Tornado- HTTP servers and clients
Last Updated :
20 Mar, 2024
Tornado is a Python web framework and a library for async networks. It is meant for non-blocking, highly effective apps. Tornado has become popular because it can handle large numbers of simultaneous connections easily. In this article, we will explain Tornado HTTP servers and clients.
What are HTTP servers and clients?
- HTTP Server: An HTTP server is a software application that runs on a computer and listens for incoming HTTP requests from clients. When it receives a request, it processes the request and sends back an appropriate response. Examples of HTTP servers include Apache HTTP Server, Nginx, Microsoft Internet Information Services (IIS), etc.
- HTTP Client: An HTTP client is a software application or a tool that accepts HTTP requests to servers to retrieve web resources such as HTML pages, images, videos, etc. Web browsers such as Google Chrome, Mozilla Firefox, Safari, etc., act as HTTP clients when users access web pages.
Tornado- HTTP servers and clients Examples
Below, are the examples of Tornado- HTTP servers and clients in Python.
Tornado – HTTP Server Example
In this example, below code sets up a Tornado web server with two handlers: MainHandler for root URL (“/”) returns “Hello, Tornado!” as text, and ApiHandler for “/api” returns a JSON response {“message”: “Hello, Tornado!”, “status”: “success”}. The server listens on port 8001 and starts running.
Python
import tornado.ioloop
import tornado.web
import json
class MainHandler(tornado.web.RequestHandler):
def get(self):
self.write("Hello, Tornado!")
class ApiHandler(tornado.web.RequestHandler):
def get(self):
data = {"message": "Hello, Tornado!", "status": "success"}
self.set_header("Content-Type", "application/json")
self.write(json.dumps(data))
def make_app():
return tornado.web.Application([
(r"/", MainHandler),
(r"/api", ApiHandler),
])
if __name__ == "__main__":
app = make_app()
app.listen(8001)
print("Tornado server is running at http://localhost:8001")
tornado.ioloop.IOLoop.current().start()
Output:
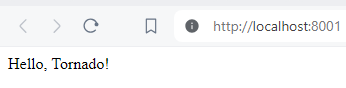
tornado server
Tornado – HTTP Client Example
In this example of a Tornado’s HTTP client that can make an asynchronous HTTP request. The function fetches data from a URL asynchronously and prints the response body. To run asynchronous code synchronously within the Tornado event loop, one can use run_sync method.
Python
import tornado.httpclient
async def fetch_data():
http_client = tornado.httpclient.AsyncHTTPClient()
response = await http_client.fetch("http://localhost:8001/api")
print(response.body)
if __name__ == "__main__":
tornado.ioloop.IOLoop.current().run_sync(fetch_data)
Output :
b'{"message": "Hello, Tornado!", "status": "success"}'
Another Example
Here, we will create a form submission and successfully message flashing web app using Tornado – HTTP servers and clients.
File Strcuture :
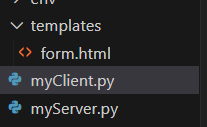
myServer.py : below, code sets up a Tornado web server with a single request handler, MainHandler. When a GET request is made to the root URL (“/”), it renders a form HTML page. When a POST request is made, it retrieves the submitted form data (name and email), validates if both are provided, and responds accordingly.
Python3
import tornado.ioloop
import tornado.web
class MainHandler(tornado.web.RequestHandler):
def get(self):
self.render("form.html")
def post(self):
name = self.get_body_argument("name")
email = self.get_body_argument("email")
if name and email:
self.write(f"Form Successfully submitted! Name: {name} Email: {email}")
else:
self.write("Please fill the name and email.")
def make_app():
return tornado.web.Application([
(r"/", MainHandler),
], template_path="templates")
if __name__ == "__main__":
app = make_app()
print("Tornado server is running at http://localhost:8003")
app.listen(8003)
tornado.ioloop.IOLoop.current().start()
myClient.py : Below, code imports the requests library to send an HTTP POST request to “http://localhost:8003” with JSON data containing a name and email. It then prints the response text returned from the server after the POST request.
