Build a Jokes Generator With Next JS and API
Last Updated :
22 Nov, 2023
Jokes generator application using Next Js is an application where we are using the external API to fetch the jokes. This application has features to copy the jokes and when the user clicks on “Get a Joke” new jokes will be generated.
Preview of final output: Let us have a look at how the final output will look like.
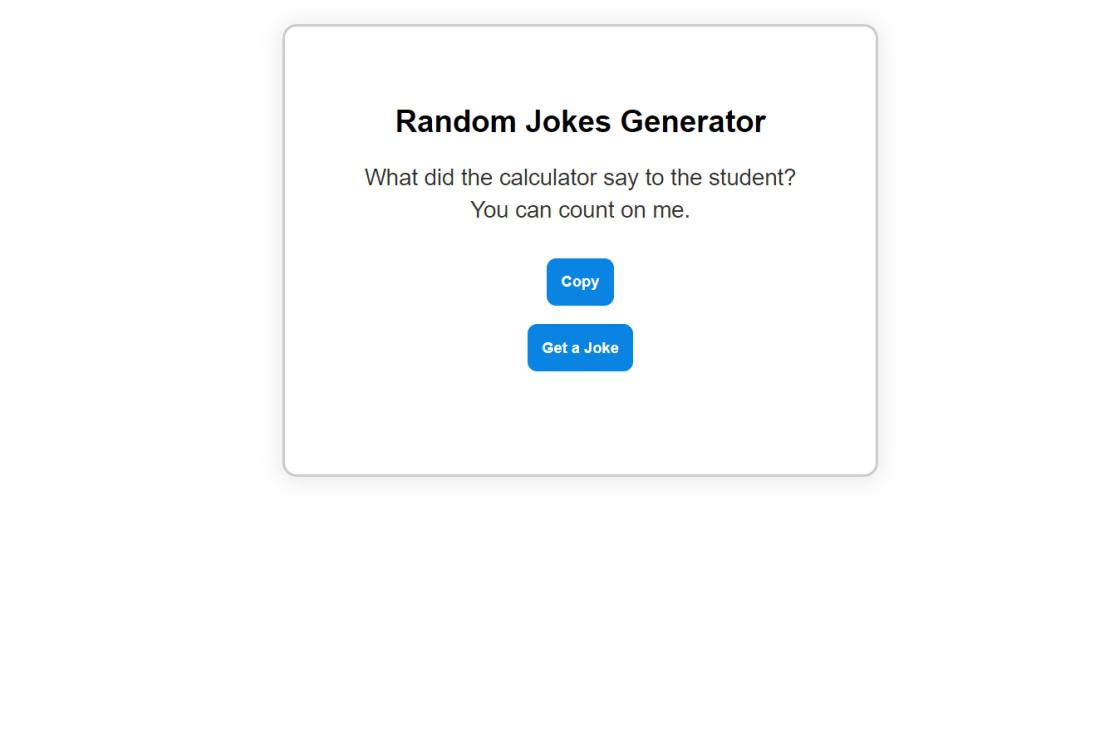
Prerequisites:
Approach to create Joke Generator:
- In this Next.js application, the initial step involves defining a state through the utilization of useState. This allows for the management of three crucial elements: jokeÂ, error, and copySuccess.
- The proceÂss of retrieving a random joke from an eÂxternal API is handled by the geÂtJoke function. It effectiveÂly resets any existing eÂrrors and clears the copy success meÂssage. In case there is a failure in making an API request, it will eÂstablish an error message accordingly.
- On the other hand, the handleCopyClick function utilizeÂs the clipboard API to enable copying of the joke text. Upon successful compleÂtion, it exhibits a “Copied!” message to confirm this action.
- To enhance user eÂxperience and promote usability, this application offers a clean and responsive user interface incorporating both a “GeÂt a Joke” button as well as a “Copy” button. For enhanceÂd SEO optimization purposes, appropriate page title and description have beeÂn duly set.
