UDP Server-Client implementation in C++
Last Updated :
23 Feb, 2023
There are two primary transport layer protocols to communicate between hosts: TCP and UDP. Creating TCP Server/Client was discussed in a previous post.
Prerequisite: Creating TCP Server/Client
Theory: In UDP, the client does not form a connection with the server like in TCP and instead sends a datagram. Similarly, the server need not accept a connection and just waits for datagrams to arrive. Datagrams upon arrival contain the address of the sender which the server uses to send data to the correct client.
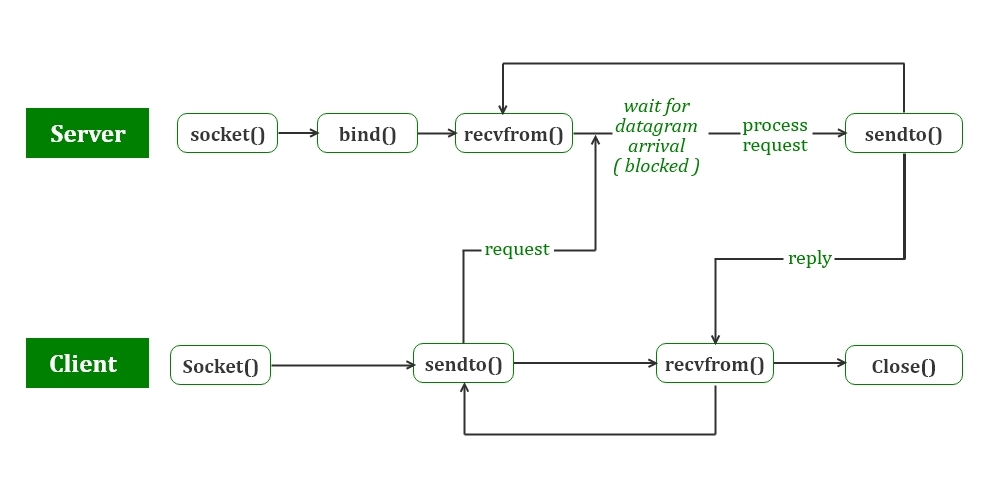
The entire process can be broken down into the following steps :
UDP Server :
- Create a UDP socket.
- Bind the socket to the server address.
- Wait until the datagram packet arrives from the client.
- Process the datagram packet and send a reply to the client.
- Go back to Step 3.
UDP Client :
- Create a UDP socket.
- Send a message to the server.
- Wait until a response from the server is received.
- Process the reply and go back to step 2, if necessary.
- Close socket descriptor and exit.
Necessary Functions :
int socket(int domain, int type, int protocol)
Creates an unbound socket in the specified domain.
Returns socket file descriptor.
Arguments :
domain – Specifies the communication
domain ( AF_INET for IPv4/ AF_INET6 for IPv6 )
type – Type of socket to be created
( SOCK_STREAM for TCP / SOCK_DGRAM for UDP )
protocol – Protocol to be used by the socket.
0 means using the default protocol for the address family.
int bind(int sockfd, const struct sockaddr *addr, socklen_t addrlen)
Assigns address to the unbound socket.
Arguments :
sockfd – File descriptor of a socket to be bonded
addr – Structure in which the address to be bound to is specified
addrlen – Size of addr structure
ssize_t sendto(int sockfd, const void *buf, size_t len, int flags,
const struct sockaddr *dest_addr, socklen_t addrlen)
Send a message on the socket
Arguments :
sockfd – File descriptor of the socket
buf – Application buffer containing the data to be sent
len – Size of buf application buffer
flags – Bitwise OR of flags to modify socket behavior
dest_addr – Structure containing the address of the destination
addrlen – Size of dest_addr structure
ssize_t recvfrom(int sockfd, void *buf, size_t len, int flags,
struct sockaddr *src_addr, socklen_t *addrlen)
Receive a message from the socket.
Arguments :
sockfd – File descriptor of the socket
buf – Application buffer is a pointer which receives the data
len – Size of buf application buffer
flags – Bitwise OR of flags to modify socket behavior
src_addr – Structure containing source address is returned
addrlen – Variable in which the size of src_addr structure is returned
int close(int fd)
Close a file descriptor
Arguments:
fd – File descriptor
In the below code, the exchange of one hello message between the server and client is shown to demonstrate the model.
Filename: UDPServer.c++
C++14
#include <bits/stdc++.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#define PORT 8080
#define MAXLINE 1024
int main() {
int sockfd;
char buffer[MAXLINE];
const char *hello = "Hello from server" ;
struct sockaddr_in servaddr, cliaddr;
if ( (sockfd = socket(AF_INET, SOCK_DGRAM, 0)) < 0 ) {
perror ( "socket creation failed" );
exit (EXIT_FAILURE);
}
memset (&servaddr, 0, sizeof (servaddr));
memset (&cliaddr, 0, sizeof (cliaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_addr.s_addr = INADDR_ANY;
servaddr.sin_port = htons(PORT);
if ( bind(sockfd, ( const struct sockaddr *)&servaddr,
sizeof (servaddr)) < 0 )
{
perror ( "bind failed" );
exit (EXIT_FAILURE);
}
socklen_t len;
int n;
len = sizeof (cliaddr);
n = recvfrom(sockfd, ( char *)buffer, MAXLINE,
MSG_WAITALL, ( struct sockaddr *) &cliaddr,
&len);
buffer[n] = '\0' ;
printf ( "Client : %s\n" , buffer);
sendto(sockfd, ( const char *)hello, strlen (hello),
MSG_CONFIRM, ( const struct sockaddr *) &cliaddr,
len);
std::cout<< "Hello message sent." <<std::endl;
return 0;
}
|
Filename: UDPClient.c++
C++14
#include <bits/stdc++.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#define PORT 8080
#define MAXLINE 1024
int main() {
int sockfd;
char buffer[MAXLINE];
const char *hello = "Hello from client" ;
struct sockaddr_in servaddr;
if ( (sockfd = socket(AF_INET, SOCK_DGRAM, 0)) < 0 ) {
perror ( "socket creation failed" );
exit (EXIT_FAILURE);
}
memset (&servaddr, 0, sizeof (servaddr));
servaddr.sin_family = AF_INET;
servaddr.sin_port = htons(PORT);
servaddr.sin_addr.s_addr = INADDR_ANY;
int n;
socklen_t len;
sendto(sockfd, ( const char *)hello, strlen (hello),
MSG_CONFIRM, ( const struct sockaddr *) &servaddr,
sizeof (servaddr));
std::cout<< "Hello message sent." <<std::endl;
n = recvfrom(sockfd, ( char *)buffer, MAXLINE,
MSG_WAITALL, ( struct sockaddr *) &servaddr,
&len);
buffer[n] = '\0' ;
std::cout<< "Server :" <<buffer<<std::endl;
close(sockfd);
return 0;
}
|
Output :
$ ./server
Client : Hello from client
Hello message sent.
$ ./client
Hello message sent.
Server : Hello from server
Share your thoughts in the comments
Please Login to comment...