How to Stop Tornado Web Server
Last Updated :
31 Jan, 2024
Tornado is a powerful and scalable web framework for Python that is known for its non-blocking network I/O, making it an excellent choice for building web applications and APIs. When it comes to stopping the Tornado web server, it’s essential to do it gracefully to ensure that ongoing connections are handled properly. In this article, we’ll explore methods of demonstrating how to stop the Tornado web server.
Proper way to stop a Tornado
Below are the two ways to stop the Tornado web server in Python.
Stop Tornado Web Server Using Keyboard Interrupt
In this example, the below code sets up a basic Tornado web server responding with “Hello, Tornado!” at the root path. It defines a handler and an application, then starts the server on port 8889. The server runs until manually interrupted (e.g., by pressing `Ctrl+C`), at which point it prints a shutdown message and gracefully stops the Tornado event loop.
Python3
import tornado.ioloop
import tornado.web
class MainHandler(tornado.web.RequestHandler):
def get( self ):
self .write( "Hello, Tornado!" )
def make_app():
return tornado.web.Application([
(r "/" , MainHandler),
])
if __name__ = = "__main__" :
app = make_app()
app.listen( 8889 )
try :
tornado.ioloop.IOLoop.current().start()
except KeyboardInterrupt:
print ( "Shutting down gracefully..." )
finally :
tornado.ioloop.IOLoop.current().stop()
|
Output:
Tornado web server started at http://localhost:8889
Shutting down gracefully...
When we press ctrl+c it’s stop the server.
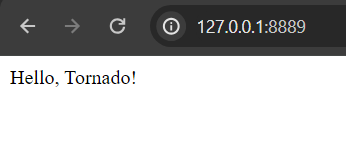
Stop Tornado Web Server Using Sleep Timer
In this example, below code creates a Tornado web server with a “Hello, Tornado!” response at the root path (“/”). The server listens on port 8889 and automatically stops after 5 seconds, thanks to the scheduled execution of the `stop_server` function using Tornado’s `IOLoop`. The `stop_server` function prints a shutdown message and stops the event loop, ensuring a graceful server shutdown.
Python3
import tornado.ioloop
import tornado.web
import time
class MainHandler(tornado.web.RequestHandler):
def get( self ):
self .write( "Hello, Tornado!" )
def stop_server():
print ( "Stopping Tornado web server..." )
tornado.ioloop.IOLoop.current().stop()
def main():
application = tornado.web.Application([
(r '/' , MainHandler),
])
port = 8889
application.listen(port)
tornado.ioloop.IOLoop.current().add_timeout(time.time() + 5 , stop_server)
tornado.ioloop.IOLoop.current().start()
if __name__ = = "__main__" :
main()
|
Output :
Tornado web server started at http://localhost:8889
Stopping Tornado web server...
After 5 sec. Tornado server automatically stopped.
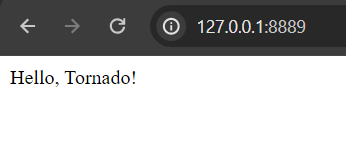
Share your thoughts in the comments
Please Login to comment...