Why useLayoutEffect Hook is beneficial in React ?
Last Updated :
23 Feb, 2024
useLayoutEffect is a React Hook that is similar to useEffect, but it fires synchronously after all DOM mutations. It’s typically used for imperative DOM mutations or measurements, where you need to be sure that the DOM is updated before continuing. Here’s an example of how you might use useLayoutEffect
Prerequisites:
Steps to Create Application:
Step 1: Initialize a new React project using Create React App.
npx create-react-app uselayouteffect
cd uselayouteffect
Example to implement useLayoutEffect: In this example there is an implementation of useLayoutEffect which handles the changing of background color while clicking anywhere on the screen.
Javascript
import React from 'react' ;
import DynamicBackgroundColor from './DynamicBackgroundColor' ;
function App() {
return (
<div className= "App" >
<DynamicBackgroundColor />
</div>
);
}
export default App;
|
Javascript
import React, { useState, useLayoutEffect } from 'react' ;
const DynamicBackgroundColor = () => {
const [color, setColor] = useState( 'white' );
useLayoutEffect(() => {
const updateColor = () => {
const randomColor =
'#' + Math.floor(Math.random() * 16777215).toString(16);
setColor(randomColor);
};
updateColor();
window.addEventListener( 'click' , updateColor);
return () => {
window.removeEventListener( 'click' , updateColor);
};
}, []);
return (
<div style={{ backgroundColor: color, minHeight: '100vh' , display: 'flex'
, justifyContent: 'center' , alignItems: 'center' }}>
<h1>Click Anywhere to Change Background Color</h1>
</div>
);
};
export default DynamicBackgroundColor;
|
To start the application run the following command.
npm start
Output:
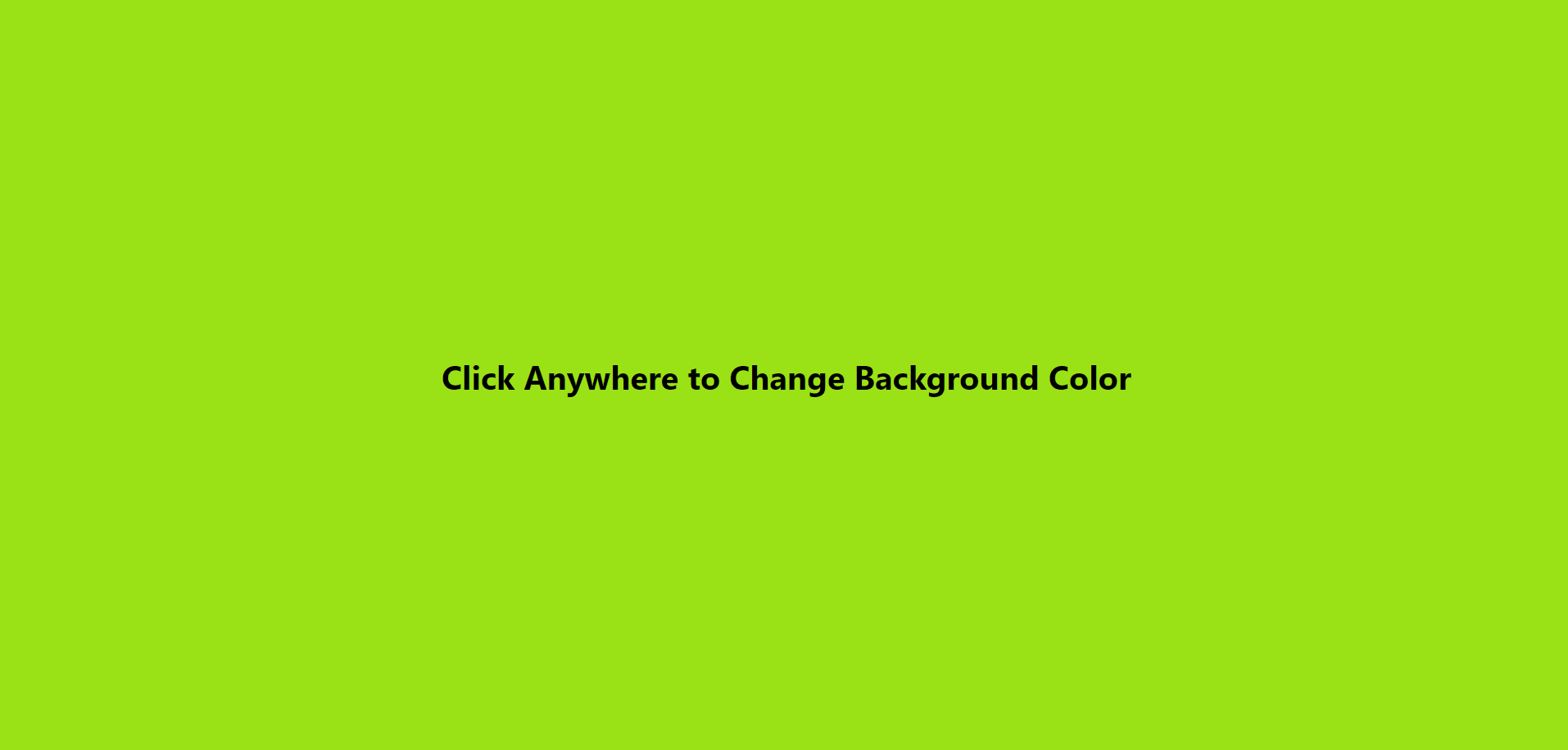
Share your thoughts in the comments
Please Login to comment...