Passing an object to Client in Node/Express.js with EJS Templating Engine
Last Updated :
02 Apr, 2024
EJS stands for Embedded JavaScript. It is a templating language used to generate dynamic HTML pages with data from the server by embedding JavaScript code.
This article provides a detailed explanation of the process of passing objects from a Node.js/Express server to clients using the EJS templating engine. It guides us through setting up a basic Express application with EJS, demonstrating how to efficiently transmit data objects for dynamic content rendering on the client side. With practical examples and best practices, we can enhance our Node.js applications by delivering personalized content easily.
Features of EJS:
- Dynamic Content: Based on data from servers or other sources, we can generate a dynamic HTML template making it more interactive and personalized.
- Efficiency: Passing objects to the client reduces the need for additional HTTP requests to fetch data from the server. Instead of making separate requests for each piece of data, we can bundle related data into a single object and send it to the client in the initial response as an EJS parameter.
- Reduced Server Load: By letting data processing to the client side, we can reduce the server’s workload and improve performance, especially for applications with a large number of concurrent users.
- Control Structures: We can use loops, conditions, and iterations over an array/object to build complex templates.
- Embedded JavaScript : Using special tags ( <% %>, <%= %>, and <%- %> ) , we can embed javascript in html markup. EJS replaces <%= %> tags, with the actual value of the variable when rendering the page.
- State Management: Objects passed to the client can contain state information, allowing us to maintain the application’s state across multiple requests. This is particularly useful for implementing features like user sessions, shopping carts, or form data persistence.
Steps to Create Node App & Install Required Modules:
Step 1: Firstly, we will make the folder named root by using the below command in the VScode Terminal. After creation use the cd command to navigate to the newly created folder.
mkdir root
cd root
Step 2: Once the folder is been created, we will initialize NPM using the below command, this will give us the package.json file.
npm init -y
Step 3: Once the project is been initialized, we need to install Express and EJS dependencies in our project by using the below installation command of NPM.
npm i express ejs
Project Structure:
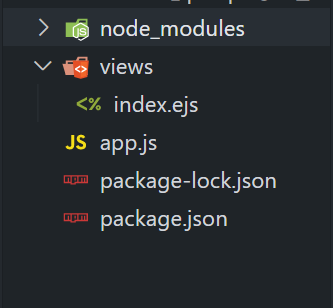
The updated depedencies in package.json file will look like:
"dependencies": {
"express": "^4.17.1",
"ejs": "^3.1.6"
}
Step 3 : Add the following code in the entry point file (here app.js) of node/express project .
HTML
<!DOCTYPE html>
<html>
<head>
<title>Passing object in EJS template</title>
</head>
<body>
<h2>Welcome To <%=data.name%></h2>
<h3><%=data.description%></h3>
<h3>Founded by <%=data.founder%></h3>
</body>
</html>
JavaScript
const express = require('express')
const app = express();
// Set EJS as templating engine
app.set('view engine', 'ejs');
const port = 3000
app.get('/', (req, res) => {
// Sample data object
const data = {
name: 'GeeksforGeeks',
description: "One stop platform for developers",
founder: "Sandeep Jain"
};
});
// Render the 'index.ejs' template and pass the data object
res.render('index', { data });
app.listen(port, () => {
console.log(`Example app listening on port ${port}`)
})
Start your server using the following command:
node app.js
Output:
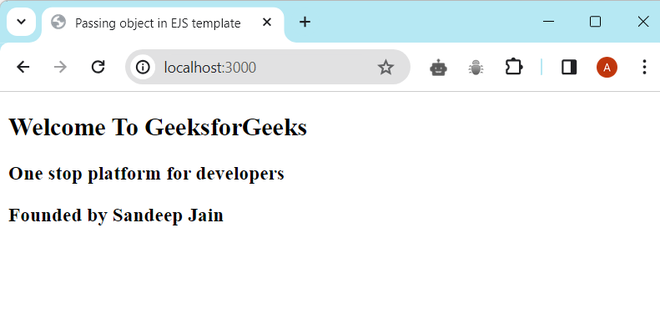
Share your thoughts in the comments
Please Login to comment...