Build a document generator with Express using REST API
Last Updated :
28 Dec, 2023
In the digital age, the need for dynamic and automated document generation has become increasingly prevalent. Whether you’re creating reports, invoices, or any other type of document, having a reliable system in place can streamline your workflow.
In this article, we’ll explore how to build a Document Generator using Node and Express, two powerful technologies for server-side JavaScript development.
Prerequisite
Approach to Create Document Generator:
- We’ll focus on a straightforward method using the Express.js framework and the pdfkit library to generate PDF documents. This approach is suitable for basic document generation needs. However, for more complex requirements, you might explore additional libraries or services.
Steps to Create Express Application:
Step 1: Create a directory in which the express application will store
mkdir Nodejs
cd Nodejs
Step 2: Initialize the project with the following command
npm init -y
Step 3: Install the required dependencies.
npm install pdfkit
Project Structure:
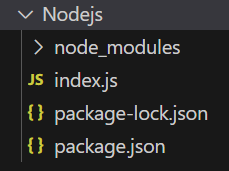
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"pdfkit": "^0.14.0",
}
Example: Building a document generator with Node.js and Express.js involves creating an API that receives requests, processes them, and generates the desired documents. In this example, I’ll demonstrate a simple document generator that generates PDF files using a popular library called pdfkit. You can install it using npm
Javascript
const express = require( 'express' );
const PDFDocument = require( 'pdfkit' );
const fs = require( 'fs' );
const app = express();
const port = 3000;
app.use(express.json());
app.post( '/generate-document' , (req, res) => {
const { title, content } = req.body;
const doc = new PDFDocument();
res.setHeader( 'Content-Type' , 'application/pdf' );
res.setHeader( 'Content-Disposition' , `attachment; filename=${title}.pdf`);
doc.pipe(res);
doc.fontSize(18).text(title, { align: 'center' });
doc.fontSize(12).text(content);
doc.end();
});
app.listen(port, () => {
console.log(`Server is running on http:
});
|
Steps to run server:
node app.js
Open command Prompt/terminal on your computer and run following command to test your document generator:
curl -X POST -H "Content-Type: application/json"
-d "{\"title\": \"My Document\", \"content\": \"Hello, this is the content of my document.\"}"
http://localhost:3000/generate-document > output.pdf
Now, type dir in cmd to see the output.pdf.
Output:
.gif)
output.pdf in home directory.
Share your thoughts in the comments
Please Login to comment...