Pagination using Node, Mongo, Express.js and EJS
Last Updated :
27 Mar, 2024
In this article, we will dive into the process of implementing pagination in our web application using NodeJS, MongoDB, Express, and EJS for dynamic HTML rendering. Additionally, we’ll enhance the visual appeal of our pagination controls with CSS. Our focus will be on creating a user profile system where users can create a new profile and scrolling beyond a specified limit prompts navigation options like ‘Next’ and ‘Previous’ to seamlessly explore more users.
Output Preview: Let’s take a look at how our final project will appear
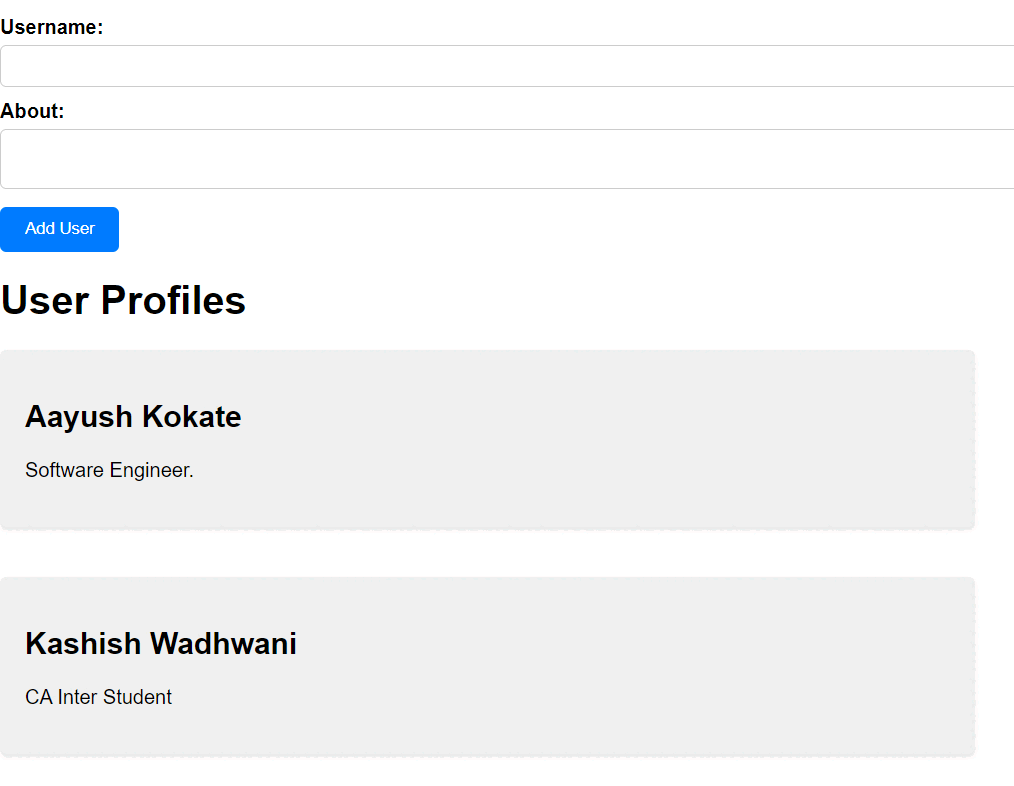
What is Pagination?
Pagination is a crucial feature in web development allowing us to split large sets of data into smaller, more manageable chunks for display. In our project, we’ll be implementing pagination to enable users to navigate through a long list of user profiles. By breaking these profiles into multiple pages, we’ll not only enhance the user experience by making content easier to digest but also improve the performance of our web application by reducing the amount of data loaded at once.
Steps to Implement Pagination:
Step 1: Set up your project.
mkdir project-name
cd project-name
npm init
Step 2: Install necessary packages using the following command.
