Differences between node.js and Tornado
Last Updated :
17 Oct, 2023
Node.js and Tornado are both popular choices for building scalable and high-performance web applications and services. However, they are based on different programming languages (JavaScript and Python, respectively) and have distinct design philosophies and features. In this guide, we will explore the key differences between Node.js and Tornado, explaining various concepts related to each technology, and providing examples where relevant.
Key Features of Node.js
Node.js is an open-source, JavaScript runtime built on Chrome’s V8 JavaScript engine. It has gained immense popularity due to its non-blocking, event-driven architecture, making it an excellent choice for building real-time applications. Some of its key features include:
- Non-blocking I/O: Node.js is designed to handle concurrent connections efficiently, making it suitable for applications that require high concurrency, such as chat applications and online gaming.
- NPM (Node Package Manager): Node.js has a vast ecosystem of libraries and modules available through NPM, which simplifies the process of adding functionality to your applications.
- Cross-platform: Node.js can run on various operating systems, providing flexibility in deployment.
- Single-threaded with event loop: Node.js utilizes a single-threaded event loop, which allows it to handle multiple requests concurrently without creating separate threads for each request.
Key Features of Tornado
Tornado, on the other hand, is a Python web framework known for its asynchronous networking capabilities. It’s particularly well-suited for building web applications that require long-lived network connections. Some key features of Tornado include:
- Asynchronous support: Tornado uses the async/await syntax in Python, enabling developers to write non-blocking, asynchronous code easily.
- WebSockets: Tornado has built-in support for WebSockets, making it an excellent choice for real-time web applications.
- High-performance: Tornado is known for its speed and can handle a large number of concurrent connections efficiently.
- Built-in security features: Tornado provides features for handling security aspects like cross-site scripting (XSS) and cross-site request forgery (CSRF) protection.
Differences between Node.js and Tornado
Programming Language
|
Node.js is based on JavaScript, which is a widely-used, single-threaded, event-driven language for both client-side and server-side development.
|
Tornado is built on Python, a versatile and widely-adopted language known for its readability and ease of use.
|
Concurrency Model
|
Node.js uses a single-threaded, event-driven, and non-blocking I/O model. It relies on an event loop that allows it to efficiently handle a large number of connections simultaneously without creating separate threads or processes.
|
Tornado also uses a single-threaded, event-driven architecture but includes support for coroutines. Tornado’s coroutines enable developers to write asynchronous code more explicitly and manage concurrency effectively.
|
Node.js is famous for its single-threaded event loop, which efficiently handles multiple concurrent connections. It uses callbacks and Promises to manage asynchronous operations.
|
Tornado uses an asynchronous event-driven architecture that relies on Python’s ‘asyncio’ framework, allowing developers to write non-blocking code using the ‘async/await’ syntax.
|
Node.js is a also a popular choice for real-time applications, and it offers real-time capabilities through libraries like Socket.io.
|
Tornado is particularly well-suited for real-time web applications due to its built-in support for WebSockets and long-lived connections.
|
Performance
|
Node.js is known for its exceptional performance when handling I/O-bound operations, making it well-suited for applications requiring real-time communication like chat applications or streaming services.
|
Tornado also offers high performance but may require a deeper understanding of Python’s concurrency model, such as the use of coroutines and asynchronous programming techniques.
|
Community and Ecosystem
|
Node.js has a vast and active community, along with a rich ecosystem of packages and modules available through the npm (Node Package Manager). This makes it easy to find solutions and third-party libraries for various tasks.
|
Tornado has a smaller but dedicated community. It may not have as extensive an ecosystem as Node.js, but it benefits from Python’s broader ecosystem, which includes numerous libraries for various purposes.
|
Use Cases
|
Node.js is often preferred for building real-time applications, web APIs, microservices, and applications that require high concurrency and low-latency communication.
|
Tornado is suitable for building web servers, long-polling systems, and web applications that require asynchronous I/O, such as chat servers and social media platforms.
|
Examples: Simple Web Server using Node.js
In these examples, we’ve demonstrated how to create simple web servers using both Node.js and Tornado. These servers respond with a “Hello, [Framework]” message when accessed in a web browser. The differences in code structure and syntax between Node.js and Tornado are evident.
