C program to Find the Largest Number Among Three Numbers
Last Updated :
21 Sep, 2023
Here, we will learn how to find the largest number among the three numbers using the C program. For example, the largest number among 2, 8, and 1 is 8.
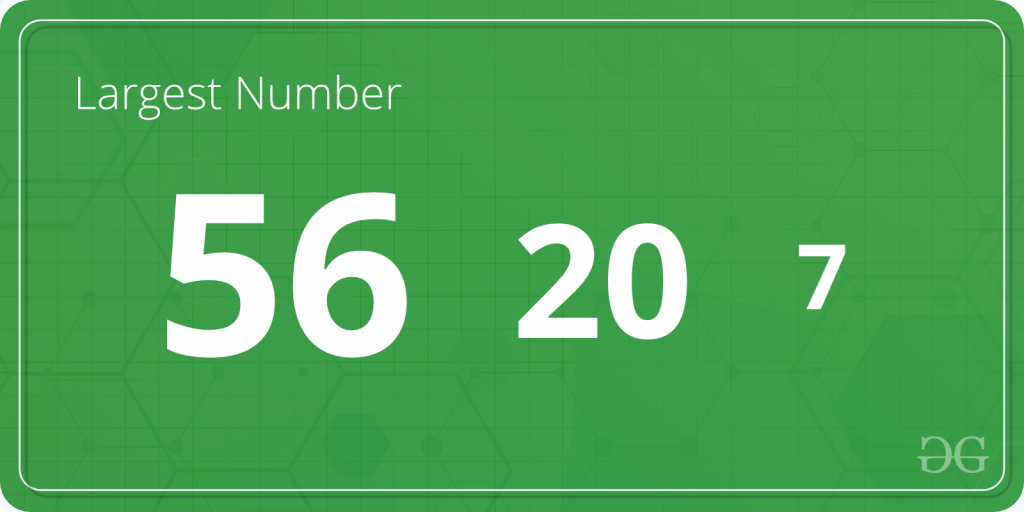
Methods to Find the Largest of Three Numbers
- Using if Statement
- Using if-else Statement
- Using nested if-else Statement
Let’s discuss each of them.
1. Find the Largest Number Using if-else Statements
The idea is to use the compound expression where each number is compared with the other two to find out which one is the maximum of them.
Algorithm
- Check if A is greater than or equal to both B and C, A is the largest number.
- Check if B is greater than or equal to both A and C, B is the largest number.
- Check if C is greater than or equal to both A and B, C is the largest number.
C Program to Find the Largest Number Using if Statement
C
#include <stdio.h>
int main()
{
int A, B, C;
printf ( "Enter the numbers A, B and C: " );
scanf ( "%d %d %d" , &A, &B, &C);
if (A >= B && A >= C)
printf ( "%d is the largest number." , A);
else if (B >= A && B >= C)
printf ( "%d is the largest number." , B);
else
printf ( "%d is the largest number." , C);
return 0;
}
|
Output
Enter the numbers A, B and C: 32764 is the largest number.
Complexity Analysis
- Time complexity: O(1) as it is doing constant operations
- Auxiliary space: O(1)
2. Find the Largest Number Using Nested if-else Statement
Unlike the previous method, we consider only one condition in each if statement and put the remaining condition inside it as a nested if-else statement.
C Program to Find the Largest Number Using if-else Statement
C
#include <stdio.h>
int main()
{
int A, B, C;
printf ( "Enter three numbers: " );
scanf ( "%d %d %d" , &A, &B, &C);
if (A >= B) {
if (A >= C)
printf ( "%d is the largest number." , A);
else
printf ( "%d is the largest number." , C);
}
else {
if (B >= C)
printf ( "%d is the largest number." , B);
else
printf ( "%d is the largest number." , C);
}
return 0;
}
|
Output
Enter three numbers: 32764 is the largest number.
Complexity Analysis
- Time complexity: O(1) as it is doing constant operations
- Auxiliary space: O(1)
3. Find the Largest Number Using Temporary Variable
In this method, we assume one of the numbers as maximum and assign it to a temporary variable. We then compare this temporary variable with the other two numbers one by one and if the max is smaller than the compared number, we assign the compared number to the max.
C Program to Find the Largest Number Among Three Using Temporary Variable
C
#include <stdio.h>
int main()
{
int a = 10, b = 25, c = 3;
int max = a;
if (max < b)
max = b;
if (max < c)
max = c;
printf ( "Maximum among %d, %d, and %d is: %d" , a, b, c,
max);
return 0;
}
|
Output
Maximum among 10, 25, and 3 is: 25
Complexity Analysis
- Time complexity: O(1) as it is doing constant operations
- Auxiliary space: O(1)
Related Articles
Share your thoughts in the comments
Please Login to comment...