TCS Coding Practice Question | Check Palindrome Number
Last Updated :
22 Mar, 2024
Given a number, the task is to check if this number is Palindrome or not using
Command Line Arguments
.
Examples:
Input: 123
Output: No
Input: 585
Output: Yes
Approach:
- Since the number is entered as Command line Argument, there is no need for a dedicated input line
- Extract the input number from the command line argument
- This extracted number will be in String type.
- Convert this number into integer type and store it in a variable, say num
- Find the reverse of this number and store in a variable, say rev_num
- Check if this rev_num and num are same or not.
- If they are not same, the number is not Palindrome
- If they are same, the number is a Palindrome
Program:
C
// C program to check if a number is Palindrome
// using command line arguments
#include <stdio.h>
#include <stdlib.h> /* atoi */
// Function to reverse the number
int reverseNumber(int num)
{
// Variable to store the
// resultant reverse number
int rev_num = 0;
// Traverse through the number digit by digit
while (num > 0) {
// Append the last digit of num
// as the next digit of rev_num
rev_num = rev_num * 10 + num % 10;
// Remove the last digit from the num
num = num / 10;
}
// Return the reversed number
return rev_num;
}
// Function to reverse a string
int isPalindrome(int num)
{
int rev_num = reverseNumber(num);
if (num == rev_num)
return 1;
else
return 0;
}
// Driver code
int main(int argc, char* argv[])
{
int num, res = 0;
// Check if the length of args array is 1
if (argc == 1)
printf("No command line arguments found.\n");
else {
// Get the command line argument and
// Convert it from string type to integer type
// using function "atoi( argument)"
num = atoi(argv[1]);
// Check if it is Palindrome
res = isPalindrome(num);
// Check if res is 0 or 1
if (res == 0)
// Print No
printf("No\n");
else
// Print Yes
printf("Yes\n");
}
return 0;
}
Java
// Java program to check if a number is Palindrome
// using command line arguments
class GFG {
// Function to reverse the number
public static int reverseNumber(int num)
{
// Variable to store the
// resultant reverse number
int rev_num = 0;
// Traverse through the number digit by digit
while (num > 0) {
// Append the last digit of num
// as the next digit of rev_num
rev_num = rev_num * 10 + num % 10;
// Remove the last digit from the num
num = num / 10;
}
// Return the reversed number
return rev_num;
}
// Function to reverse a string
public static int isPalindrome(int num)
{
int rev_num = reverseNumber(num);
if (num == rev_num)
return 1;
else
return 0;
}
// Driver code
public static void main(String[] args)
{
// Check if length of args array is
// greater than 0
if (args.length > 0) {
// Get the command line argument and
// Convert it from string type to integer type
int num = Integer.parseInt(args[0]);
// Get the command line argument
// and check if it is Palindrome
int res = isPalindrome(num);
// Check if res is 0 or 1
if (res == 0)
// Print No
System.out.println("No\n");
else
// Print Yes
System.out.println("Yes\n");
}
else
System.out.println("No command line "
+ "arguments found.");
}
}
Python3
import sys
def is_palindrome(number):
# Convert the number to a string
number_str = str(number)
# Check if the number is the same forwards and backwards
return number_str == number_str[::-1]
if __name__ == "__main__":
# Check if length of argv is not equal to 2
if len(sys.argv) != 2:
print("Usage: python palindrome_checker.py <number>")
sys.exit(1)
# Get the command line argument and
# Convert it from string type to integer type
# using function "int(sys.argv[1])"
number = int(sys.argv[1])
if is_palindrome(number):
# print if number is palindrome
print(number, "is a palindrome.")
else:
# print if numner is not a palindrome
print(number, "is not a palindrome.")
Output:
- In C:
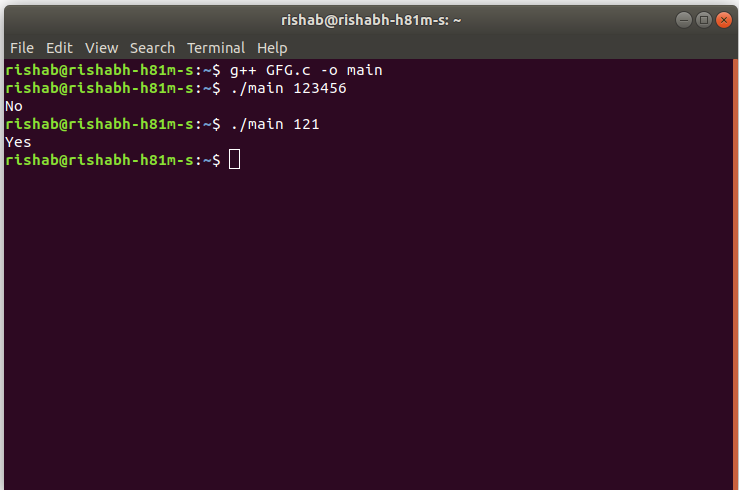
- In Java :
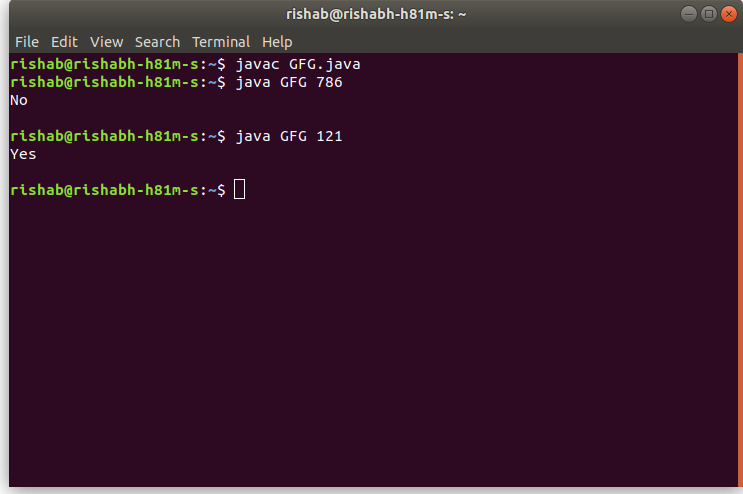
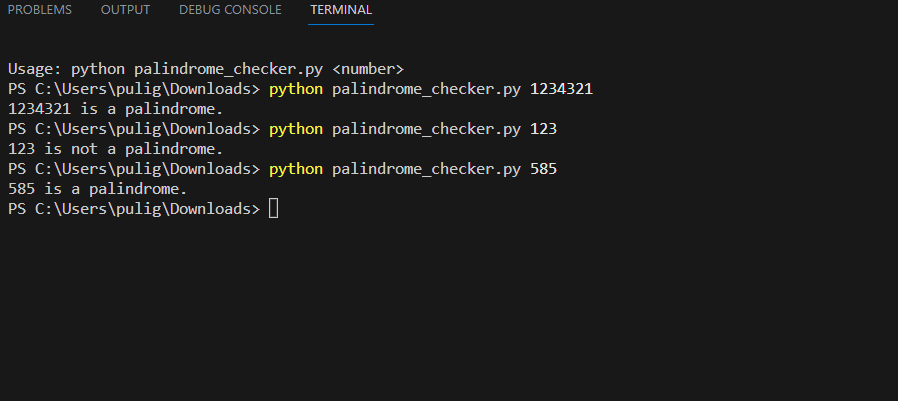
Share your thoughts in the comments
Please Login to comment...