Python Tornado Utilities
Last Updated :
15 Mar, 2024
Tornado-Utilities takes center stage, providing an arsenal of tools and features within the Tornado web framework. This article delves into the syntax-centric aspects of Tornado-Utilities, elucidating how these tools facilitate a smoother and more efficient web development process.
Tornado Utilities Syntax in Python
Tornado-Utilities comprises diverse modules and classes, each designed with a specific purpose to simplify common development tasks. Let’s explore the key functionalities through a syntax-centric lens.
Tornado.autoreload Syntax
The autoreload module streamlines development by automatically detecting code changes, initiated through the start()
function. This feature eliminates the need for manual reloading, enhancing the development experience.
import tornado.autoreload
tornado.autoreload.start()
Tornado.concurrent Syntax
Tornado-Utilities empowers asynchronous programming through the concurrent module, featuring the Future
class. Developers can efficiently manage asynchronous computations using this syntax, tapping into Tornado’s full potential.
from tornado.concurrent import Future
# Asynchronous programming with Future objects
future = Future()
Tornado.log Syntax
Logging support is paramount, and Tornado-Utilities delivers with the tornado.log
module. This syntax allows for specialized logging tailored for Tornado applications, ensuring a comprehensive view of the application’s behavior.
import tornado.log
# Specialized logging for Tornado applications
tornado.log.app_log.info(“Application log message”)
Tornado.options Syntax
Managing command-line options and configurations is simplified through the define()
and parse_command_line()
functions. Developers can effortlessly handle command-line arguments and configuration files using this syntax.
from tornado.options import define, options
# Command-line parsing and configuration options
define(“port”, default=8888, help=”run on the given port”, type=int)
options.parse_command_line()
Tornado.testing Syntax
Tornado-Utilities supports unit testing for asynchronous code. Developers can create test cases and runners tailored for Tornado’s asynchronous programming model, ensuring the reliability of their code.
import tornado.testing
# Asynchronous code unit testing
class MyAsyncTestCase(tornado.testing.AsyncTestCase):
async def test_something_asynchronous(self):
# Test logic here
Tornado Utilities in Python
Below are some of the examples of Tornado Utilities in Python:
- Automatic Code Reloading
- Command-Line Options
- Logging Support
Automatic Code Reloading
In this example, in below code Tornado web app, set to run on port 8888, employs automatic code reloading. The `MainHandler` responds to GET requests with a greeting. With `tornado.autoreload`, changes in “app.py” trigger automatic server refresh, streamlining development.
Python3
import tornado.ioloop
import tornado.web
from tornado.autoreload import start, watch
class MainHandler(tornado.web.RequestHandler):
def get(self):
self.write("Hello, Tornado with Automatic Code Reloading!")
def make_app():
return tornado.web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
app = make_app()
app.listen(8888)
# Start monitoring for code changes
watch("app.py")
# Start the Tornado I/O loop with automatic reloading enabled
start()
tornado.ioloop.IOLoop.current().start()
Output
Command-Line Options
In this example, below Tornado code defines a simple web application with command-line options. It utilizes Tornado’s `options` module to declare and parse options like the server port and debug mode. The main handler responds with a greeting message. The script allows customization of options via the command line and starts the Tornado server.
Python3
import tornado.ioloop
import tornado.web
from tornado.options import define, options, parse_command_line
# Define options
define("port", default=8888, help="run on the given port", type=int)
define("debug", default=False, help="enable debug mode", type=bool)
class MainHandler(tornado.web.RequestHandler):
def get(self):
self.write("Hello, Tornado with Options!")
def make_app():
return tornado.web.Application([
(r"/", MainHandler),
], debug=options.debug)
if __name__ == "__main__":
# Parse command-line options
parse_command_line()
# Create the Tornado application
app = make_app()
# Start the Tornado server
app.listen(options.port)
print(f"Server started on port {options.port}")
# Start the I/O loop
tornado.ioloop.IOLoop.current().start()
Output
Logging Support
In this example, This Tornado web application, set to run on port 8888, includes a `MainHandler` responding to GET requests with a greeting. The `make_app` function configures the application, and `enable_pretty_logging()` enhances log output in the terminal. The server startup is logged, and the Tornado I/O loop initiates for seamless execution.
Python3
import logging
import tornado.ioloop
import tornado.web
from tornado.log import enable_pretty_logging
class MainHandler(tornado.web.RequestHandler):
def get(self):
self.write("Hello, Tornado with Logging Support!")
def make_app():
return tornado.web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
app = make_app()
app.listen(8888)
# Enable colored log output in the terminal
enable_pretty_logging()
logging.info("Tornado server starting on port 8888...")
tornado.ioloop.IOLoop.current().start()
Output
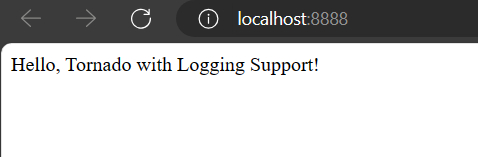
Share your thoughts in the comments
Please Login to comment...