npm install express mongoose ejs path dotenv body-parser
Project Structure:
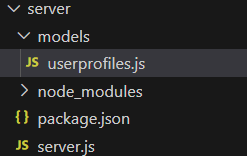
Project Structure
The updated dependencies in package.json file :
"dependencies": {
"dotenv": "^16.4.5",
"body-parser": "^1.20.2",
"ejs": "^3.1.9",
"express": "^4.18.3",
"mongoose": "^8.2.2",
"path": "^0.12.7"
}
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>User Profiles</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
position: relative;
min-height: 100vh;
}
.user-list {
display: flex;
flex-wrap: wrap;
gap: 20px;
}
.user-card {
background-color: #f0f0f0;
padding: 20px;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
width: calc(50% - 20px);
margin-bottom: 20px;
}
.pagination {
margin-top: 20px;
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
display: flex;
justify-content: center;
align-items: center;
}
.pagination a {
margin-right: 10px;
text-decoration: none;
color: blue;
padding: 8px 16px;
border-radius: 5px;
background-color: #f0f0f0;
transition: background-color 0.3s ease;
}
.pagination a:hover {
background-color: #ddd;
}
form {
margin-top: 20px;
}
label {
display: block;
margin-bottom: 5px;
font-weight: bold;
}
input[type="text"],
textarea {
width: 100%;
padding: 8px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
button[type="submit"] {
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
button[type="submit"]:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<form action="/mynetwork" method="POST">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="about">About:</label>
<textarea id="about" name="about" required></textarea>
<button type="submit">Add User</button>
</form>
<h1>User Profiles</h1>
<div class="user-list">
<% users.forEach(user => { %>
<div class="user-card">
<h2><%= user.username %></h2>
<p><%= user.about %></p>
</div>
<% }) %>
</div>
<div class="pagination">
<% if (page > 1) { %>
<a href="/mynetwork?page=<%= page - 1 %>&perPage=<%= perPage %>">Previous</a>
<% } %>
<% if (users.length === perPage) { %>
<a href="/mynetwork?page=<%= page + 1 %>&perPage=<%= perPage %>">Next</a>
<% } %>
</div>
</body>
</html>
JavaScript
const mongoose = require("mongoose");
const profileSchema = new mongoose.Schema({
username: {
type: String,
required: true
},
about: {
type: String
}
}, { collection: 'profileSchema' });
const user = mongoose.model('user', profileSchema);
module.exports = user;
JavaScript
require("dotenv").config()
const express = require("express");
const path = require("path");
const mongoose = require("mongoose");
const bodyParser = require('body-parser');
const User = require('./models/userprofiles');
const app = express();
const PORT = process.env.PORT || 3000
const URI = process.env.MONGODB_URI;
app.set('view engine', 'ejs');
app.set('views', path.join(__dirname, 'views'));
app.use(express.json());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
mongoose.connect(URI);
const database = mongoose.connection;
database.on('err', (err) => {
console.log(err);
});
database.once('connected', () => {
console.log('Database connected to server');
})
app.route('/mynetwork')
.get(async (req, res) => {
try {
const page = parseInt(req.query.page) || 1;
const perPage = parseInt(req.query.perPage) || 7;
const skip = (page - 1) * perPage;
const users = await User.find()
.skip(skip)
.limit(perPage)
.exec();
res.render('index', {
users: users,
page: page,
perPage: perPage });
} catch (error) {
console.error(error);
res.status(500).json({ message: 'Server Error' });
}
})
.post(async (req, res) => {
try {
const { username, about } = req.body;
const newUser = new User({ username, about });
await newUser.save();
res.redirect('/mynetwork');
} catch (error) {
console.error(error);
res.status(500).json({ message: 'Server Error' });
}
});
app.listen(PORT, () => {
console.log(`Server is listening on port ${PORT}`)
});
Steps to Run Application:
Step 1: Run the application using the following command from the root directory of the project:
npm start
Step 2: Type the following link in your browser
http://localhost:3000/mynetwork
Output:
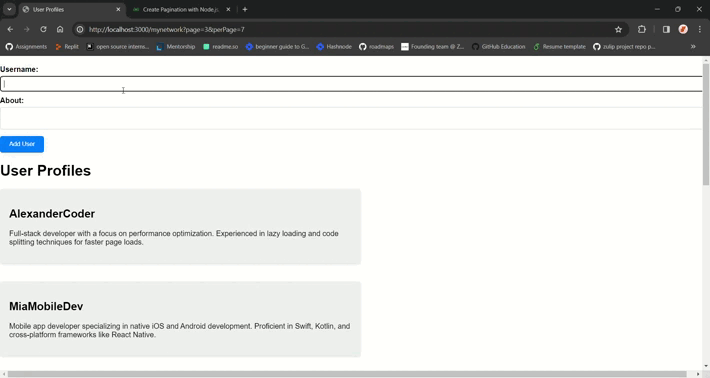
Share your thoughts in the comments
Please Login to comment...