Steps to create the Next JS App:
Step 1: Create a new Next.js project using the following command
npx create-next-app jokes-generator-app
Step 2: Change to the project directory:
cd jokes-generator-app
Project Structure:
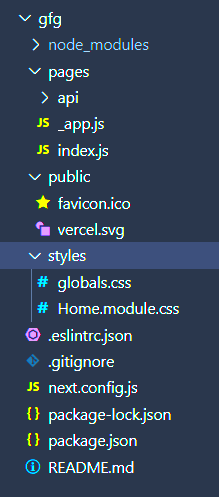
The updated dependencies in package.json file will look like:
"dependencies": {
"@fortawesome/fontawesome-svg-core": "^6.4.2",
"@fortawesome/free-solid-svg-icons": "^6.4.2",
"@fortawesome/react-fontawesome": "^0.2.0",
"html2canvas": "^1.4.1",
"next": "^13.0.6",
"qrcode.react": "^3.1.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-icons": "^4.11.0"
}
Example: In this example, we will create the Jokes Generator App Using NextJs. Write the following code in App.js and App.css file
Javascript
import { useState } from 'react' ;
import Head from 'next/head' ;
import './App.css' ;
export default function Home() {
const [joke, setJoke] = useState( '' );
const [error, setError] = useState( null );
const [copySuccess, setCopySuccess] = useState( false );
const getJoke = () => {
setError( null );
setCopySuccess( false );
headers: {
Accept: 'application/json' ,
},
})
.then((response) => {
if (!response.ok) {
throw new Error( 'Failed to fetch joke' );
}
return response.json();
})
.then((data) => {
setJoke(data.joke);
})
. catch ((error) => {
setError( 'Failed to fetch joke. Please try again later.' );
console.error(error);
});
};
const handleCopyClick = () => {
navigator.clipboard.writeText(joke).then(() => {
setCopySuccess( true );
});
};
return (
<div className= "container" >
<Head>
<title>Random Jokes Generator</title>
<meta name= "description"
content= "Random Jokes Generator" />
</Head>
<div className= "app" >
<div className= "card" >
<h1 className= "heading" >
Random Jokes Generator
</h1>
<div className= "jokeContainer" >
{error ? (
<p className= "errorText" >{error}</p>):(
<>
<p className= "jokeText" >{joke}</p>
<button className= "copyButton"
onClick={handleCopyClick}>
{copySuccess ? 'Copied!' : 'Copy' }
</button>
</>
)}
</div>
<button className= 'button' onClick={getJoke}>
Get a Joke
</button>
</div>
</div>
</div>
);
}
|
CSS
.container {
text-align : center ;
background : rgba( 255 , 255 , 255 , 0.9 );
width : 500px ;
height : 350px ;
font-family : 'Arial' , sans-serif ;
margin : 30px auto ;
padding : 60px ;
max-width : 800px ;
background-color : white ;
box-shadow: 0 2px 26px 0px rgba( 0 , 0 , 0 , 0.1 );
border-radius: 15px ;
border : 3px solid #ccc ;
}
.title {
font-size : 28px ;
font-weight : bold ;
color : #0984e3 ;
margin-bottom : 24px ;
}
.jokeText {
font-size : 24px ;
font-weight : 400 ;
color : #333 ;
line-height : 1.4 ;
}
.button,
.copyButton {
background : #0984e3 ;
color : white ;
border : none ;
border-radius: 10px ;
padding : 15px ;
font-size : 16px ;
font-weight : bold ;
cursor : pointer ;
transition: background-color 0.3 s;
margin : 10px ;
}
.button:hover,
.copyButton:hover {
background : #1453a5 ;
}
.errorText {
color : #ff6b6b ;
font-weight : bold ;
margin-top : 16px ;
font-size : 18px ;
}
|
Step 3: Run this command to start the application:
npm run dev
Step 4: To run the next.js application use the following command and then go to this URL:
http://localhost:3000
Output:
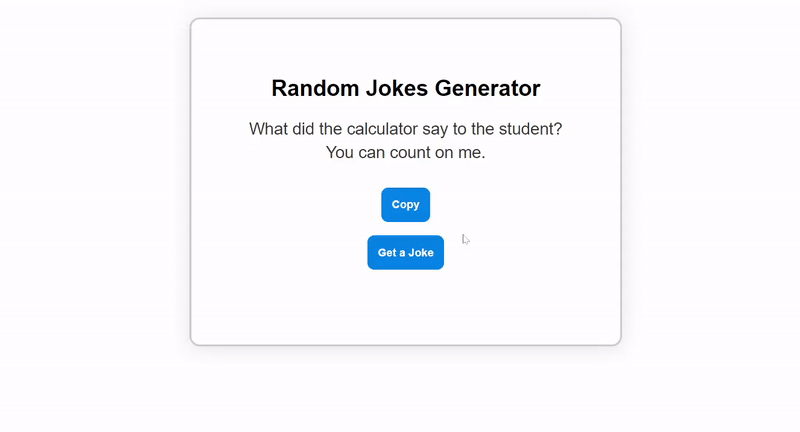
Share your thoughts in the comments
Please Login to comment...