Animation and Transitions using React Hooks
Last Updated :
03 Apr, 2024
Animations allows to control the elements by changing their motions or display. Animation is a technique to change the appearance and behaviour of various elements in web pages.
Transitions in CSS allow us to control the way in which transition takes place between the two states of the element. We can use the transitions to animate the changes and make the changes visually appealing to the user and hence, giving a better user experience and interactivity. In this article, we will learn simple animations and transitions using React hooks.
Handling animations in React using hooks like useEffect
 and useState
 along with CSS transitions or animation libraries provides a flexible and powerful way to create dynamic user interfaces.
Approach to Create Animations and Transitions using React Hooks:
We will be showing very simple examples of CSS animations and transitions on HTML elements and applying basic React hooks like useState and useEffect to manage the state of animation triggers, such as whether an element should be visible or hidden. The useEffect
 hook to control when animations should start or stop and maintain a incremental count. This could involve setting up timers (setInterval
, setTimeout
) or responding to changes in state.
Steps to Create React Application And Installing Module:
Step 1:Â Create a React application using the following command:
npx create-react-app react-animation-transition
Step 2: After creating your project folder(i.e. react-animation-transition), move to it by using the following command:
cd react-animation-transition
Step 3:Â After creating the React application, Install the required package using the following command:
npm i @chakra-ui/react @emotion/react@^11 @emotion/styled@^11 framer-motion@^6
Project Structure:
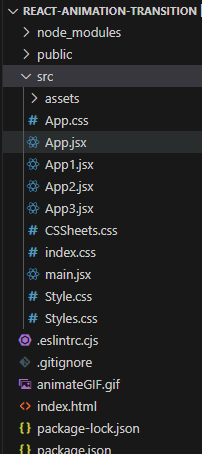
The updated dependencies in the package.json file
"dependencies": {
"@chakra-ui/react": "^2.8.2",
"@emotion/react": "^11.11.1",
"@emotion/styled": "^11.11.0",
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"framer-motion": "^6.5.1",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
},
Example 1: Write the code in the respective files. Conditionally apply CSS classes to elements based on state changes. This allows you to trigger animations using CSS transitions or keyframes.
The animation can be seen with the change in the color of the div text content. Initially the div content is visible but it is hidden after the button is clicked by the user.
CSS
/* App.css */
.btn-container {
display: flex;
margin: 1rem;
flex-direction: row;
}
.btn {
padding: 5px;
margin: 20px;
cursor: pointer;
background-color: #FFFF;
border-radius: 10px;
font-weight: bold;
}
/* The animation rule */
@keyframes myKeyframe {
0% {
background-color: red;
}
25% {
background-color: yellow;
}
50% {
background-color: blue;
}
100% {
background-color: green;
}
}
/* The above rule is applied to this element*/
.animate {
width: 200px;
height: 200px;
background-color: red;
animation-name: myKeyframe;
animation-duration: 4s;
}
JavaScript
// App.js
import {
ChakraProvider,
Text, Box, Flex,
} from "@chakra-ui/react";
import { useState } from 'react'
import './App.css'
function App() {
const [visible, setVisible] = useState(true);
function hide() {
setVisible(false);
}
if (!visible) {
style.display = "none";
}
return (
<ChakraProvider>
<Box bg="lightgrey" w="100%" h="100%" p={4}>
<Text
color="#2F8D46" fontSize="2rem"
textAlign="center" fontWeight="400"
my="1rem"
>
GeeksforGeeks - React JS concepts
</Text>
<h3><b>React hooks with animation</b></h3>
<br />
<div className="animate">
<div>A computer science portal for geeks designed
for who wish to get hands-on Data Science.
Learn to apply DS methods and techniques,
and acquire analytical skills.</div>
<button className="btn btn-container"
onClick={hide}>
Click this to hide
<i className="" />{" "}
</button>
</div>
</Box>
</ChakraProvider>
);
};
export default App
Start your application using the following command:
npm start
Output:

Example 2: Write the codes in the respective files. As the text Shadow is animated using the CSS keyframe rules, the count is incremented to demonstrate the useState and useEffect React hooks.
