Network Programming Python – HTTP Clients
Last Updated :
25 Oct, 2020
The request from the client in HTTP protocol reaches the server and fetches some data assuming it to be a valid request. This response from the server can be analyzed by using various methods provided by the requests module. Some of the ways below provide information about the response sent from the server to the Python program running on the client-side :
Getting initial Response
The get() method is used to get the basic information of the resources used at the server-side. This function fetches the data from the server and returns as a response object which can be printed in a simple text format.
Python3
import requests
print (req.text[: 2000 ])
|
Output:
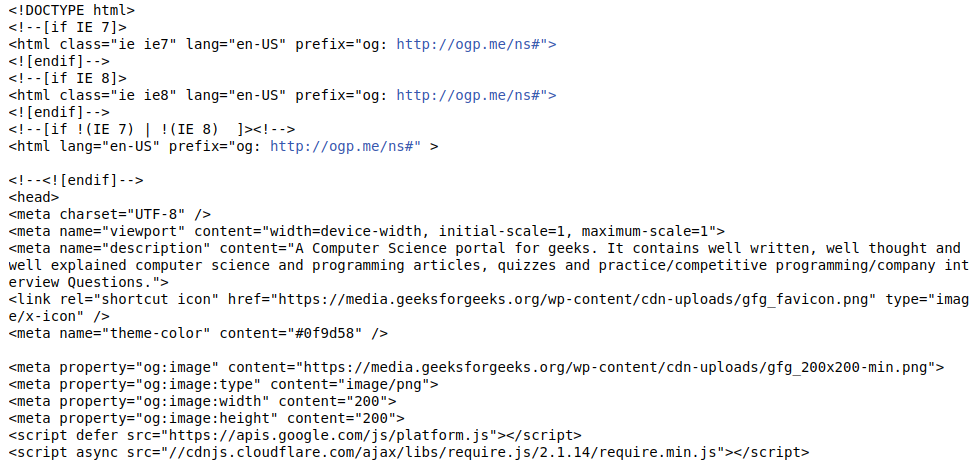
Getting session-info
The session() method returns the session object which provides certain parameters to manipulate the requests. It can also manipulate cookies for all the requests initiated from the session object. If a large amount of requests are made to a single host then, the associated TCP connection is recalled.
Python3
import requests
s = requests.Session()
print (r.text)
|
Output:
{
"cookies": {
"sessioncookie": "419735271"
}
}
Error Handling
If some error is raised in processing the request to the server, the exception is raised by the program which can be handled using the timeout attribute, which defines the time value until the program waits and after that, it raises the timeout error.
Python3
import requests
try :
except requests.exceptions.RequestException as e:
raise SystemExit(e)
|
Output:
HTTPSConnectionPool(host=’www.geeksforgeeks.org’, port=443): Max retries exceeded with url: / (Caused by ConnectTimeoutError(<urllib3.connection.HTTPSConnection object at 0x0000018CFDAD83C8>, ‘Connection to www.geeksforgeeks.org timed out. (connect timeout=1e-06)’))
Share your thoughts in the comments
Please Login to comment...