Check if two given Circles are Orthogonal or not
Last Updated :
19 Mar, 2022
Given are two circles with their centres C1(x1, y1) and C2(x2, y2) and radius r1 and r2, the task is to check if both the circles are orthogonal or not.
Two curves are said to be orthogonal if their angle of intersection is a right angle i.e the tangents at their point of intersection are perpendicular.
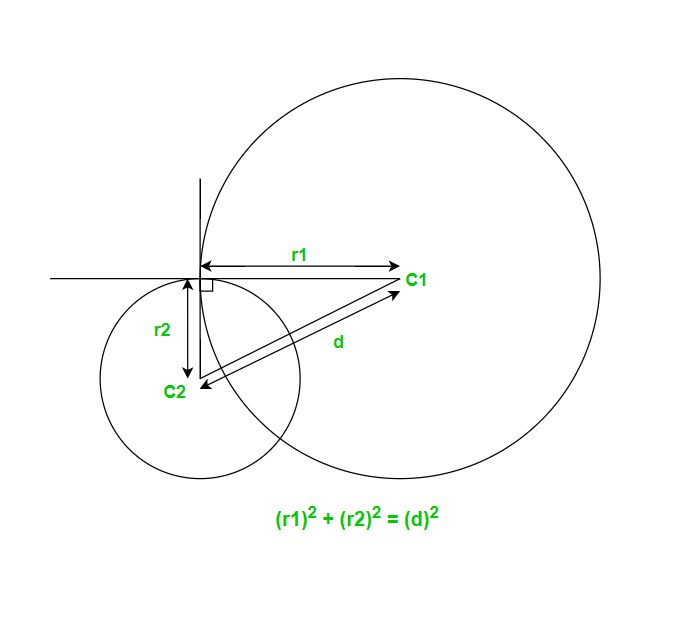
The above two circles are orthogonal
Examples:
Input: C1(4, 3), C2(0, 1), r1 = 2, r2 = 4
Output: Yes
Input: C1(4, 3), C2(1, 2), r1 = 2, r2 = 2
Output: No
Approach:
- Find the distance between the centres of two circles ‘d’ with distance formula.
- For the circles to be orthogonal we need to check if
r1 * r1 + r2 * r2 = d * d
- If it is true, then both the circles are orthagonal. Else not.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
bool orthogonality( int x1, int y1, int x2,
int y2, int r1, int r2)
{
int dsquare = (x1 - x2) * (x1 - x2)
+ (y1 - y2) * (y1 - y2);
if (dsquare == r1 * r1 + r2 * r2)
return true ;
else
return false ;
}
int main()
{
int x1 = 4, y1 = 3;
int x2 = 0, y2 = 1;
int r1 = 2, r2 = 4;
bool f = orthogonality(x1, y1, x2,
y2, r1, r2);
if (f)
cout << "Given circles are"
<< " orthogonal." ;
else
cout << "Given circles are"
<< " not orthogonal." ;
return 0;
}
|
Java
import java.util.*;
import java.lang.*;
import java.io.*;
class GFG
{
public static boolean orthogonality( int x1, int y1, int x2,
int y2, int r1, int r2)
{
int dsquare = (x1 - x2) * (x1 - x2) +
(y1 - y2) * (y1 - y2);
if (dsquare == r1 * r1 + r2 * r2)
return true ;
else
return false ;
}
public static void main(String[] args) throws java.lang.Exception
{
int x1 = 4 , y1 = 3 ;
int x2 = 0 , y2 = 1 ;
int r1 = 2 , r2 = 4 ;
boolean f = orthogonality(x1, y1, x2, y2, r1, r2);
if (f)
System.out.println( "Given circles are orthogonal." );
else
System.out.println( "Given circles are not orthogonal." );
}
}
|
Python3
def orthogonality(x1, y1, x2, y2, r1, r2):
dsquare = (x1 - x2) * (x1 - x2) + \
(y1 - y2) * (y1 - y2);
if (dsquare = = r1 * r1 + r2 * r2):
return True
else :
return False
x1, y1 = 4 , 3
x2, y2 = 0 , 1
r1, r2 = 2 , 4
f = orthogonality(x1, y1, x2, y2, r1, r2)
if (f):
print ( "Given circles are orthogonal." )
else :
print ( "Given circles are not orthogonal." )
|
C#
using System;
class GFG
{
public static bool orthogonality( int x1, int y1, int x2,
int y2, int r1, int r2)
{
int dsquare = (x1 - x2) * (x1 - x2) +
(y1 - y2) * (y1 - y2);
if (dsquare == r1 * r1 + r2 * r2)
return true ;
else
return false ;
}
public static void Main()
{
int x1 = 4, y1 = 3;
int x2 = 0, y2 = 1;
int r1 = 2, r2 = 4;
bool f = orthogonality(x1, y1, x2, y2, r1, r2);
if (f)
Console.WriteLine( "Given circles are orthogonal." );
else
Console.WriteLine( "Given circles are not orthogonal." );
}
}
|
Javascript
<script>
function orthogonality(x1, y1, x2, y2, r1, r2)
{
let dsquare = (x1 - x2) * (x1 - x2)
+ (y1 - y2) * (y1 - y2);
if (dsquare == r1 * r1 + r2 * r2)
return true ;
else
return false ;
}
let x1 = 4, y1 = 3;
let x2 = 0, y2 = 1;
let r1 = 2, r2 = 4;
let f = orthogonality(x1, y1, x2,
y2, r1, r2);
if (f)
document.write( "Given circles are orthogonal." );
else
document.write( "Given circles are not orthogonal." );
</script>
|
Output:
Given circles are orthogonal.
Time Complexity: O(1)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...