In this example, we created a basic HTTP web server using the built-in ‘http’ module. Here’s a step-by-step explanation:
- We import the ‘http’ module, which is part of the Node.js standard library, to access the necessary functionality for creating an HTTP server.
- We create an HTTP server by calling http.createServer(). This function takes a callback function that will be executed every time a client makes a request to the server.
- Inside the callback function, we configure the server’s response. We use res.writeHead() to set the HTTP response header with a status code of 200 (indicating a successful response) and the content type as ‘text/plain’.
- We send the response content (‘Hello, Node.js!’) using res.end(), which will be displayed in the client’s browser when they access the server.
- Finally, we start the server by calling server.listen() on port 3000. When the server is successfully started, a message is logged to the console.
Javascript
const http = require( 'http' );
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type' : 'text/plain' });
res.end( 'Hello, Node.js!' );
});
server.listen(3000, () => {
console.log( 'Node.js server is running on port 3000' );
});
|
Output
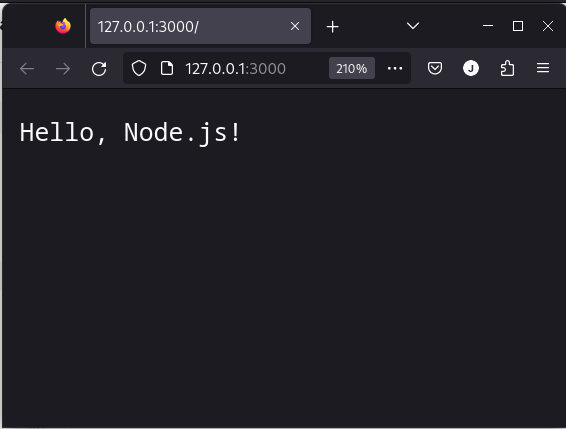
Example: Simple Web Server using Tornado(Python)
In this Tornado example, we create a simple web server using the Tornado web framework, a popular choice for building asynchronous web applications in Python. Here’s a step-by-step explanation:
- We import the required modules from the Tornado library: tornado.ioloop and tornado.web. These modules provide the necessary functionality for setting up a Tornado web application and handling incoming HTTP requests.
- We define a class called MainHandler that inherits from tornado.web.RequestHandler. This class will handle incoming HTTP GET requests.
- Inside the MainHandler class, we define a get() method. This method is called when a GET request is made to the root path (‘/’). In this method, we use self.write() to send the response content (‘Hello, Tornado!’) back to the client.
- We define a function called make_app() that creates a Tornado web application instance. We specify the URL pattern that maps requests to the MainHandler class when the root path (‘/’) is accessed.
- In the if __name__ == “__main__”: block, we create an instance of the Tornado application using make_app().
- We start the Tornado server by calling app.listen() on port 8888.
- Finally, we start the Tornado I/O loop using tornado.ioloop.IOLoop.current().start(). This I/O loop handles incoming requests and manages asynchronous operations within the Tornado application
Python3
import tornado.ioloop
import tornado.web
class MainHandler(tornado.web.RequestHandler):
def get( self ):
self .write( "Hello, Tornado!" )
def make_app():
return tornado.web.Application([(r "/" , MainHandler)])
if __name__ = = "__main__" :
app = make_app()
app.listen( 8888 )
tornado.ioloop.IOLoop.current().start()
|
Output
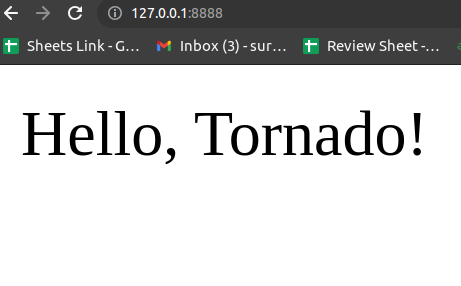
Conclusion :
Node.js and Tornado are both excellent frameworks, but their suitability depends on the specific requirements of your web application. Node.js shines in I/O-bound tasks and real-time applications, while Tornado excels in handling long-lived connections and asynchronous programming. Consider your project’s needs and choose the framework that best aligns with your goals.
Share your thoughts in the comments
Please Login to comment...