Stack for Competitive Programming
Last Updated :
09 Jan, 2024
For competitive programming to be successful, efficient data structures and algorithms are essential. The stack is one such tool. In this article, we will examine how stack data structures play an important role in solving problems efficiently during competitive programming challenges. We will explore the basics of stacks with its use cases and some important problems related to stack that will help you to sharpen your competitive programming skills.
A stack is a linear data structure in which the insertion of a new element and removal of an existing element takes place at the same end represented as the top of the stack. A Stack works on the LIFO process (Last In First Out), i.e., the element that was inserted last will be removed first.
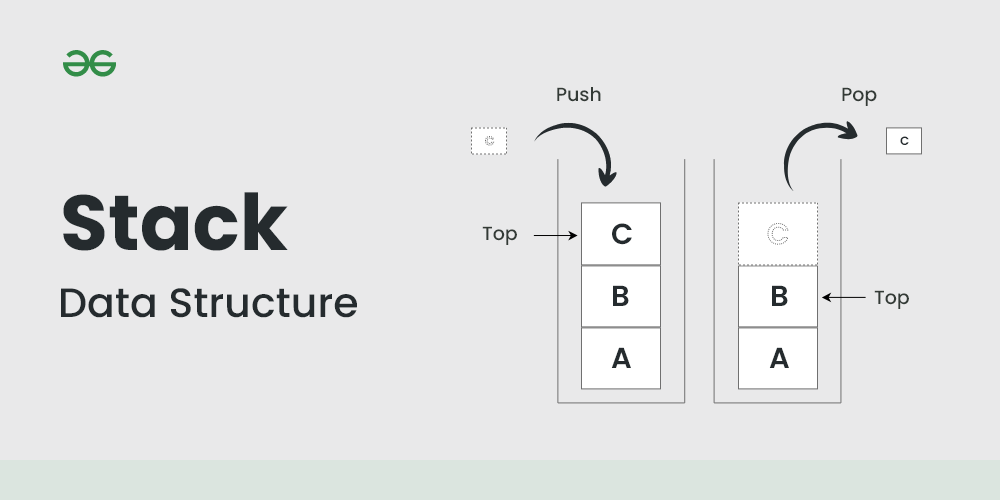
Stack Data Structure
Declaring a Stack
C++
#include<iostream>
#include <stack>
using namespace std;
int main(){
stack<dataType> myStack;
}
|
Java
import java.util.Stack;
public class Main {
public static void main(String[] args) {
Stack<DataType> myStack = new Stack<>();
}
}
|
Python3
C#
using System;
using System.Collections.Generic;
public class Main
{
public static void Main( string [] args)
{
Stack<DataType> myStack = new Stack<DataType>();
}
public class DataType
{
}
}
|
Javascript
There are various stack operations that are applicable on a stack. Stack operations are generally used to extract information and data from a stack data structure.
Some of the stack operations are given below:
1. Push element X into the stack
C++
Java
Python3
C#
Javascript
2. Pop the topmost element of the stack
C++
Java
Python3
C#
Javascript
3. Get the Topmost of the stack
C++
int topElement = myStack.top();
|
Java
int topElement = myStack.top();
|
Python3
top_element = my_stack[ - 1 ]
|
C#
int topElement = myStack.Top();
|
Javascript
let topElement = myStack[myStack.length - 1];
|
4. Size of Stack
C++
int stackSize = myStack.size();
|
Java
int stackSize = myStack.size();
|
Python3
stack_size = len (my_stack)
|
C#
int stackSize = myStack.Size();
|
Javascript
let stackSize = myStack.length;
|
5. Checks if the stack is empty
C++
Java
if (myStack.isEmpty()) {
}
|
Python3
C#
Javascript
if (myStack.length === 0) {
}
|
6. Swap two stacks
C++
stack< int > myStack;
stack< int > anotherStack;
myStack.swap(anotherStack);
|
Java
Deque<Integer> myStack = new ArrayDeque<>();
Deque<Integer> anotherStack = new ArrayDeque<>();
Deque<Integer> tempStack = myStack;
myStack = anotherStack;
anotherStack = tempStack;
|
Python3
my_stack = []
another_stack = []
my_stack, another_stack = another_stack, my_stack
|
C#
Queue< int > myStack = new Queue< int >();
Queue< int > anotherStack = new Queue< int >();
Queue< int > tempStack = myStack;
myStack = anotherStack;
anotherStack = tempStack;
|
Javascript
let myStack = [];
let anotherStack = [];
[myStack, anotherStack] = [anotherStack, myStack];
|
Must do Stack problems to understand the working of Stack
Use Cases of Stack in Competitive Programming
Here are some use cases of the stack data structure in competitive programming:
Many coding problems requires the pre-calculation of next smaller/greater element for each index of the array on left as well as right side. This can be calculated using stack in O(N) complexity
Example: Arr = [4,2,1,5,2,3,1]
Next greater on Left: [-1,4,2,-1,5,5,3] //-1 indicates that no greater element exists on left
Next greater on right:[5,5,5,-1,3,-1,-1] //-1 indicates that no greater element exists on right
Next smaller on left: [-1,-1,-1,1,1,2,-1] //-1 indicates that no smaller element exists on left
Next smaller on right:[2,1,-1,2,1,1,-1] //-1 indicates that no smaller element exists on right
Bracket problems in programming typically refer to problems that involve working with parentheses, and/or braces in expressions or sequences. It typically refers to problems related to the correct and balanced usage of parentheses, and braces in expressions or code.
These problems often involve checking if a given sequence of these symbols is well-formed, meaning that each opening symbol has a corresponding closing symbol in the correct order, and there are no unmatched or incorrectly nested symbols.
The most basic problem that falls under this category is balanced parenthesis, which state that Given a string containing various types of parentheses, such as ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘, ‘]’, you need to determine if the parentheses are balanced.
This problem is solved using a stack data structure. A stack can help you keep track of the opening parentheses you’ve seen so far. When you encounter a closing parenthesis, you can easily check if the top element of the stack matches it. If it does, you pop the opening parenthesis from the stack, indicating that it has been properly closed.
A monotonic stack is a stack whose elements are monotonically increasing or decreasing. It contains all qualities that a typical stack has and its elements are all monotonic decreasing or increasing.
Below are the features of a monotonic stack:
- It is a range of queries in an array situation
- The minima/maxima elements
- When an element is popped from the monotonic stack, it will never be utilised again.
The monotonic stack problem is mainly the previous/next smaller/larger problem. It maintains monotonicity while popping elements when a new item is pushed into the stack.
Suppose we have a problem in which we need to calculate the maximum for each and every contiguous subarray of size K for a given any given array. This problem can be solved in O(n) using a Deque data structure which has functionality of a stack as well as a queue. click here to see how.
To find cycle in a directed graph we can use the Depth First Traversal (DFS) technique. It is based on the idea that there is a cycle in a graph only if there is a back edge [i.e., a node points to one of its ancestors] present in the graph.
To detect a back edge, we need to keep track of the nodes visited till now and the nodes that are in the current recursion stack [i.e., the current path that we are visiting]. If during recursion, we reach a node that is already in the recursion stack, there is a cycle present in the graph.
Practice problems on Stack for Competitive Programming
Share your thoughts in the comments
Please Login to comment...