Using a http service in Angular 17 with standalone components
Last Updated :
17 Apr, 2024
In Angular 17, they’ve introduced standalone components, which change how you build and organize Angular applications. These components are self-sufficient and can be used on their own without being tied to a specific NgModule. But, sometimes, when you’re working with these standalone components, you might need to fetch data from servers or interact with APIs using HTTP requests. This article will guide you through various ways of incorporating an HTTP service into your Angular 17 standalone components.
Steps to use HTTP service in Angular
Step 1: Set up a new Angular 17 project
ng new my-app
Note: Don’t forget to select “Yes” for server side rendering.

Folder Structure:
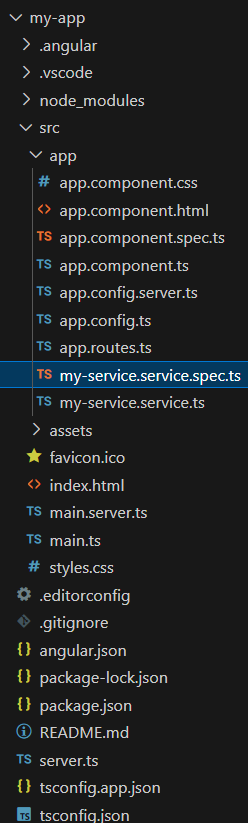
Dependencies:
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
}
Step 2: To start the application run the following command.
ng serve
Approach 1: Injecting the HttpClient service directly into the component
This approach involves importing the HttpClient service from the @angular/common/http module and injecting it into the component’s constructor using Angular’s dependency injection system.
Code Example:
HTML
<!-- app.component.html -->
<div>
<h4>{{post | json}}</h4>
</div>
JavaScript
//app.component.ts
import { CommonModule, JsonPipe, NgIf } from '@angular/common';
import { HttpClient, HttpClientModule } from '@angular/common/http';
import { Component, inject } from '@angular/core';
import { RouterOutlet } from '@angular/router';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, JsonPipe, HttpClientModule],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
export class AppComponent {
private http = inject(HttpClient);
post: any;
constructor() {
this.http.get('https://jsonplaceholder.typicode.com/posts/1')
.subscribe(data => {
this.post = data;
});
}
}
Output:
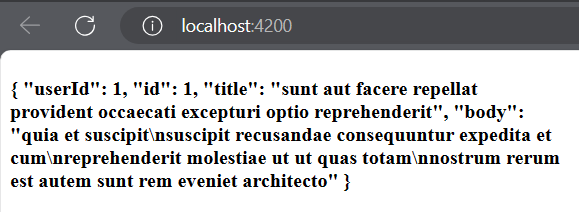
Approach 2: Creating a standalone service with HttpClient injected
In this approach, you create a separate standalone service that encapsulates the HTTP functionality. The HttpClient service is injected into this standalone service, which can then be used by multiple components.
Step 1: Create a new service using the following command.
ng g service my-service
JavaScript
//main.ts
import { bootstrapApplication } from '@angular/platform-browser';
import { AppComponent } from './app/app.component';
import { provideHttpClient, withFetch } from '@angular/common/http';
import { provideRouter } from '@angular/router';
import { routes } from './app/app.routes';
bootstrapApplication(AppComponent, {
providers: [
provideRouter(routes),
provideHttpClient()
]
}).catch((err) =>
console.error(err)
);
JavaScript
//app.component.ts
import { CommonModule, JsonPipe, NgIf } from '@angular/common';
import { HttpClient, HttpClientModule } from '@angular/common/http';
import { Component, inject } from '@angular/core';
import { RouterOutlet } from '@angular/router';
import { MyServiceService } from './my-service.service';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, JsonPipe, HttpClientModule],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
export class AppComponent {
post: any;
constructor(private myService: MyServiceService) {
this.post = this.myService.getData();
}
}
JavaScript
//my-service.service.ts
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class MyServiceService {
constructor(private httpClient: HttpClient) { }
getData() {
this.httpClient.get('https://jsonplaceholder.typicode.com/posts/1')
.subscribe(data => {
return data;
});
}
}
Output:
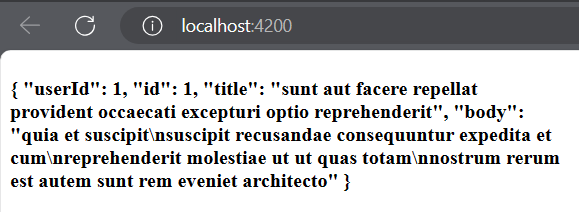
Approach 3: Using the HttpClientModule in a shared NgModule
Although standalone components are designed to be self-contained, you can still create a shared NgModule that provides the HttpClientModule and other services or pipes that may be required by multiple components.
Step 1: Create a new NgModule:
ng g module shared
JavaScript
//shared.module.ts
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
imports: [
HttpClientModule
],
exports: [
HttpClientModule
]
})
export class SharedModule { }
JavaScript
//app.component.ts
//import the SharedModule:
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet, NgIf, JsonPipe, SharedModule],
templateUrl: './app.component.html',
styleUrl: './app.component.css'
})
Output:
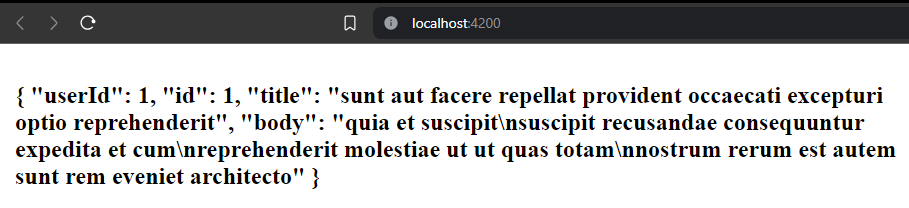
Share your thoughts in the comments
Please Login to comment...