What is React Router?
Last Updated :
29 Feb, 2024
React Router is like a traffic controller for your React application. Just like how a traffic controller directs vehicles on roads, React Router directs users to different parts of your app based on the URL they visit. So, when you click on a link or type a URL in your browser, React Router decides which part of your React app to show, making it easy to create seamless and interactive single-page applications.
Key Features of React Router:
- Declarative Routing: Set up navigation using a declarative syntax, making it easy to define routes clearly and intuitively.
- Component-Based: Routes are associated with React components, allowing each route to render a specific component when navigated.
- Nested Routing: Supports nested routes, enabling the creation of complex page structures with subcomponents and layouts.
- Dynamic Routing: Allows for dynamic route parameters, enabling the creation of flexible and parameterized routes.
- Programmatic Navigation: Enables navigation via JavaScript code, facilitating redirections and transitions based on user interactions or application logic.
Steps to Create React App and Installing React Router:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. folder name, move to it using the following command:
cd foldername
Step 3: Install React Router package.
npm install react-router-dom
Project Structure:
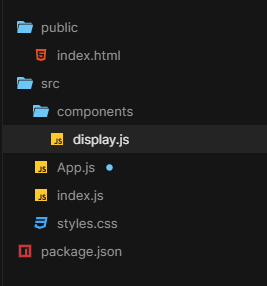
Example Code: Write the following code in the respective files.
- App.js: This file implements Routing
- Home.js: This file contains the Home Component used in Routing
- About.js: This file contains the About Component used in Routing
Javascript
import React from 'react' ;
import {
BrowserRouter as Router,
Routes,
Route,
Link
} from 'react-router-dom' ;
import Home from './Home' ;
import About from './About' ;
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to= "/" >Home</Link>
</li>
<li>
<Link to= "/about" >About</Link>
</li>
</ul>
</nav>
<Routes>
<Route path= "/" element={<Home />} />
<Route path= "/about" element={<About />} />
</Routes>
</div>
</Router>
);
}
export default App;
|
Javascript
import React from 'react'
const Home = () => {
return (
<div>Home</div>
)
}
export default Home
|
Javascript
import React from 'react'
const About = () => {
return (
<div>About</div>
)
}
export default About
|
Start your application using the following command.
npm start
Output:
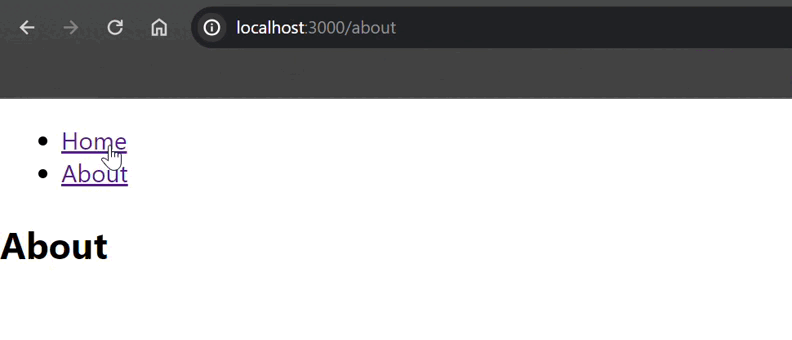
Routing to Different Components
Share your thoughts in the comments
Please Login to comment...