Exploring React Reveal: Creating Stunning Animations in React
Last Updated :
12 Dec, 2023
React Reveal is an animation library for React. In this article, we will see few of the implementation of this library and learn more about it.
We will discuss the following two approaches:
Prerequisites
Understanding React Reveal
React Reveal is a React library that makes it easy to implement animations in web applications. It can be used to create various looping animations in your application. We can easily create interactive animation in our application using this library.
Steps to create a React app and install React Reveal
Step 1: Create a React application using the commands given below.
npx create-react-app foldername
Step 2: Once you have created the project folder (folder name), navigate to it using the following command:
cd foldername
Step 3: After creating the ReactJS application, install React Reveal using the command below.
npm install react-reveal
Folder Structure:
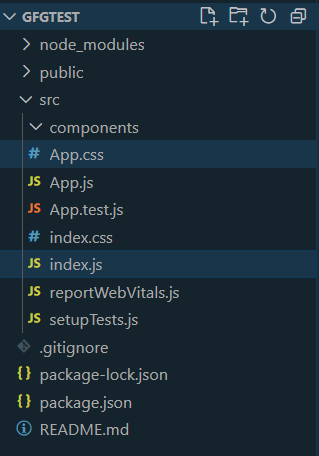
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-reveal": "^1.2.2",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Approach 1: Using the Fade Effect
The fade effect gradually fades out of an element by adjusting its transparency. To apply the fade effect to Read Reveal, import the fade component and cover your target element. Here’s an example:
Javascript
import React from 'react' ;
import Fade from 'react-reveal/Fade' ;
const App = () => {
return (
<div>
<Fade>
<h1>Geeks For Geeks</h1>
</Fade>
</div>
);
};
export default App;
|
Output:
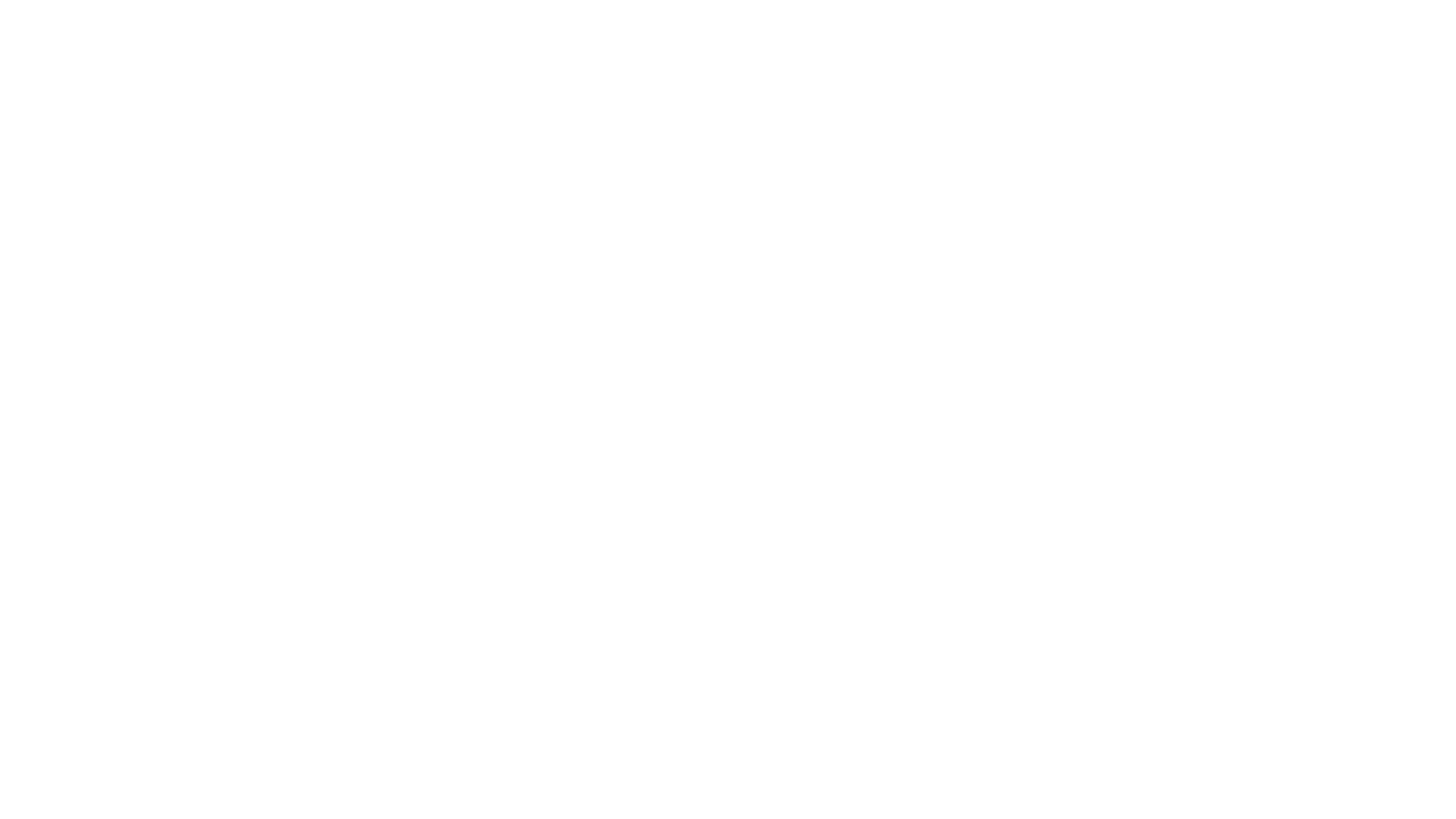
Fade effect Using React Reveal
Approch 2: Using the slide Effect
The slide effect allows you to visually animate elements. You can control the slide direction and distance. Here’s an example:
Javascript
import React from 'react' ;
import Slide from 'react-reveal/Slide' ;
const App = () => {
return (
<div>
<Slide right>
<h1>Sliding Heading</h1>
</Slide>
</div>
);
};
export default App;
|
Output:
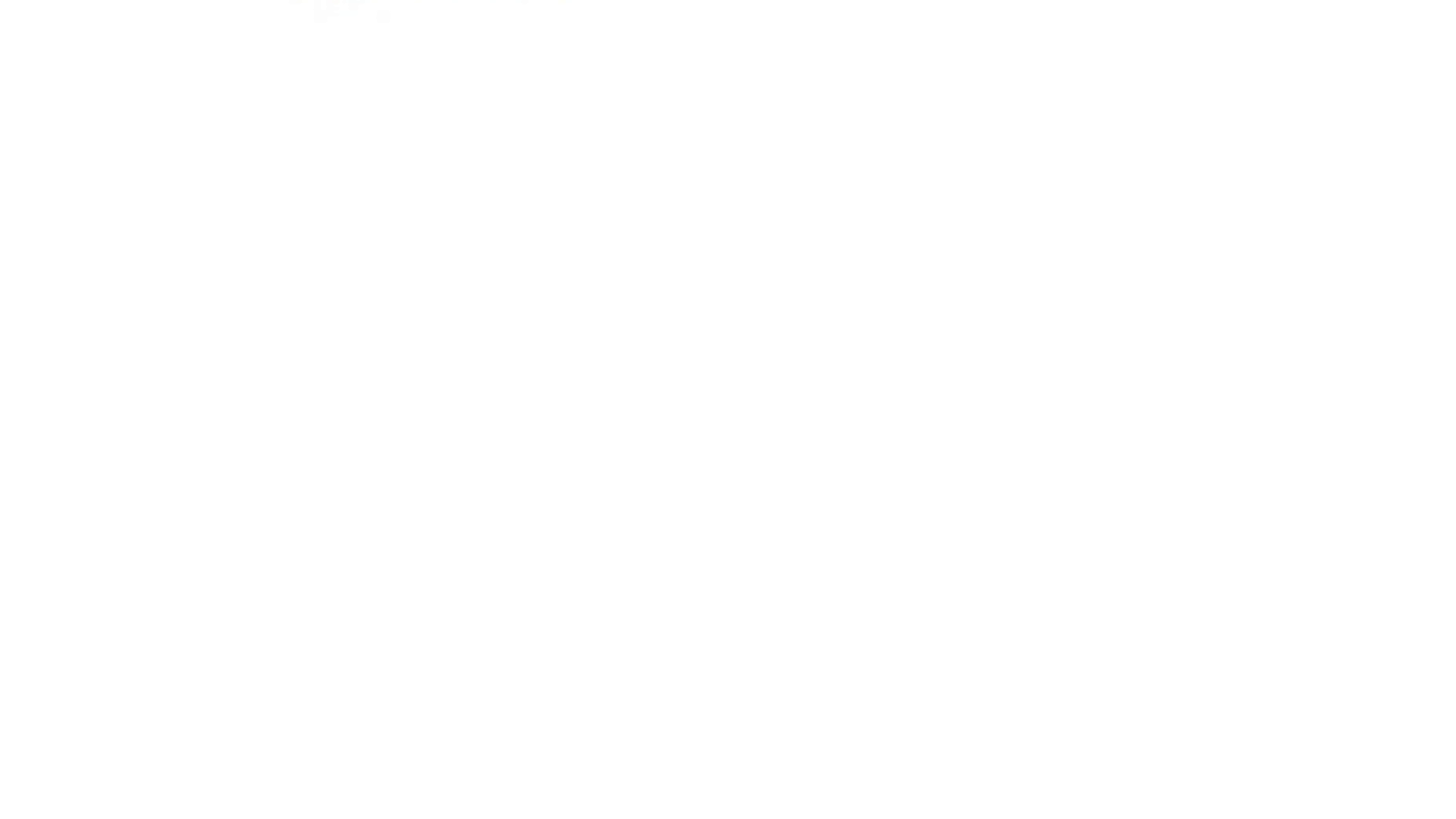
Slide Right Animation using React Reveal
Approach 3: Combining Animation and Sequence
React Reveal also supports combining multiple animations and creating complex sequences. This allows you to set multiple animations to achieve more dynamic effects. Here’s an example:
Javascript
import React from 'react' ;
import { Fade, Rotate } from 'react-reveal' ;
const App = () => {
return (
<div>
<Fade>
<h1>Geeks For Geeks</h1>
</Fade>
<Rotate>
<p>Rotating Paragraph</p>
</Rotate>
</div>
);
};
export default App;
|
Output:
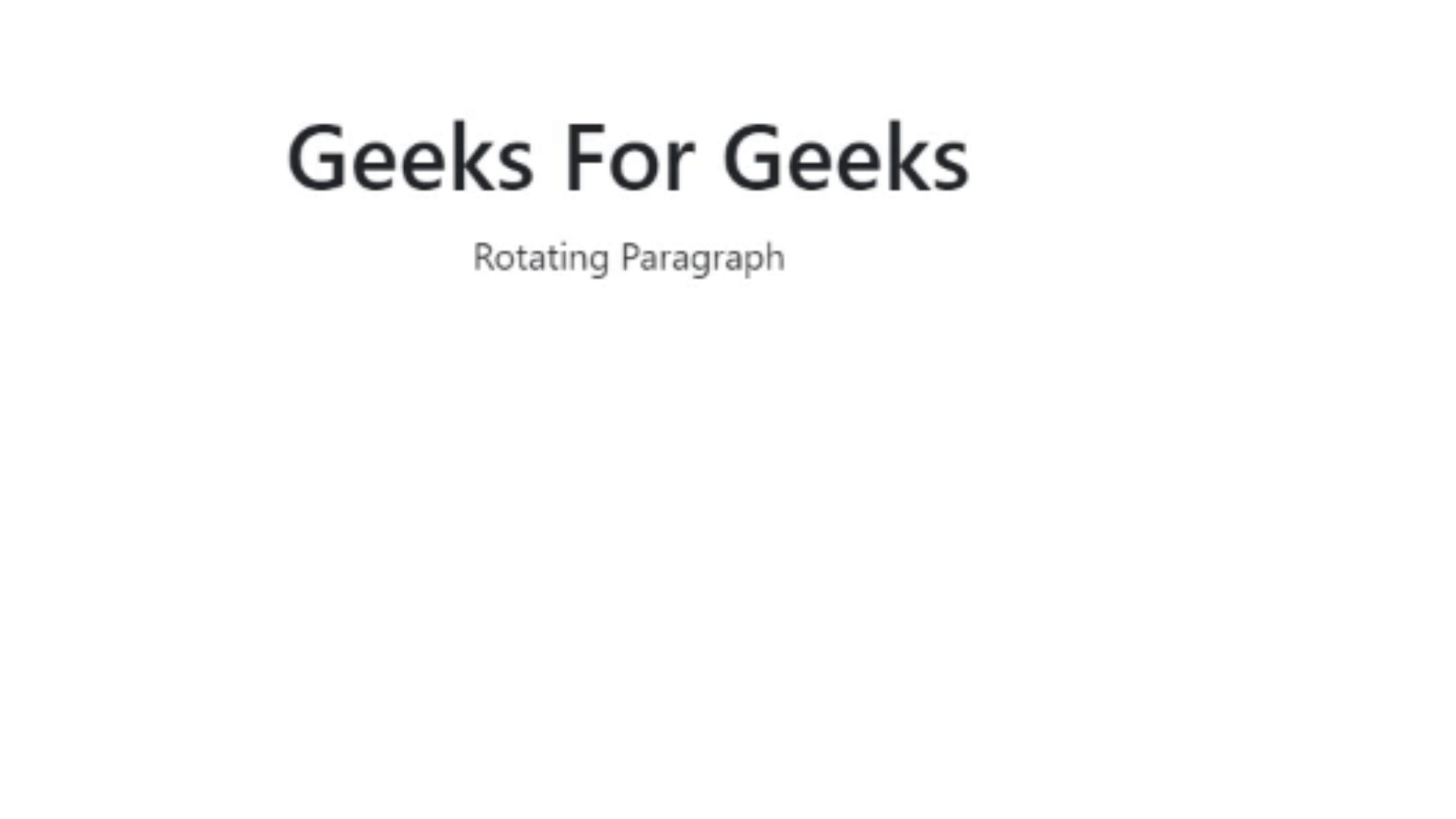
Combining Animation Using React Reveal
Share your thoughts in the comments
Please Login to comment...