CSS
/* Styles.css */
h2 {
font-size: 36px;
font-weight: bold;
animation: textShadow 1s ease-in-out infinite alternate;
}
@keyframes textShadow {
from {
text-shadow: 2px 2px #333;
}
to {
text-shadow: 10px 10px #333;
}
}
JavaScript
// App.js
import {
ChakraProvider,
Text, Box,
} from "@chakra-ui/react";
import React, { useState, useEffect } from 'react'
import './Styles.css'
function App() {
const [count, setCount] = useState(0);
useEffect(() => {
//Implementing the setInterval method
const interval = setInterval(() => {
setCount(count + 1);
}, 1000);
//Clearing the interval
return () => clearInterval(interval);
}, [count]);
return (
<ChakraProvider>
<Box bg="lightgrey" w="100%" h="100%" p={4}>
<h1 style={{ color: "green" }}>
GeeksforGeeks
</h1>
<Text
color="#2F8D46" fontSize="2rem"
textAlign="center" fontWeight="400"
my="1rem"
>
React hooks with animation
</Text>
<h2>Animated text Shadow</h2>
<h1>{count}</h1>
</Box>
</ChakraProvider>
);
};
export default App
Output:
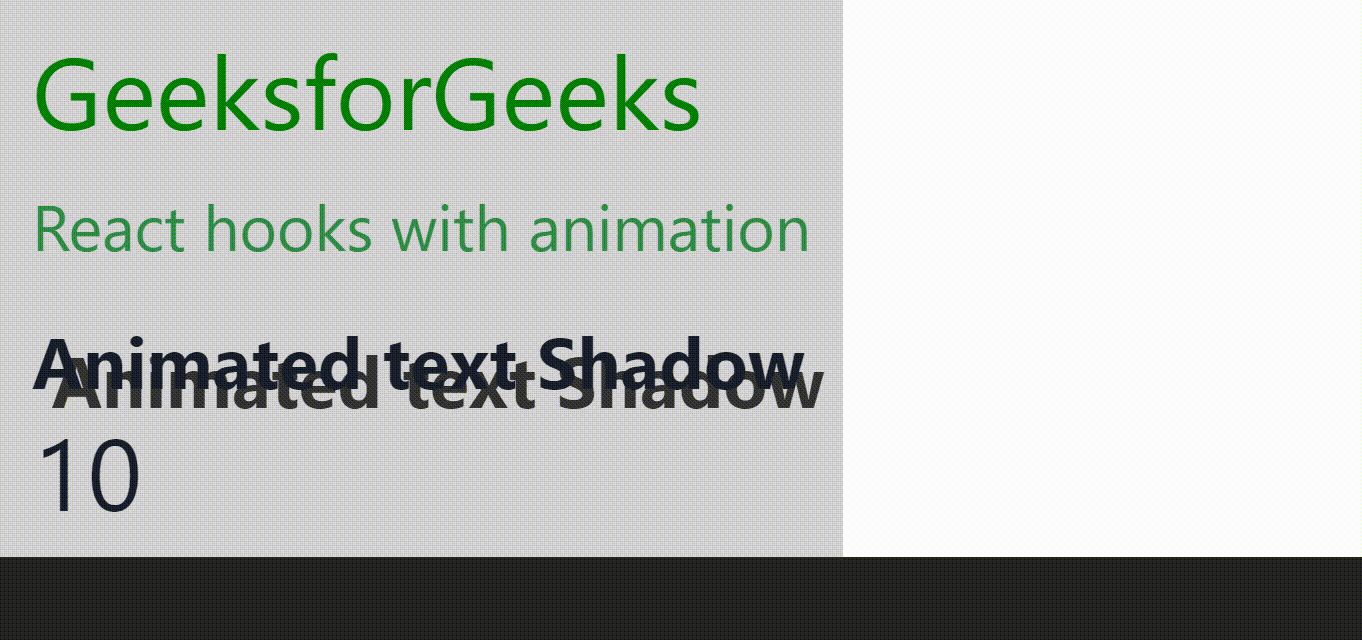
Example 3: The following code demonstrates gradient animation and displaying message using the useEffect React hook. The setTimeout
 function in JavaScript is a method used to introduce a delay before executing a specified function.
