cin in C++
Last Updated :
29 Jul, 2021
The cin object in C++ is an object of class iostream. It is used to accept the input from the standard input device i.e. keyboard. It is associated with the standard C input stream stdin. The extraction operator(>>) is used along with the object cin for reading inputs. The extraction operator extracts the data from the object cin which is entered using the keyboard.
Program 1:
Below is the C++ program to implement cin object:
C++
#include <iostream>
using namespace std;
int main()
{
string s;
cin >> s;
cout << s;
return 0;
}
|
Input:
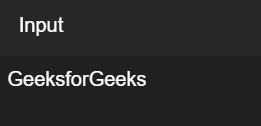
Output:
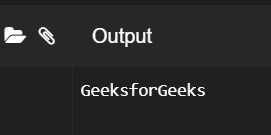
Program 2:
Multiple inputs using the extraction operators(>>) with cin. Below is the C++ program to take multiple user inputs:
C++
#include <iostream>
using namespace std;
int main()
{
string name;
int age;
cin >> name >> age;
cout << "Name : " << name << endl;
cout << "Age : " << age << endl;
return 0;
}
|
Input:
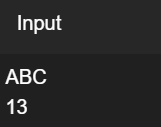
Output:
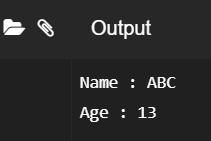
The cin can also be used with some member functions which are as follows:
cin.getline(char *buffer, int N):
It reads a stream of characters of length N into the string buffer, It stops when it has read (N – 1) characters or it finds the end of the file or newline character(\n). Below is the C++ program to implement cin.getline():
C++
#include <iostream>
using namespace std;
int main()
{
char name[5];
cin.getline(name, 3);
cout << name << endl;
return 0;
}
|
Input:
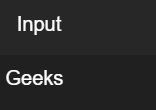
Output:
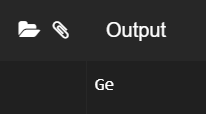
cin.get(char& var):
It reads an input character and stores it in a variable. Below is the C++ program to implement cin.get():
C++
#include <iostream>
using namespace std;
int main()
{
char ch;
cin.get(ch, 25);
cout << ch;
}
|
Input:
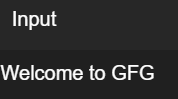
Output:
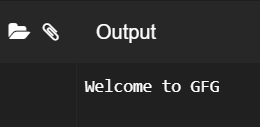
cin.read(char *buffer, int N):
Reads a stream of characters of length N. Below is the C++ program to implement cin.read():
C++
#include <iostream>
using namespace std;
int main()
{
char gfg[20];
cin.read(gfg, 10);
cout << gfg << endl;
return 0;
}
|
Input:
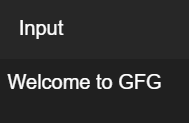
Output:
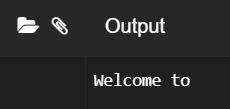
cin.ignore():
It ignores or clears one or more characters from the input buffer. Below is the C++ program to implement cin.ignore():
C++
#include <iostream>
#include <ios>
#include <limits>
using namespace std;
int main()
{
int x;
char str[80];
cout << "Enter a number andstring:\n" ;
cin >> x;
cin.ignore(numeric_limits<streamsize>::max(), '\n' );
cin.getline(str, 80);
cout << "You have entered:\n" ;
cout << x << endl;
cout << str << endl;
return 0;
}
|
Input:
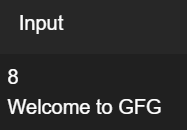
Output:
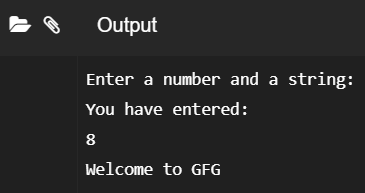
Explanation: In the above program if cin.ignore() has not been used then after entering the number when the user presses the enter to input the string, the output will be only the number entered. The program will not take the string input. To avoid this problem cin.ignore() is used, this will ignore the newline character.
Share your thoughts in the comments
Please Login to comment...