TCS Coding Practice Question | Reverse a Number
Last Updated :
15 Sep, 2023
Given a number, the task is to reverse this number using Command Line Arguments. Examples:
Input: num = 12345
Output: 54321
Input: num = 786
Output: 687
Approach:
- Since the number is entered as Command line Argument, there is no need for a dedicated input line
- Extract the input number from the command line argument
- This extracted number will be in String type.
- Convert this number into integer type and store it in a variable, say num
- Initialize a variable, say rev_num, to store the reverse of this number with 0
- Now loop through the number num till it becomes 0, i.e. (num > 0)
- In each iteration,
- Multiply rev_num by 10 and add remainder of num to it. This will store the last digit of num in rev_num
- Divide num by 10 to remove the last digit from it.
- After the loop has ended, rev_num has the reverse of num.
Program:
C++
#include <iostream>
#include <string>
#include <sstream>
using namespace std;
int reverseNumber( int num)
{
int rev_num = 0;
while (num > 0) {
rev_num = rev_num * 10 + num % 10;
num = num / 10;
}
return rev_num;
}
int main( int argc, char * argv[])
{
int num;
if (argc == 1)
cout << "No command line arguments found.\n" ;
else {
num = atoi (argv[1]);
cout << reverseNumber(num) << endl;
}
return 0;
}
|
C
#include <stdio.h>
#include <stdlib.h> /* atoi */
int reverseNumber( int num)
{
int rev_num = 0;
while (num > 0) {
rev_num = rev_num * 10 + num % 10;
num = num / 10;
}
return rev_num;
}
int main( int argc, char * argv[])
{
int num;
if (argc == 1)
printf ( "No command line arguments found.\n" );
else {
num = atoi (argv[1]);
printf ( "%d\n" , reverseNumber(num));
}
return 0;
}
|
Java
class GFG {
public static int reverseNumber( int num)
{
int rev_num = 0 ;
while (num > 0 ) {
rev_num = rev_num * 10 + num % 10 ;
num = num / 10 ;
}
return rev_num;
}
public static void main(String[] args)
{
if (args.length > 0 ) {
int num = Integer.parseInt(args[ 0 ]);
System.out.println(reverseNumber(num));
}
else
System.out.println( "No command line "
+ "arguments found." );
}
}
|
Python
import sys
def reverseNumber(num):
rev_num = 0
while num > 0 :
rev_num = rev_num * 10 + num % 10
num = num / / 10
return rev_num
if __name__ = = "__main__" :
if len (sys.argv) = = 1 :
print ( "No command line arguments found." )
else :
num = int (sys.argv[ 1 ])
print (reverseNumber(num))
|
Output:
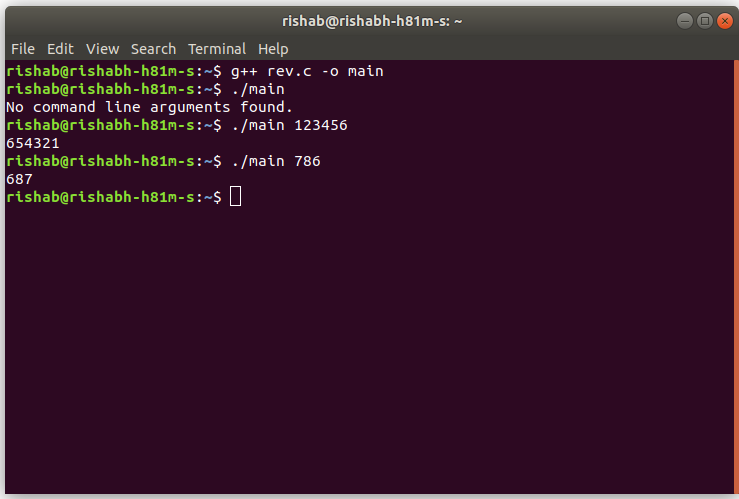
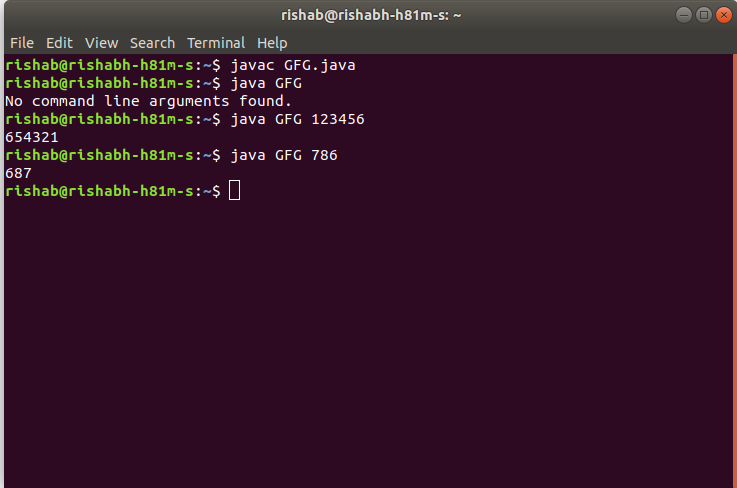
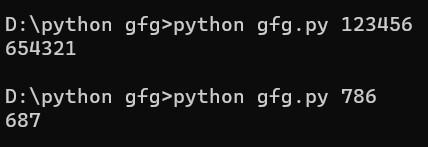
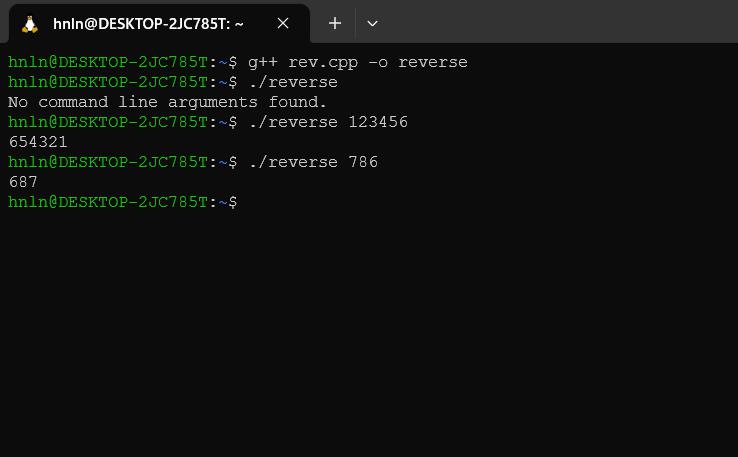
Share your thoughts in the comments
Please Login to comment...