
setRotation({ x: -e.clientY, y: e.clientX });
};
document.addEventListener('mousemove',
handleMouseMove);
return () => {
document.removeEventListener('mousemove',
handleMouseMove);
};
}, []);
return (
<CubeContainer>
<Cube rotation={`
rotateX(${rotation.x}deg) rotateY(${rotation.y}deg)`}>
<FrontFace color="red"></FrontFace>
<BackFace color="blue"></BackFace>
<RightFace color="green"></RightFace>
<LeftFace color="yellow"></LeftFace>
<TopFace color="orange"></TopFace>
<BottomFace color="purple"></BottomFace>
</Cube>
</CubeContainer>
);
};
export default CubeViewer;
Output:
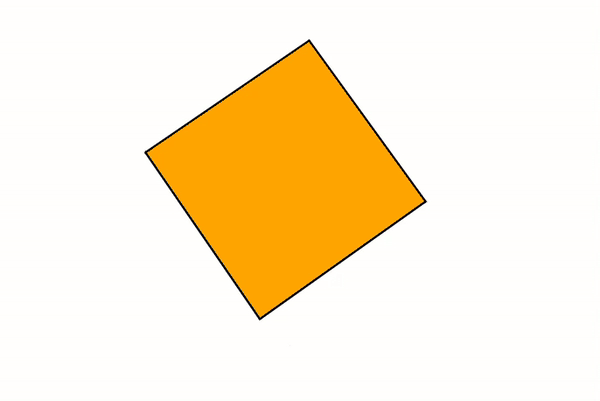
Conclusion:
Creating a CSS viewer cube in React without using Three.js is achievable through CSS animations, React state management, and CSS layout techniques like Grid or Flexbox. By combining these approaches, you can create engaging and interactive 3D effects for your React applications.
Share your thoughts in the comments
Please Login to comment...