GreenSock ScrollTrigger with React JS
Last Updated :
17 Dec, 2023
GreenSock Animation Platform (GSAP) is a powerful JavaScript library that is used by front-end developers to create robust timeline-based animations. GSAP is used to animate anything that can be accessed with JavaScript.
Prerequisites:
Approach to create GreenSock ScollTrigger:
- Create a new functional component and then import GSAP and ScrollTrigger and register the plugin. Registering a plugin ensures that it works smoothly and prevents tree-shaking issues in build tools/bundlers.
- We will use React useRef to reference the component whenever it mounts, once we have the reference to the object, then we can easily apply animations to it. We will be putting the animation code inside a useEffect so that the components animate only once when the browser loads.
- We will use the gsap.fromTo() tween with scrollTrigger.
Syntax:
gsap.fromTo(target_element, { from_parameter }, { To_parameter, scrollTrigger: {
trigger: target_element
}})
Steps to install the ScrollTrigger Animation in React Project
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: Installing Required module
npm install gsap
Step 4: Import the GSAP library into your App.js file:
import gsap from 'gsap';
import { ScrollTrigger } from "gsap/ScrollTrigger";
Project Structure:
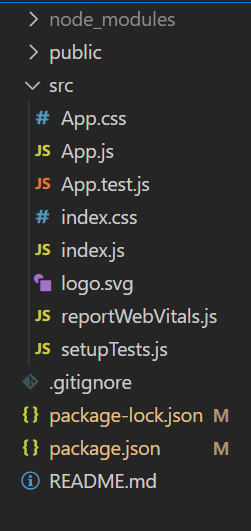
The updated dependencies in package.json file will look like:
"dependencies": {
"gsap": "^3.12.3",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example 1: we will try to animate the element’s scale when it comes into view while scrolling. In the code, the scale is set to 0 in the “from parameter”, and in the “to parameter”, it is set to 1.4. That means when the element will come into view while scrolling, the size of the element will update from 0 to 1.4 in the duration of 1 second.
Javascript
import React from 'react'
import './App.css'
import { useEffect, useRef } from 'react' ;
import gsap from 'gsap' ;
import { ScrollTrigger } from 'gsap/ScrollTrigger' ;
gsap.registerPlugin(ScrollTrigger);
const App = () => {
const ref = useRef();
useEffect(() => {
const el = ref.current;
gsap.fromTo(el, { scale: 0 }, {
scale: 1.4, duration: 1, scrollTrigger: {
trigger: el
}
})
}, [])
return (
<div className= 'App' >
<div style={{ height: '100vh' }}></div>
<img ref={ref}
src=
alt= "" />
</div>
)
}
export default App;
|
Steps to run: Start the server by typing the following command in your terminal:
npm start
Output: Now open your browser and go to http://localhost:3000/, you will see the following output:
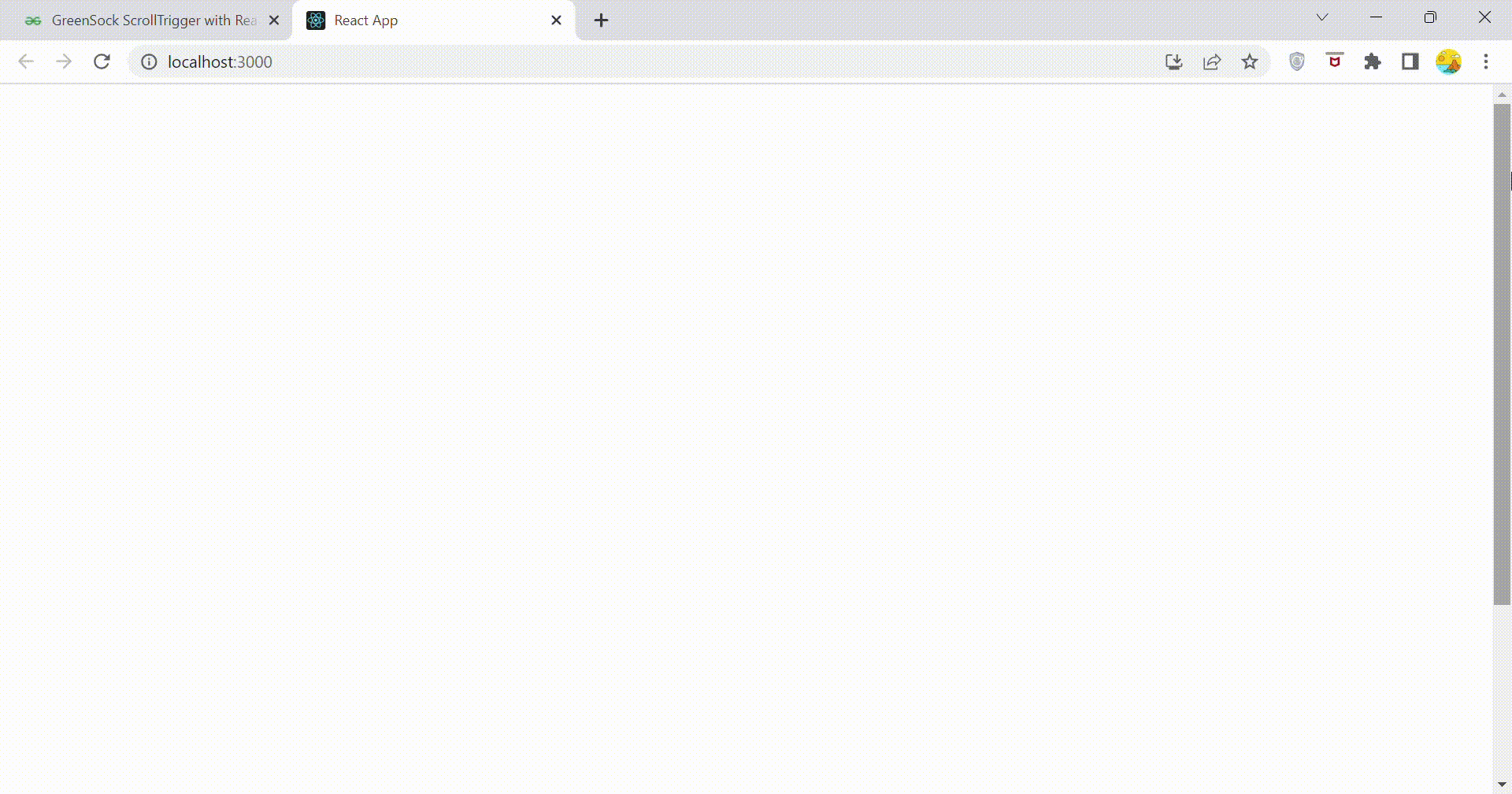
Example 2: We will animate several components by using an array of refs. For that, there is the addtoRefs function which keeps adding the references of the box components one by one to the array when they come into view while scrolling
Javascript
import React from 'react'
import './App.css'
import { useEffect, useRef } from 'react' ;
import gsap from 'gsap' ;
import { ScrollTrigger } from 'gsap/ScrollTrigger' ;
gsap.registerPlugin(ScrollTrigger);
const App = () => {
const ref = useRef([]);
ref.current = [];
useEffect(() => {
ref.current.forEach((el) => {
gsap.fromTo(el, { autoAlpha: 0 }, {
autoAlpha: 1, left: 0,
duration: 0.5, scrollTrigger: {
trigger: el,
start: "top bottom-=100" ,
toggleActions: "play none none reverse"
}
})
})
}, [])
const addtoRefs = (el) => {
if (el && !ref.current.includes(el)) {
ref.current.push(el);
}
}
return (
<div className= 'App' >
<div className= 'divSpace' >Scroll Down</div>
<div className= 'box-container' >
<div ref={addtoRefs} className= "box" >box1</div>
<div ref={addtoRefs} className= "box" >box2</div>
<div ref={addtoRefs} className= "box" >box3</div>
<div ref={addtoRefs} className= "box" >box4</div>
<div ref={addtoRefs} className= "box" >box5</div>
<div ref={addtoRefs} className= "box" >box6</div>
<div ref={addtoRefs} className= "box" >box7</div>
</div>
</div>
)
}
export default App;
|
CSS
body {
margin : 0 ;
padding : 0 ;
box-sizing: border-box;
scroll-behavior: smooth;
overflow-x: hidden ;
}
.divSpace {
height : 100 vh;
width : 100 vw;
display : flex;
justify- content : center ;
align-items: center ;
font-size : 40px ;
}
.box-container {
min-height : 100 vh;
width : 100 vw;
display : grid;
justify- content : center ;
align-items: center ;
margin-bottom : 400px ;
}
.box {
height : 50px ;
width : 200px ;
text-align : center ;
position : relative ;
left : -200px ;
}
.box:nth-child( 1 ) {
background-color : green ;
}
.box:nth-child( 2 ) {
background-color : crimson;
}
.box:nth-child( 3 ) {
background-color : orange;
}
.box:nth-child( 4 ) {
background-color : yellow;
}
.box:nth-child( 5 ) {
background-color : green ;
}
.box:nth-child( 6 ) {
background-color : crimson;
}
.box:nth-child( 7 ) {
background-color : orange;
}
|
Steps to Run: Start the server by typing the following command in your terminal:
npm start
Output: Now open your browser and go to http://localhost:3000/
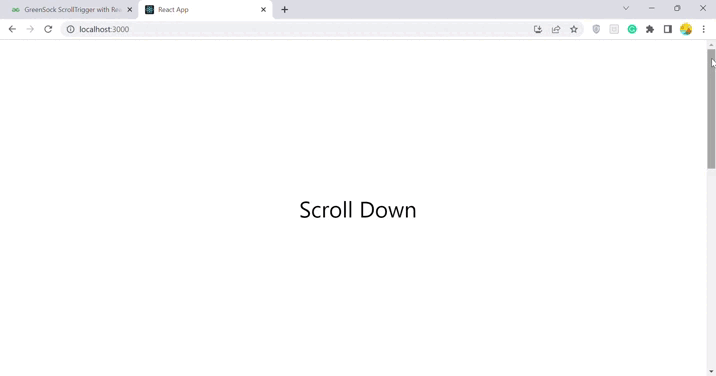
Share your thoughts in the comments
Please Login to comment...