TCS Coding Practice Question | Factorial of a Number
Last Updated :
15 Sep, 2023
Given a number, the task is to find the Factorial of this number using Command Line Arguments. Factorial of a non-negative integer is the multiplication of all integers smaller than or equal to n.
Examples:
Input: 3
Output: 6
1 * 2 * 3 = 6
Input: 6
Output: 720
1 * 2 * 3 * 4 * 5 * 6 = 720
Approach:
- Since the number is entered as Command line Argument, there is no need for a dedicated input line
- Extract the input number from the command line argument
- This extracted number will be in String type.
- Convert this number into integer type and store it in a variable, say num
- Find the reverse of this number and store in a variable, say rev_num
- Check if this rev_num and num are same or not.
- If they are not same, the number is not Palindrome
- If they are same, the number is a Palindrome
Program:
C
#include <stdio.h>
#include <stdlib.h> /* atoi */
unsigned int factorial(unsigned int n)
{
int res = 1, i;
for (i = 2; i <= n; i++)
res *= i;
return res;
}
int main( int argc, char * argv[])
{
int num, res = 0;
if (argc == 1)
printf ( "No command line arguments found.\n" );
else {
num = atoi (argv[1]);
printf ( "%d\n" , factorial(num));
}
return 0;
}
|
Java
class GFG {
static int factorial( int n)
{
int res = 1 , i;
for (i = 2 ; i <= n; i++)
res *= i;
return res;
}
public static void main(String[] args)
{
if (args.length > 0 ) {
int num = Integer.parseInt(args[ 0 ]);
System.out.println(factorial(num));
}
else
System.out.println( "No command line "
+ "arguments found." );
}
}
|
Python
import sys
def factorial(n):
res = 1
for i in range ( 2 , n + 1 ):
res * = i
return res
if __name__ = = "__main__" :
if len (sys.argv) = = 1 :
print ( "No command line arguments found." )
else :
num = int (sys.argv[ 1 ])
print (factorial(num))
|
Output:
- In C:
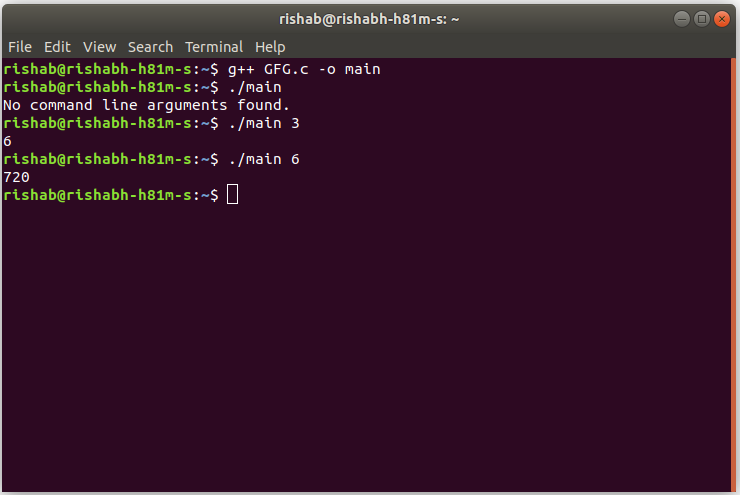
- In Java:
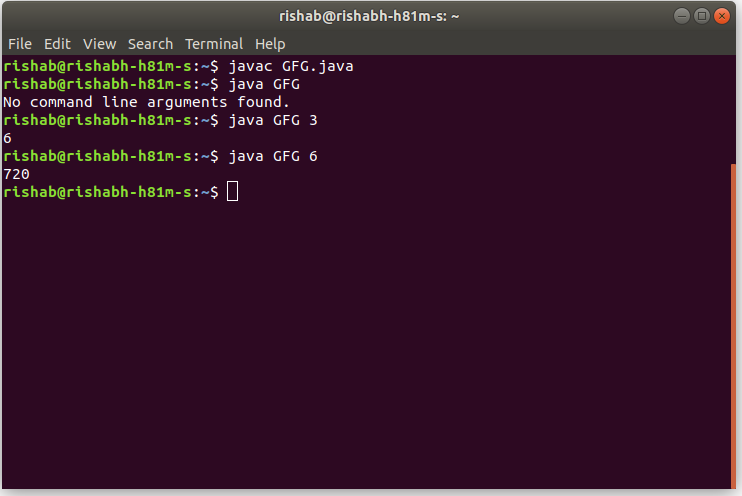
- In Python
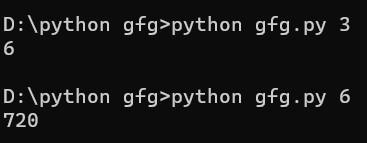
Time Complexity: O(N)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...