Python3
import requests
url = "http://localhost:8003"
data = {
"name": "Abul Hasan",
"email": "abulhax@gmail.com"
}
response = requests.post(url, data=data)
print(response.text)
Creating GUI
templates/form.html : Below HTML code defines a simple form with fields for entering a name and an email address. When submitted, the form sends a POST request to the root URL (“/”) of a server.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Tornado Form</title>
</head>
<body>
<form action="/" method="post">
<label>Name: <input type="text" name="name"></label><br>
<label>Email: <input type="email" name="email"></label><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Output:
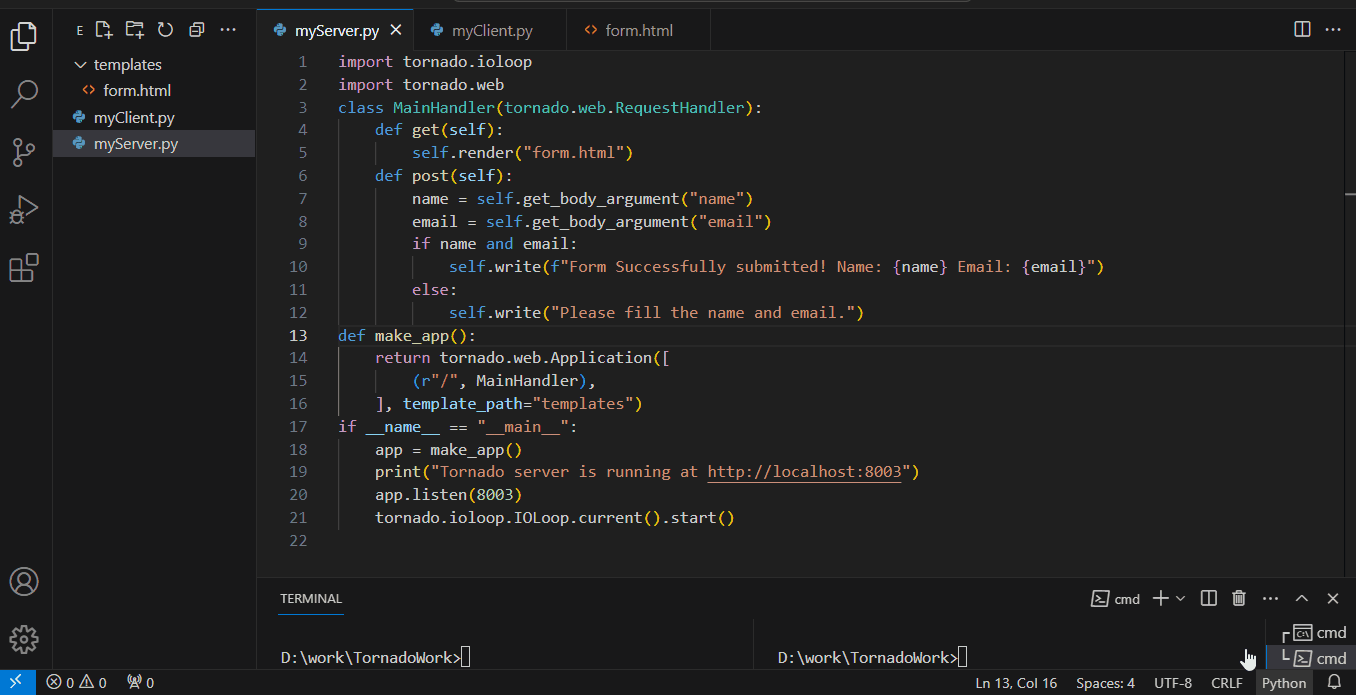
Output demo
Conclusion
In conclusion, Tornado provides a powerful solution for developing high-performance web applications and APIs. It has an asynchronous framework and simple request handling that allow developers to build scalable servers with high efficiency capable of handling many simultaneous connections. Moreover, Tornado can also easily work together with JSON serialization; hence creating a platform where data is communicated swiftly between clients and servers.
Share your thoughts in the comments
Please Login to comment...