CSS
/* CSSheets.css */
h2 {
font-size: 36px;
font-weight: bold;
animation: gradientText 5s ease-in-out infinite;
}
@keyframes gradientText {
0% {
color: red;
}
25% {
color: yellow;
}
50% {
color: blue;
}
100% {
color: green;
}
}
JavaScript
// App.js
import {
ChakraProvider,
Text, Box,
} from "@chakra-ui/react";
import React, { useState, useEffect } from 'react'
import './CSSheets.css'
function App() {
const [message, setMessage] = useState('');
useEffect(() => {
// setTimeout method to update the message
// after 3000 milliseconds or 3 seconds
const timeoutId = setTimeout(() => {
setMessage('Message delayed after 3 seconds!');
}, 3000);
// Clear the timeout after component unmounting
return () => clearTimeout(timeoutId);
}, []);
return (
<ChakraProvider>
<Box bg="lightgrey" w="100%" h="100%" p={4}>
<h1 style={{ color: "green" }}>
GeeksforGeeks
</h1>
<Text
color="#2F8D46" fontSize="2rem"
textAlign="center" fontWeight="400"
my="1rem"
>
React hooks with animation
</Text>
<h2>Animated Gradient text </h2>
<h1>{message}</h1>
</Box>
</ChakraProvider>
);
};
export default App
Output:
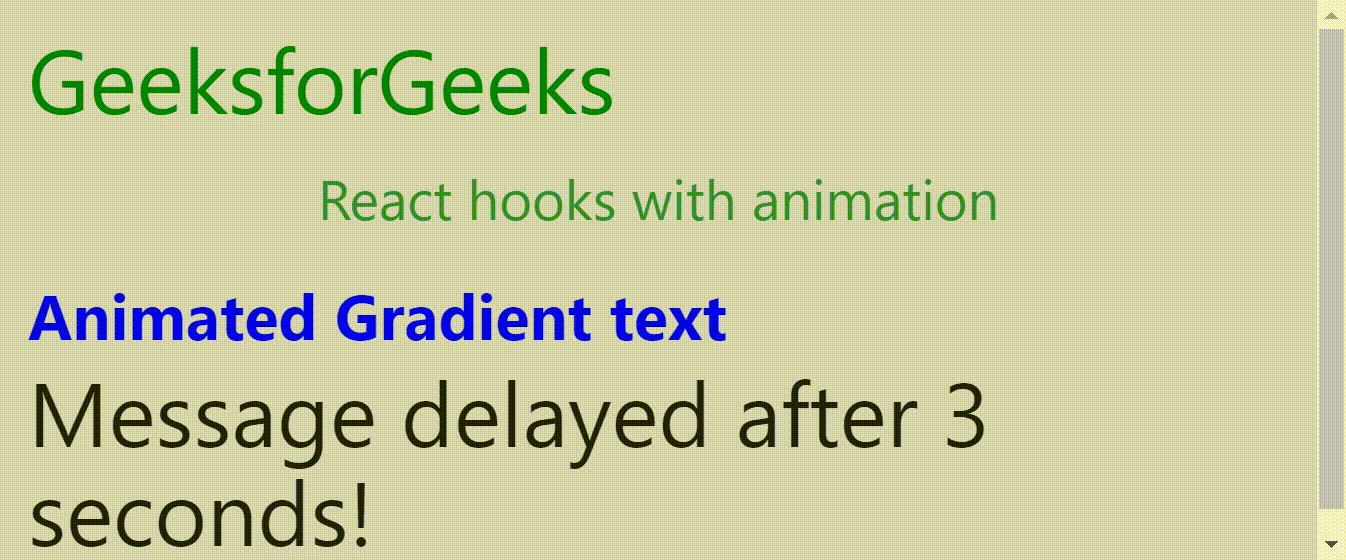
Example 4: CSS transitions are a simple way to add animations to elements. In this example, CSS transition properties (like transition-property
, transition-duration
, transition-timing-function
, and transition-delay
) are defined in your CSS to smoothly animate changes.
