Python tornado.httputil.HTTPServerRequest() Examples
Last Updated :
27 Feb, 2024
Python’s Tornado is a powerful web framework known for its non-blocking I/O and high performance. One crucial component of Tornado is the HTTPServerRequest class, which represents an incoming HTTP request. In this article, we’ll delve into the tornado.httputil.HTTPServerRequest class and explore practical examples to better understand its usage in Python.
What is tornado.httputil.HTTPServerRequest Class?
The tornado.httputil.HTTPServerRequest class in the Tornado web framework represents an incoming HTTP request. It provides access to various aspects of the request, such as headers, query parameters, cookies, and request body. Here’s a brief overview of the HTTPServerRequest class along with its commonly used methods and attributes:
Syntax:
class tornado.httputil.HTTPServerRequest(method, uri, version, headers=None, body=None, host=None, files=None, connection=None)
Parameters:
- method (str): HTTP method (e.g., “GET”, “POST”).
- uri (str): Request URI.
- version (str): HTTP version (e.g., “HTTP/1.1”).
- headers (tornado.httputil.HTTPHeaders, optional): Request headers.
Python tornado.httputil.HTTPServerRequest() Examples
below, are the Python tornado.httputil.HTTPServerRequest() Examples in Python.
Example 1: Tornado Web Server with Query Parameters
In this example, The Tornado web server, listening on port 8888, responds to a GET request at the root (“/”). The `MainHandler` class extracts “name” and “age” query parameters from the URL, dynamically generates a greeting, and displays it on the webpage. The server is initiated using the `make_app` function, and the `IOLoop` ensures continuous listening for incoming requests.
Python3
import tornado.ioloop
import tornado.web
from tornado.httputil import HTTPServerRequest
class MainHandler(tornado.web.RequestHandler):
def get( self ):
name = self .get_query_argument( "name" )
age = self .get_query_argument( "age" )
self .write(f "Hello, {name}! Age: {age}" )
def make_app():
return tornado.web.Application([
(r "/" , MainHandler),
])
if __name__ = = "__main__" :
app = make_app()
app.listen( 8888 )
tornado.ioloop.IOLoop.current().start()
|
Run the Server
python script_name.py
Output
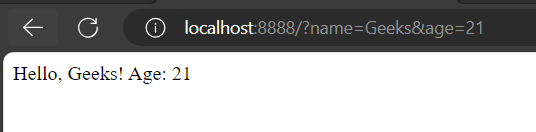
Example 2: Tornado Server Handling POST Requests
app.py : In this example, Tornado web server, running on port 8888, defines a single route (“/post“) handled by the PostHandler class. When a POST request is made, the server decodes the request body, removing any “data=” prefix, and responds by acknowledging the received data. The make_app function creates the Tornado web application, and the server is initiated to listen for incoming requests.
Python3
import tornado.ioloop
import tornado.web
from tornado.httputil import HTTPServerRequest
class PostHandler(tornado.web.RequestHandler):
def post( self ):
data = self .request.body.decode( "utf-8" )
if data.startswith( "data=" ):
data = data[ len ( "data=" ):]
self .write(f "Received POST data: {data}" )
def make_app():
return tornado.web.Application([
(r "/post" , PostHandler),
])
if __name__ = = "__main__" :
app = make_app()
app.listen( 8888 )
tornado.ioloop.IOLoop.current().start()
|
form.html : HTML code defines a simple form with a POST method, sending data to “http://localhost:8888/post” when submitted. The form includes a text input labeled “Data” with an initial value of “example data.” Upon submission, the entered data is sent to the specified server endpoint for processing.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Test POST Request</ title >
</ head >
< body >
< label for = "data" >Data:</ label >
< input type = "text" id = "data" name = "data" value = "example data" >
< br >
< br >
< input type = "submit" value = "Submit" >
</ form >
</ body >
</ html >
|
Run the Server
python script_name.py
Output:
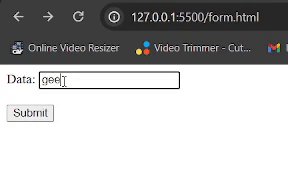
Example 3: Tornado Server for Display Upload Images
app.py : In this example, The Tornado web server, running on port 8888, defines a single route (“/upload”) handled by the UploadHandler class. When a POST request with a file upload is made, the server sets the appropriate image Content-Type header and directly writes the binary image data to the response. If no file is uploaded, a message indicating the absence of an uploaded file is displayed.
Python3
import tornado.ioloop
import tornado.web
from tornado.httputil import HTTPServerRequest
class UploadHandler(tornado.web.RequestHandler):
def post( self ):
file_info = self .request.files.get( "file_upload" )
if file_info:
file_content = file_info[ 0 ][ "body" ]
file_name = file_info[ 0 ][ "filename" ]
self .set_header( "Content-Type" , "image/jpeg" )
self .write(file_content)
else :
self .write( "No file uploaded." )
def make_app():
return tornado.web.Application([
(r "/upload" , UploadHandler),
])
if __name__ = = "__main__" :
app = make_app()
app.listen( 8888 )
tornado.ioloop.IOLoop.current().start()
|
upload.html : HTML code creates a file upload form with a title “File Upload Form.” The form, with enctype=”multipart/form-data”, allows users to select a file using the “Select File” input. Upon submission, the selected file is sent to the server at “http://localhost:8888/upload” using the POST method.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta http-equiv = "X-UA-Compatible" content = "IE=edge" >
< meta name = "viewport" content = "width=device-width, initial-scale=1.0" >
< title >File Upload Form</ title >
</ head >
< body >
< h2 >File Upload Form</ h2 >
< label for = "file_upload" >Select File:</ label >
< input type = "file" name = "file_upload" id = "file_upload" required>
< br >
< input type = "submit" value = "Upload" >
</ form >
</ body >
</ html >
|
Run the Server
python script_name.py
Output:
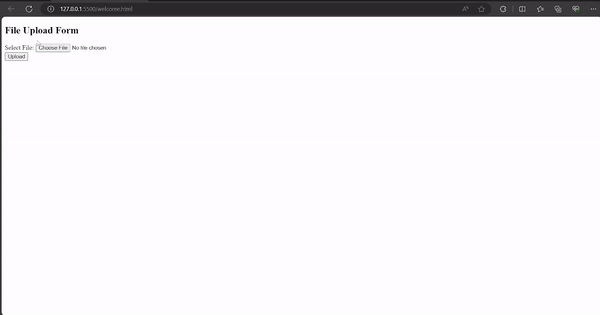
Conclusion
In conclusion, the tornado.httputil.HTTPServerRequest class in Tornado proves essential for handling incoming HTTP requests in Python web development. Through examples, we explored its versatility in managing query parameters, processing POST requests, handling cookies, and facilitating file uploads. Leveraging this class empowers developers to build responsive and high-performance web applications with Tornado’s non-blocking I/O.
Share your thoughts in the comments
Please Login to comment...