What are the pros & cons of EJS for Node.js Templating ?
Last Updated :
15 Apr, 2024
NodeJS, an influential JavaScript runtime, is becoming increasingly popular for the development of scalable, fast server-side applications. EJS (Embedded JavaScript) is among the leading templating engines for NodeJS. Here we shall discuss some merits and demerits of using this template engine in your work.
What is EJS?
EJS stands for Embedded Javascript. It helps to make dynamic pages. It has a simple syntax to show dynamic content. We can easily use Javascript methods like map, and forEach to render the data.
Syntax:
- To show the dynamic value
<h1> <%= title %> </h1>
- To render Javascript methods and logic.
<% for(let i = 0; i < arr.length; i++) { %>
<%= arr[i] %>
<% } %>
Pros of EJS:
1. Ease of Integration:
- EJS is easy to integrate within 2-3 lines we can easily integrate nodejs with ejs.
- render allows to render EJS efficiently.
- Also if any user familiar with JS already, then it is also easy to use EJS.
// index.js
app.use(express.static(path.join(__dirname, 'public')))
.set('views', path.join(__dirname, 'views'))
.set('view engine', 'ejs');
app.get('/', (req, res) => {
res.render('index',{title:"Geeks"});
});
2. Dynamic Content:
- By integrating Javascript capaabilities, it helps to make dynamic web pages.
- It helps of render any value, any array data from API’s.
- By the use of Dynamic content, most of the times no need to make any other static HTML page for that data.
const authors = [
{
id:1,
name:"Akash"
},
{
id:2,
name:"Aman"
},
{
id:3,
name:"John"
}
]
app.get('/', (req, res) => {
res.render('index',{authors});
});
// index.ejs
<body>
<% for (let i = 0; i< authors.length;i++) { %>
<p><%= authors[i].name %></p>
<% } %>
</body>
Output:
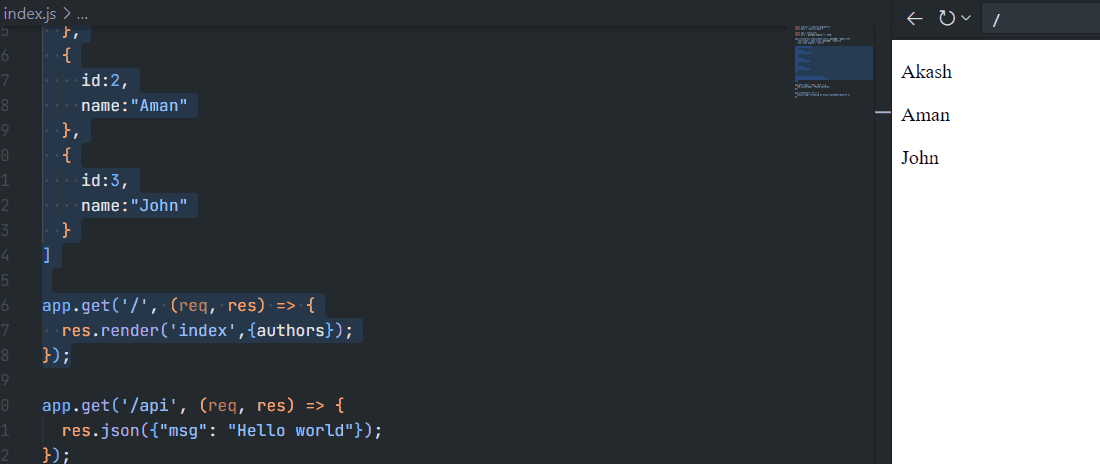
Output
3. Active Community Support:
There is an active community around EJS that ensures continual development, debugging, and overall bettering. This means that this can be relied upon when building projects where support-driven updates and improvements are a precondition.
You can checkout more about this here.
4. Composability and Template Inheritence:
- This feature allows developers to develop complex applications from using EJS
- This helps developers to develop complex applications in small parts.
- It integrate dynamicity along with composability.
HTML
<!-- home.ejs -->
<%- include('header',{title:"Home"}) %>
<%- include('layout', {content: 'Welcome to the Home Page!'}) %>
<%- include('footer') %>
HTML
<!-- header.ejs -->
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title><%= title %></title>
</head>
HTML
<!-- footer.ejs -->
<footer>
<p>© 2024 My Website</p>
</footer>
HTML
<!-- layout.ejs -->
<body>
<header>
<h1>Welcome to My Website</h1>
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<p><%= content %></p>
</main>
</body>
JavaScript
// server.js
const express = require('express');
const ejs = require('ejs');
const path = require('path');
const app = express();
// Set EJS as the view engine
app.set('view engine', 'ejs');
// Set views directory
app.set('views', path.join(__dirname, 'views'));
// Define a route to render the EJS template
app.get('/', (req, res) => {
// Pass data to the template
const data = {
pageTitle: 'My EJS App'
};
res.render('index', data);
});
// Start the server
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Output:
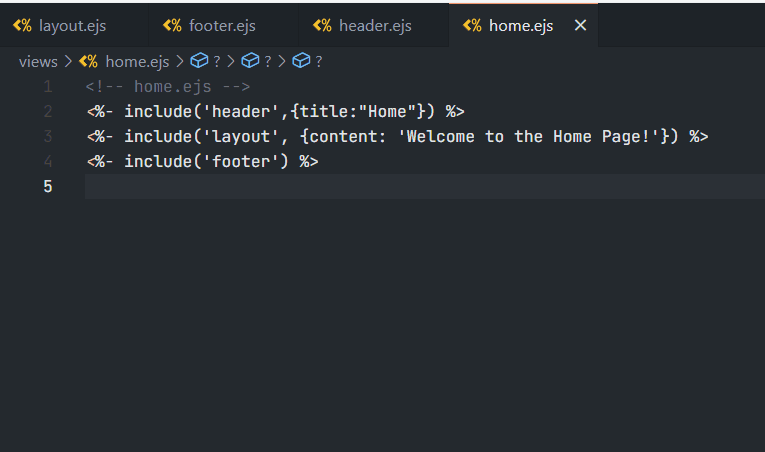
Output
Cons of EJS:
1. Mixing Logic with Presentation:
However, it is worth noting that though JavaScript code can be embedded in templates, this can have its negative impact. This is because mixing logic with presentation in the same file may lead to code that is more difficult to read and maintain especially in larger projects.
<!-- Mixing logic with presentation example -->
<% if (user.isAdmin) { %>
<p>Welcome, Admin!</p>
<% } else { %>
<p>Welcome, User!</p>
<% } %>
2. Limited Features Compared to Other Engines:
EJS provides basic functionality for templating and it might not have all the advanced features like some other template engines do. Developers who need more complicated templating jobs may find EJS less convenient compared with other more feature-rich alternatives.
3. Performance Concerns:
Also, there are cases where EJS might suffer performance issues particularly when dealing with many templates or complex logic inside templates. With strict performance requirements for a given project, developers have to take into account performance implications and explore alternatives.
Conclusion:
Embedded JavaScript (EJS) remains a popular choice for templating in Node.js due to its ease of integration, dynamic content capabilities, and active community support. However, developers should weigh these aspects against each other before settling for EJS as their Node.js development platform considering factors such as code maintainability, feature requirements and performance considerations.
Share your thoughts in the comments
Please Login to comment...