TCS Coding Practice Question | Check Odd or Even
Last Updated :
06 Apr, 2024
Given a number, the task is to check if this number is Odd or Even using Command Line Arguments. A number is called even if the number is divisible by 2 and is called odd if it is not divisible by 2.
Â
Examples:
Input: 123
Output: No
Input: 588
Output: Yes
Approach:
- Since the number is entered as Command line Argument, there is no need for a dedicated input line
- Extract the input number from the command line argument
- This extracted number will be in string type.
- Convert this number into integer type and store it in a variable, say num
- Check if this number is completely divided by 2
- If completely divisible, the number is Even
- If not completely divisible, the number is Odd
Program:Â
C++
#include <iostream>
#include <cstdlib> // For atoi function
// Function to check if a number is even or odd
int isEvenOrOdd(int num) {
return (num % 2);
}
// Driver code
int main(int argc, char* argv[]) {
int num, res = 0;
// Check if the length of args array is 1
if (argc == 1)
std::cout << "No command line arguments found." << std::endl;
else {
// Convert the command line argument to an integer
num = std::atoi(argv[1]);
// Check if it is even or odd
res = isEvenOrOdd(num);
// Check if res is 0 or 1
if (res == 0)
std::cout << "Even" << std::endl;
else
std::cout << "Odd" << std::endl;
}
return 0;
}
// code contributed by Oprix
C
// C program to check
// if a number is even or odd
// using command line arguments
#include <stdio.h>
#include <stdlib.h> /* atoi */
// Function to the check Even or Odd
int isEvenOrOdd(int num)
{
return (num % 2);
}
// Driver code
int main(int argc, char* argv[])
{
int num, res = 0;
// Check if the length of args array is 1
if (argc == 1)
printf("No command line arguments found.\n");
else {
// Get the command line argument and
// Convert it from string type to integer type
// using function "atoi( argument)"
num = atoi(argv[1]);
// Check if it is even or odd
res = isEvenOrOdd(num);
// Check if res is 0 or 1
if (res == 0)
// Print Even
printf("Even\n");
else
// Print Odd
printf("Odd\n");
}
return 0;
}
Java
// Java program to check
// if a number is even or odd
// using command line arguments
class GFG {
// Function to the check Even or Odd
public static int isEvenOrOdd(int num)
{
return (num % 2);
}
// Driver code
public static void main(String[] args)
{
// Check if length of args array is
// greater than 0
if (args.length > 0) {
// Get the command line argument and
// Convert it from string type to integer type
int num = Integer.parseInt(args[0]);
// Get the command line argument
// and check if it is even or odd
int res = isEvenOrOdd(num);
// Check if res is 0 or 1
if (res == 0)
// Print Even
System.out.println("Even\n");
else
// Print Odd
System.out.println("Odd\n");
}
else
System.out.println("No command line "
+ "arguments found.");
}
}
Python
import sys
# Function to check if a number is even or odd
def is_even_or_odd(num):
return num % 2
# Driver code
if len(sys.argv) == 1:
print("No command line arguments found.")
else:
num = int(sys.argv[1])
res = is_even_or_odd(num)
if res == 0:
print("Even")
else:
print("Odd")
# contributed by oprix
JavaScript
// Function to check if a number is even or odd
function isEvenOrOdd(num) {
return num % 2;
}
// Driver code
if (process.argv.length === 2) {
console.log("No command line arguments found.");
} else {
const num = parseInt(process.argv[2]);
const res = isEvenOrOdd(num);
if (res === 0) {
console.log("Even");
} else {
console.log("Odd");
}
}
// code contributed by Oprix
Output:
- In C:
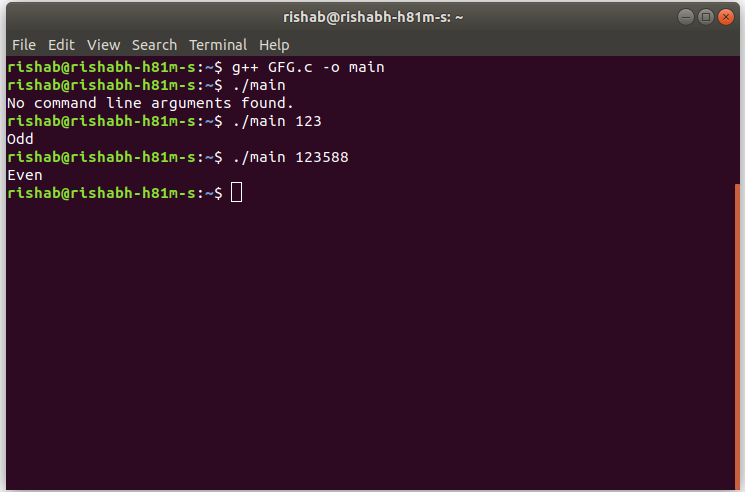
- In Java:
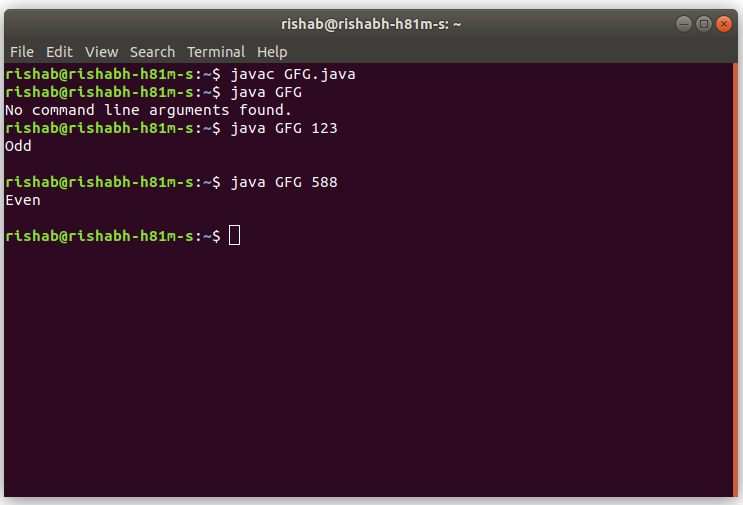
Time Complexity: O(1)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...