CSS
/* Style.css */
div {
width: 400px;
height: 320px;
transition: width 2s ease-in .2s;
display: inline-block;
}
div:hover {
width: 600px;
}
JavaScript
// App.js
import {
ChakraProvider,
Text, Box,
} from "@chakra-ui/react";
import React, { useState, useEffect } from 'react'
import './Style.css'
function App() {
const [count, setCount] = useState(0);
useEffect(() => {
setTimeout(() => {
setCount((count) => count + 1);
}, 1000);
});
return (
<ChakraProvider>
<Box bg="lightgrey" w="100%" h="100%" p={4}>
<h1 style={{ color: "green" }}>
GeeksforGeeks
</h1>
<Text
color="#2F8D46" fontSize="2rem"
textAlign="center" fontWeight="400"
my="1rem"
>
React hooks with transition
</Text>
<h2><b>Transition Property </b></h2>
<div>
<p>transition-property: width</p>
<p>transition-duration: 5s</p>
<p>transition-timing-function: ease-in</p>
<p>transition-delay: .2s</p>
<p><b>We have rendered {count} times</b></p>
</div>
</Box>
</ChakraProvider>
);
};
export default App
Output:
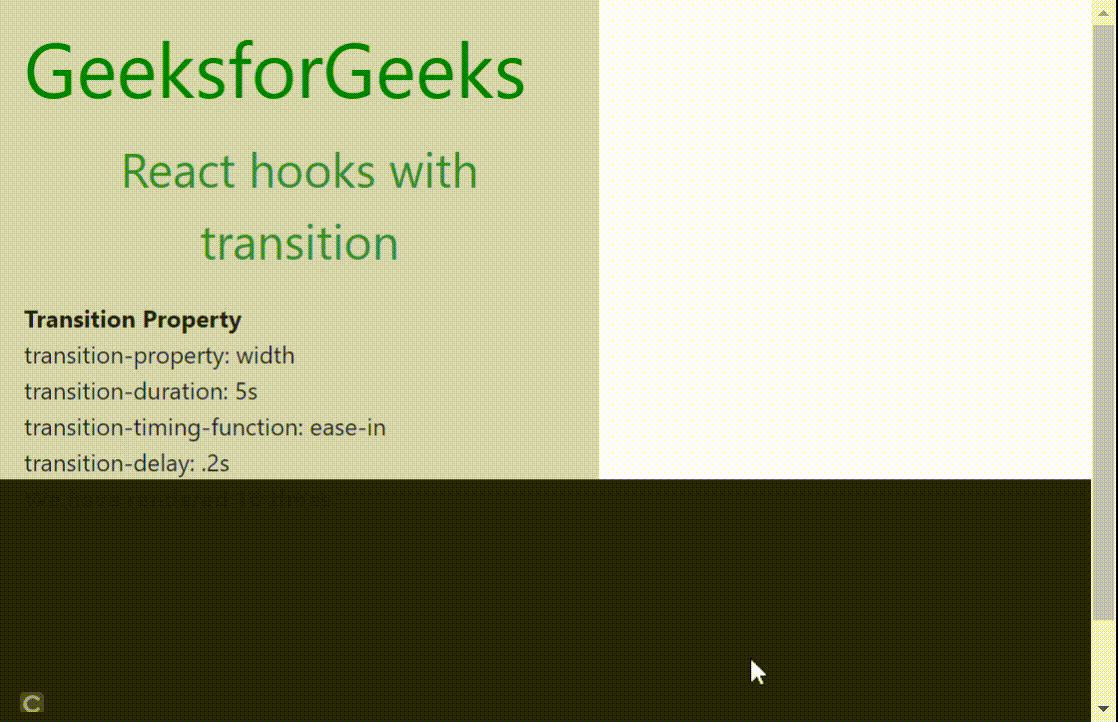
Share your thoughts in the comments
Please Login to comment...